Introduction
Python offers powerful tools for working with date and time values. However, one common problem in working with these values is calculating the difference between two dates or times. Python's timedelta module provides an elegant solution to this problem. With the help of timedelta, you can easily perform arithmetic operations on date and time objects. By adding or subtracting a timedelta object to a date or time object, you can get a new date or time object that represents an offset from the original date or time. In this tutorial, we'll dive deeper into the timedelta module in Python and explore some common use cases.
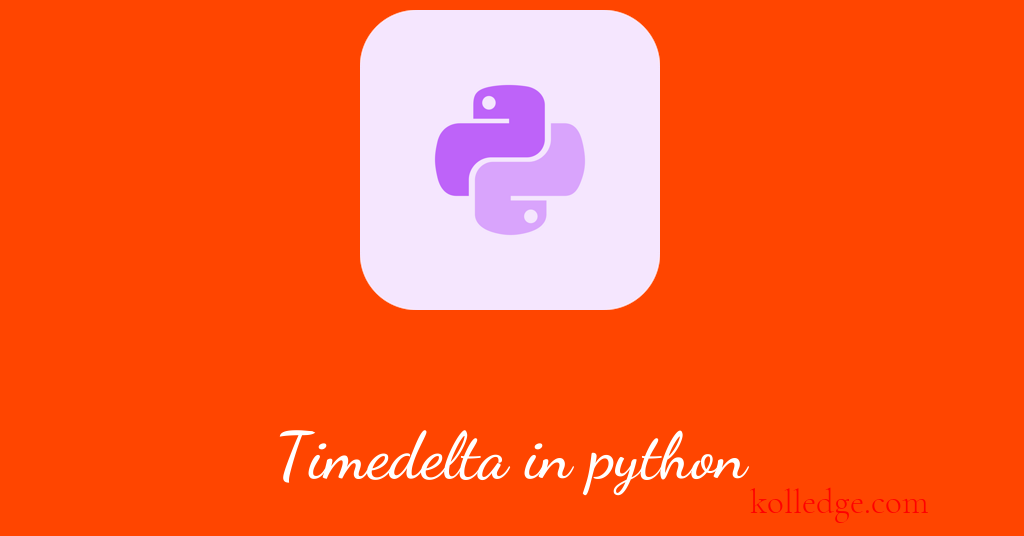
Table of Contents :
- Timedelta in Python
- Creating a timedelta Object
- With weeks constructor
- With eays constructor
- With hours constructor
- With seconds constructor
- With microseconds constructor
- Timedelta Arithmetic
- Find the difference between two dates.
- Add a time duration to a datetime object.
- Subtract a time duration from a datetime object.
- Add a time duration to a date object.
- Subtract a time duration from a date object.
- Add two timedelta objects
- Subtract two timedelta objects
- Compare two timedelta objects
- Timedelta Attributes
- Converting timedelta to Seconds
- Formatting a timedelta
- How to convert String to timedelta
Timedelta in Python
timedelta
is a class in Python's datetime module used to represent time duration.- It can represent duration in days, hours, minutes, seconds, and microseconds.
- We can import the
timedelta
class by using the construct :from datetime import timedelta
Creating a timedelta object
- The
timedelta()
function can be used to create atimedelta
object. - There are different ways of creating a timedelta object :
- Creating a timedelta object with weeks constructor
- Creating a timedelta object with days constructor
- Creating a timedelta object with hours constructor
- Creating a timedelta object with seconds constructor
- Creating a timedelta object with microseconds constructor
- Code Sample :
from datetime import timedelta
delta = timedelta(days=10, hours=5, minutes=30, seconds=20)
print("Timedelta:", delta)
# Output
# Timedelta: 10 days, 5:30:20
Creating a timedelta Object with weeks constructor
- We can create a
timedelta
object with weeks constructor using itsweeks
argument. - Code Sample :
from datetime import timedelta
delta = timedelta(weeks=3)
print("Timedelta:", delta)
# Output
# Timedelta: 21 days, 0:00:00
Creating a timedelta object with days constructor
- We can create a
timedelta
object with days constructor using itsdays
argument. - Code Sample :
from datetime import timedelta
delta = timedelta(days=20)
print("Timedelta:", delta)
# Output
# Timedelta: 20 days, 0:00:00
Creating timedelta object with hours constructor:
- We can create a
timedelta
object with hours constructor using itshours
argument. - Code Sample :
from datetime import timedelta
delta = timedelta(hours=122)
print("Timedelta:", delta)
# Output
# Timedelta: 5 days, 2:00:00
Creating a timedelta object with seconds constructor:
- We can create a
timedelta
object with seconds constructor using itsseconds
argument. - Code Sample :
from datetime import timedelta
delta = timedelta(seconds=3600)
print("Timedelta:", delta)
# Output
# Timedelta: 1:00:00
Creating a timedelta object with microseconds constructor:
- We can create a
timedelta
object with microseconds constructor using itsmicroseconds
argument. - Code Sample :
from datetime import timedelta
delta = timedelta(microseconds=500000)
print("Timedelta:", delta)
# Output
# Timedelta: 0:00:00.500000
Timedelta Arithmetic
- The
timedelta
class can be used to perform arithmetic on dates and times. - There are many different ways in which we can use the
timedelta
class to perform arithmetic on time duration. - Here we'll discuss the following ways of manipulating date and time with timedelta :
- Find the difference between two dates.
- Add a time duration to a
datetime
object. - Subtract a time duration from a
datetime
object. - Add a time duration to a
date
object. - Subtract a time duration from a
date
object. - Add two
timedelta
objects - Subtract two
timedelta
objects - Compare two
timedelta
objects
Find the difference between two dates
- To find the difference between two dates we can simply use the subtraction operator.
