Introduction
One important feature of python is the ability to define class methods, which are methods that are bound to a class rather than an instance of that class. Class methods can be a powerful tool for developers looking to simplify their code and streamline their development process. In this Python tutorial, we will explore the basics of class methods, including how they work, how to define them, and how they can be used to improve the efficiency and functionality of your Python code. Whether you are a novice Python developer or a seasoned pro, this tutorial will provide you with a thorough understanding of this important programming concept.
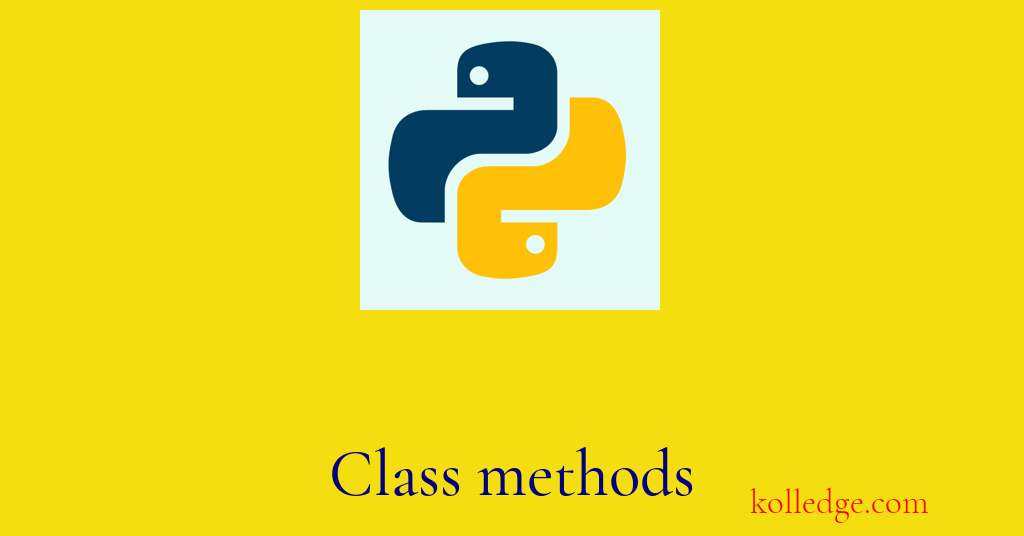
Table of Contents :
- Class methods
- Defining a class method
- Defining class methods by using the @classmethod decorator
- Defining class methods by using the classmethod() function
- Accessing a class method
- Add new class method dynamically
- Deleting class methods dynamically
- Class methods in case of inheritance
Class methods
- In the previous tutorial we have read that any method defined inside a class is an instance method by default.
- We can explicitly indicate that a method inside a class is a class method.
- Class methods are methods that are attached to the class itself.
- Class methods are used to access and process class variables.
- Class methods have access to only class attributes and not instance attributes.
- The first parameter of a class method is the
cls
keyword. - cls keyword refers to the class.
- We can use any other name instead of cls for the first parameter, but it is advised that we follow this convention.
- In case of inheritance, the class methods of parent class are available to the child class.
Defining a class method :
- The syntax of defining a class method is same as defining regular functions except that the first parameter in case of class methods is always
cls
. - The basic syntax of defining class methods is :
def method_name(cls, arg):
# method body
- By default, any method created inside a class is an instance method.
- If we want to define a class method, we need to explicitly indicate this by using :
- @classmethod decorator
- classmethod() function
Defining class methods by using the @classmethod decorator :
- We can tell python interpreter that the function inside the class is a class method by adding a
@classmethod
decorator before the function definition. - The first parameter of the class method should be
cls
. - Code Sample :
class Employee:
company_name = "ABC Inc."
def __init__(self, emp_name, emp_id, emp_desig):
# data members (instance variables - name, id and desig)
self.name = emp_name
self.id = emp_id
self.desig = emp_desig
@classmethod
def show_company(cls):
print(f"The name of the company is : {Employee.company_name}")
def print_details(self):
print(f"Name of employee = {self.name}")
print(f"ID of employee = {self.id}")
print(f"Designation of employee = {self.desig}")
print()
emp_1 = Employee("Steve", "k2591", "Manager")
emp_1.print_details()
Employee.show_company()
# Output
# Name of employee = Steve
# ID of employee = k2591
# Designation of employee = Manager
# The name of the company is : ABC Inc.
Defining class methods by using the classmethod() function :
- This is the older method of creating class methods.
- We can convert a normal function into a class method by using the built-in classmethod() function.
- The syntax of using classmethod() function is :
classmethod(function_name)
- Note that the method that we want to convert to class method should accept cls as the first parameter.
