Introduction
The seek() function is an important built-in method in Python that allows us to move the file cursor to a particular position within a file. This function is commonly used when working with large files or binary data where you need to read or write data at specific locations within the file. The seek() function also supports different modes of file access like read, write, and append. In this tutorial, we will cover the syntax of the seek() function, its parameters, and different use cases with examples. By the end of this tutorial, you will have a better understanding of the seek() function and would be able to use it effectively in your programs.
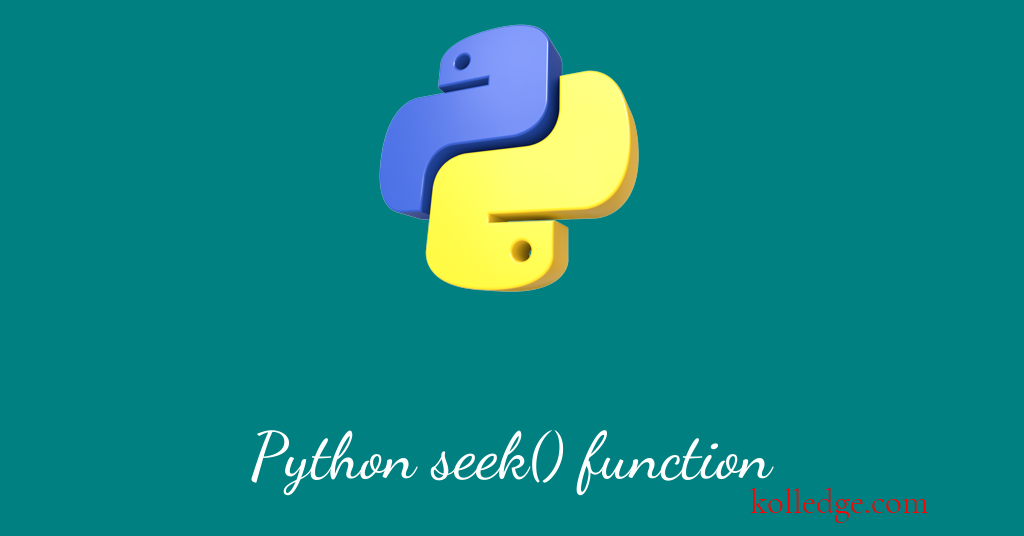
Table of Contents :
- What is seek() in Python
- How to use seek() method
- whence argument
- Different seek operations
- Seek the beginning of the file
- Seeking the end of file
- Seek from the current position
- Seek backward with negative offset
- tell() function to get file handle position
What is seek() in python
- The
seek()
function is used to change the file handle's position in a file. - The
seek()
function takes two parameters :- The first parameter is the Offset. It is the number of positions to move forward.
- The second parameter is whence. It is an optional parameter and It is used to set the starting position before moving forward.
How to use seek() method
- Before using the
seek()
method, open the file in read mode using theopen()
method. - Then, call the
seek()
method, with the desired position as the argument. - Code Sample :
# Example code for using seek() function
file = open("example.txt", "r")
# move file handle to position 10
file.seek(10)
# read from position 10 to the end of file
print(file.read())
file.close()
whence argument
- whence argument is used to specify the starting position for the
seek()
function. - The whence argument can have three values :
- 0 : The reference point is set to the beginning of the file.
- 1 : The reference point is set to the current file position.
- 2 : The reference point is set to the end of the file.
- Code Sample :
# Example code for using seek() function
file = open("example.txt", "r")
# move file handle to position 10
file.seek(10)
# read from position 10 to the end of file
print(file.read())
file.close()
Different seek operations
Seek the beginning of the file
- To move to the beginning of the file, use
seek(0)
orseek(0, 0)
. - Code Sample :
# Example code for using seek() function
file = open("example.txt", "r")
# move file handle to position 10
file.seek(10)
# read from position 10 to the end of file
print(file.read())
file.close()
Seeking the end of file
- To move to the end of the file, use
seek(0, 2)
. - Code Sample :
# Example code for using seek() function
file = open("example.txt", "r")
# move file handle to position 10
file.seek(10)
# read from position 10 to the end of file
print(file.read())
file.close()
Seek from the current position
- To move from the current position, use `seek(offset, 1)`, where `offset` is the number of positions to move forward or backward.
- A positive `offset` value moves the position forward, while a negative `offset` value moves the position backward.
- Code Sample :
# Example code for using seek() function
file = open("example.txt", "r")
# move file handle to position 10
file.seek(10)
# read from position 10 to the end of file
print(file.read())
file.close()
Seek backward with negative offset
- To move backward with negative offset, use `seek(offset, 2)`, where `offset` is the number of positions to move backward.
- Code Sample :
# Example code for using seek() function
file = open("example.txt", "r")
# move file handle to position 10
file.seek(10)
# read from position 10 to the end of file
print(file.read())
file.close()
tell() function to get file handle position
- The
tell()
function returns the current position of the file handle in the file. - Code Sample :
# Example code for using seek() and tell() functions
file = open("example.txt", "r")
file.seek(10) # move file handle to position 10
print(file.read()) # read from position 10 to the end of file
print(file.tell()) # print the current file handle position
file.close()
Prev. Tutorial : Check if a file exists
Next Tutorial : Rename Files