- The operation will return a
timedelta
object. - Code Sample :
from datetime import date
d1 = date(2017, 5, 19)
d2 = date(2017, 5, 15)
# Getting the difference between two dates
diff = d1 - d2
print("Time Diff :", diff)
print("Data type of diff : ", type(diff))
print()
# Output
# Time Diff : 4 days, 0:00:00
# Data type of diff : <class 'datetime.timedelta'>
Add a time duration to a datetime object.
- To add a time duration to a
datetime
object we can usetimedelta
object. - The resultant is a new
datetime
object. - Code sample :
from datetime import datetime
from datetime import timedelta
# Adding a time duration to a datetime object
dt = datetime(2017, 5, 9)
new_date = dt + timedelta(days=7)
print("Orig. date :", dt)
print("New date :", new_date)
print("Data type of New date :", type(new_date))
print()
# Output
# Orig. date : 2017-05-09 00:00:00
# New date : 2017-05-16 00:00:00
# Data type of New date : <class 'datetime.datetime'>
Subtract a time duration from a datetime object.
- We can subtract a time duration from a
datetime
object usingtimedelta
object. - The resultant is a new
datetime
object. - Code sample :
from datetime import datetime
from datetime import timedelta
# Subtracting a time duration to a datetime object
dt = datetime(2017, 5, 9)
new_date = dt - timedelta(days=7)
print("Orig. date :", dt)
print("New date :", new_date)
print("Data type of New date :", type(new_date))
print()
# Output
# Orig. date : 2017-05-09 00:00:00
# New date : 2017-05-02 00:00:00
# Data type of New date : <class 'datetime.datetime'>
Add a time duration to a date object.
- We can add a time duration to a
date
object usingtimedelta
object. - The resultant is a new
date
object. - Code sample :
from datetime import date
from datetime import timedelta
# Adding a time duration to a date object
d = date(2017, 5, 19)
new_date = d + timedelta(days=7)
print("Orig. date :", d)
print("New date :", new_date)
print("Data type of New date :", type(new_date))
print()
# Output
# Orig. date : 2017-05-19
# New date : 2017-05-26
# Data type of New date : <class 'datetime.date'>
Subtract a time duration from a date object.
- We can subtract a time duration from a
date
object usingtimedelta
object. - The resultant is a new
date
object. - Code sample :
from datetime import date
from datetime import timedelta
# Subtracting a time duration to a date object
d = date(2017, 5, 19)
new_date = d - timedelta(days=7)
print("Orig. date :", d)
print("New date :", new_date)
print("Data type of New date :", type(new_date))
print()
# Output
# Orig. date : 2017-05-19
# New date : 2017-05-12
# Data type of New date : <class 'datetime.date'>
Add two timedelta objects
- We can add two
timedelta
objects to get a newtimedelta
object. - Code sample :
from datetime import timedelta
delta1 = timedelta(days=10)
delta2 = timedelta(hours=12)
result = delta1 + delta2
print("Result:", result)
# Output:
# Result: 10 days, 12:00:00
Subtract two timedelta objects
- We can subtract two
timedelta
objects to get a newtimedelta
object. - Code sample :
from datetime import timedelta
delta1 = timedelta(days=10)
delta2 = timedelta(days=4, hours=10)
result = delta1 - delta2
print("Result:", result)
# Output:
# Result: 5 days, 14:00:00
Compare two timedelta objects
- We can compare two
timedelta
objects using the usual comparison operators. - Code sample :
from datetime import timedelta
delta1 = timedelta(days=10)
delta2 = timedelta(days=9, hours=10)
if delta1 > delta2:
print("delta1 is greater than delta2")
else:
print("delta1 is less than or equal to delta2")
# Output
# delta1 is greater than delta2
Timedelta Attributes:
- We can access the number of days, seconds, and microseconds in a
timedelta
object using its attributes - The name of the attributes are :
- days,
- seconds, and
- microseconds respectively.
- Code Sample :
from datetime import timedelta
delta = timedelta(days=10, hours=5, minutes=30, seconds=20, microseconds=30)
print("Days :", delta.days)
print("Seconds :", delta.seconds)
print("Microseconds :", delta.microseconds)
# Output:
# Days : 10
# Seconds : 19820
# Microseconds : 30
Converting timedelta object to seconds
- We can convert a
timedelta
object to the total number of seconds using itstotal_seconds()
method. - Code Sample :
from datetime import timedelta
delta = timedelta(hours=1, minutes=30, seconds=20)
seconds = delta.total_seconds()
print("Total seconds:", seconds)
# Output
# Total seconds: 5420.0
Formatting a timedelta object
- We can use the
str()
function and format codes to convert atimedelta
object to a string. - Code Sample :
from datetime import timedelta
delta = timedelta(days=10, hours=5, minutes=30, seconds=20)
delta_string = str(delta)
print("Timedelta string:", delta_string)
# Output
# Timedelta string: 10 days, 5:30:20
How to convert string to timedelta
- We can convert a string to timedelta using the strptime() function in python.
- The process of converting a string to timedelta is :
- First convert the string to
datetime
object using thestrptime()
function. - then we calculate the total number of seconds in the obtained datetime object.
- then we can use the
timedelta()
function of thedatetime
module to get the timedelta value.
- First convert the string to
- Code Sample :
import datetime
str_td = "15:12:40"
dt = datetime.datetime.strptime(str_td, "%H:%M:%S")
# Calculating total number of seconds
total_sec = dt.hour*3600 + dt.minute*60 + dt.second
td = datetime.timedelta(seconds=total_sec)
print("Timedelta : ",td)
print("Data Type of td : ", type(td))
# Output
# Timedelta : 15:12:40
# Data Type of td : <class 'datetime.timedelta'>
Prev. Tutorial : timestamp to datetime
Next Tutorial : Using time module