Beginner Level
Intermediate Level
Advanced Level
Introduction
In object-oriented programming, destructors are special methods that are called when an object is destroyed or goes out of scope. They are used to de-allocate the resources that were allocated during the object's lifetime, such as memory or file handles. Python's garbage collector takes care of most of the memory management for you, but sometimes it's necessary to clean up resources that are not managed by the garbage collector. In this tutorial, we'll explore the concept of destructors in Python and learn how to implement them in your code. So let's dive in and get started!
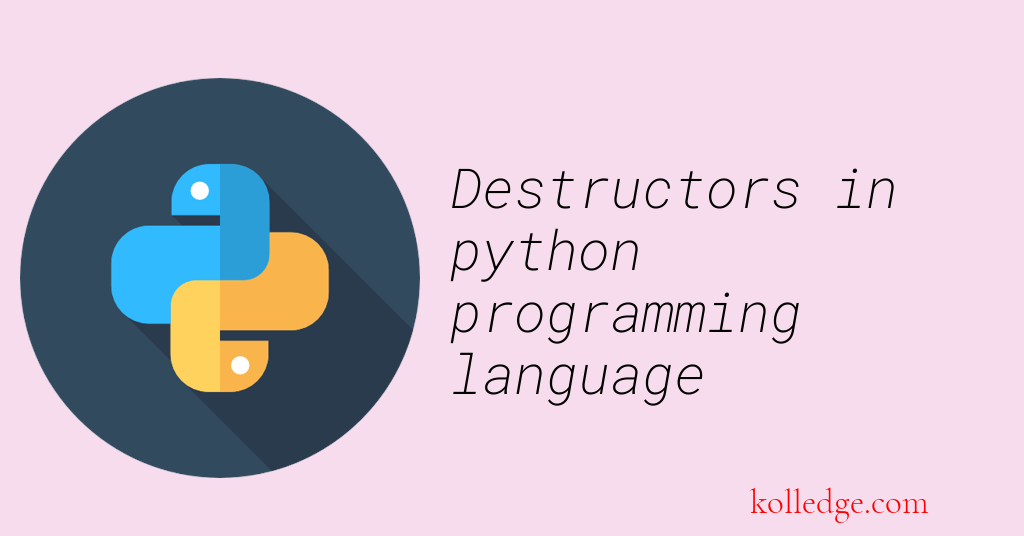
Table of Contents :
- What is a destructor in Python
- Some Points about Destructors
What is a destructor in Python
- A destructor is a special method that is called when an object is destroyed.
- Destructors are usually used to free memory or to close files.
- In Python, destructors are named as
__del__
. __del__
is called when an object is about to be destroyed.- It is called just before the memory is freed or the reference count reaches zero.
- Sample Code : Here is a simple example to demonstrate destructors in python.
class MyClass:
def __del__(self):
print ("MyClass is being destroyed")
Some Points about Destructors :
- When an object is destroyed, all its references are removed.
- If there are no other references to the object, the
__del__
method is called. - If the object has multiple references,
__del__
is called when the last reference is removed. - If an object is created inside another object and the parent object is destroyed, the child object is also destroyed.
- However, the child object's
__del__
method is not called. - Sample Code : Here is a sample code that creates an object and then removes all references to it :
class MyClass:
def __del__(self):
print ("MyClass is being destroyed")
obj = MyClass()
del obj
# Output
# MyClass is being destroyed
Prev. Tutorial : Constructors
Next Tutorial : Instance variables