Beginner Level
Intermediate Level
Advanced Level
Introduction
In Python, the issubclass() function is a built-in method that helps us to determine whether a given class is a subclass of another class. In this tutorial, we will explore the ins and outs of the issubclass() function, including its basic syntax, proper usage, and some helpful examples to illustrate its usefulness. By the end of this tutorial, you should have a strong understanding of how to use the issubclass() function in your Python projects to create efficient and effective object-oriented code.
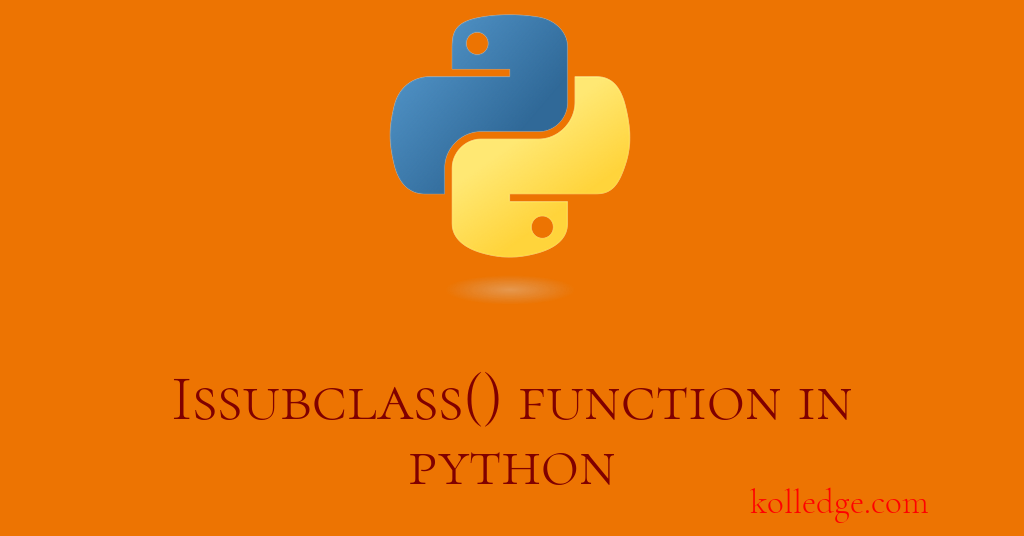
Table of Contents :
- issubclass() function
- Syntax
- issubclass() with Multiple Inheritance
- Object as Second Argument
issubclass() function :
- The `issubclass()` function in Python is used to check if a class is a subclass of another class.
- The function returns a boolean value of `True` if the given class is a subclass of the specified class.
Syntax:
- The syntax to use the `issubclass()` function is as follows:
- issubclass(subclass, superclass)
- The `subclass` argument is the class that we want to check if it is a subclass of the `superclass` argument.
Example:
class A:
pass
class B(A):
pass
class C(B):
pass
print(issubclass(C, A))
# Output: True
# In the above example, `C` is a subclass of `A`, so `issubclass(C, A)` returns `True`.
issubclass() with Multiple Inheritance :
- The `issubclass()` function also works with multiple inheritance.
- If the first argument is a tuple, the `issubclass()` function will check if the first argument is a subclass of any of the classes in the tuple.
Example:
class A:
pass
class B:
pass
class C(A, B):
pass
print(issubclass(C, (A, B)))
# Output: True
# In the above example, `C` is a subclass of both `A` and `B`, so the `issubclass()` function returns `True`.
Object as Second Argument :
- If the second argument of the `issubclass()` function is `object`, the function will return `True` if the first argument is a new-style class (inheriting from `object`), and `False` otherwise.
Example:
class A:
pass
class B:
pass
class C(A, B):
pass
print(issubclass(A, object)) # Output: True
print(issubclass(C, object)) # Output: True
# In the above example, both `A` and `C` inherit from `object`, so the `issubclass()` function returns `True` for both.
Prev. Tutorial : Method Resolution Order
Next Tutorial : What is exception handling