- Code Sample :
class Employee:
company_name = "ABC Inc."
def __init__(self, emp_name, emp_id, emp_desig):
# data members (instance variables - name, id and desig)
self.name = emp_name
self.id = emp_id
self.desig = emp_desig
def show_company(cls):
print(f"The name of the company is : {Employee.company_name}")
def print_details(self):
print(f"Name of employee = {self.name}")
print(f"ID of employee = {self.id}")
print(f"Designation of employee = {self.desig}")
print()
emp_1 = Employee("Steve", "k2591", "Manager")
emp_1.print_details()
# Creating a class method
Employee.show_company = classmethod(Employee.show_company)
# Calling the class method
Employee.show_company()
# Output
# Name of employee = Steve
# ID of employee = k2591
# Designation of employee = Manager
# The name of the company is : ABC Inc.
Accessing a class method :
- class methods can be accessed :
- using the class name and dot operator.
- using the object name and dot operator.
- The syntax of accessing a class method using class name is :
class_name.class_method_name()
- e.g.
Employee.show_company()
- The syntax of accessing a class method using object name is :
object_name.class_method_name()
- e.g.
emp_1.show_company()
Add new class method dynamically
- In Python we can add new class methods to a class at runtime.
- We might want to add a new class method at runtime in some scenarios like :
- We do not have access to the code of the class due to some reason.
- We do not want to change the structure of the original class as it is being used by others as well.
- Follow the steps below to add a class method dynamically :
- define the function that we want to add to the class dynamically.
- The first argument of this function should be
cls
. - Use the
classmethod()
function to add the function defined above to the class. - The function name is passed as argument to the
classmethod()
function.
- Code Sample :
class Employee:
company_name = "ABC Inc."
def __init__(self, emp_name, emp_id, emp_desig):
# data members (instance variables - name, id and desig)
self.name = emp_name
self.id = emp_id
self.desig = emp_desig
def print_details(self):
print(f"Name of employee = {self.name}")
print(f"ID of employee = {self.id}")
print(f"Designation of employee = {self.desig}")
print()
# Function that will be added to class
def show_company(cls):
print(f"The name of the company is : {Employee.company_name}")
# Adding the class method dynamically
Employee.show_company = classmethod(show_company)
emp_1 = Employee("Steve", "k2591", "Manager")
Employee.show_company() # Calling the class method added dynamically
emp_1.print_details()
# Output
# The name of the company is : ABC Inc.
# Name of employee = Steve
# ID of employee = k2591
# Designation of employee = Manager
Deleting class methods dynamically
- We can delete a class method of a class using :
- del keyword
- delattr() function
Using del keyword
- We can delete a class method by using
del
keyword. - The syntax for deleting class method is :
del class_name.class_method
- Code Sample :
class Employee:
company_name = "ABC Inc."
def __init__(self, emp_name, emp_id, emp_desig):
# data members (instance variables - name, id and desig)
self.name = emp_name
self.id = emp_id
self.desig = emp_desig
@classmethod
def show_company(cls):
print(f"The name of the company is : {Employee.company_name}")
# Deleting the class method
del Employee.show_company
# Calling the class method added
Employee.show_company()
# Output
# AttributeError: type object 'Employee' has no attribute 'show_company'
Using delattr() function
- We can delete a class method by using
delattr()
function. delattr()
function takes two arguments :- the class whose method needs to be deleted
- the name of the class method that we want to delete
- Code Sample :
class Employee:
company_name = "ABC Inc."
def __init__(self, emp_name, emp_id, emp_desig):
# data members (instance variables - name, id and desig)
self.name = emp_name
self.id = emp_id
self.desig = emp_desig
@classmethod
def show_company(cls):
print(f"The name of the company is : {Employee.company_name}")
# Deleting the class method
delattr(Employee, "show_company")
# Calling the class method added
Employee.show_company()
# Output
# AttributeError: type object 'Employee' has no attribute 'show_company'
Class methods in case of inheritance
- When we inherit a class from another class in Python, all the class methods of the parent class become available to the child class as well.
- We can call the class method using the name of the parent class or the child class.
- Code Sample :
class Employee:
company_name = "ABC Inc."
def __init__(self, emp_name, emp_id, emp_desig):
self.name = emp_name
self.id = emp_id
self.desig = emp_desig
@classmethod
def show_company(cls):
print(f"The name of the company is : {Employee.company_name}")
class ContractEmployees(Employee):
contract_term = "6 months"
def print_details(self):
print(f"Name of employee = {self.name}")
print(f"ID of employee = {self.id}")
print(f"Designation of employee = {self.desig}")
print(f"Contract Term = {self.contract_term}")
print()
emp_1 = ContractEmployees("Steve", "k2591", "Manager")
ContractEmployees.show_company()
emp_1.print_details()
# Output
# The name of the company is : ABC Inc.
# Name of employee = Steve
# ID of employee = k2591
# Designation of employee = Manager
# Contract Term = 6 months
Prev. Tutorial : Instance methods
Next Tutorial : Static methods