Introduction
Learning how to work with data in Python is an essential skill for any data analyst or scientist. One common format for storing data is in CSV (Comma Separated Values) files. Python offers a variety of libraries and functions to read, write, manipulate, and analyze CSV files. In this tutorial, we will explore how to use Python to work with CSV files. From reading data into a dataframe, sorting and filtering data, to exporting data for further analysis, we will cover everything you need to know to start using Python for CSV files. Whether you are new to Python or looking to improve your data processing skills, this tutorial will provide you with a solid foundation.
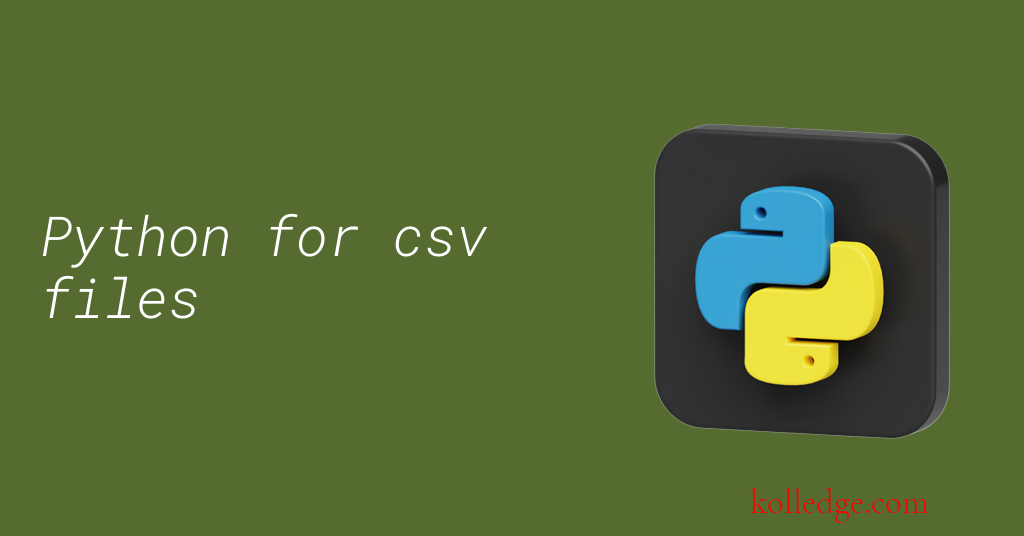
Table of Contents :
- What is a CSV File
- Uses of CSV Files
- Advantages of CSV Files
- Working with CSV Files in Python
- Few things to Remember
What is a CSV File :
- CSV stands for Comma Separated Values,
- A CSV file is a file format used for storing tabular data.
- Each row in the file represents a record.
- The columns represent the fields of the record.
- The values of each column are separated by commas.
Uses of CSV Files :
- CSV files are widely used in
- data analysis,
- importing and exporting data between different software programs,
- sharing data with others.
- CSV files can be easily created and edited using spreadsheet software such as Microsoft Excel or Google Sheets.
- They are also easy to read and manipulate using programming languages like Python, Java, and R.
Advantages of CSV Files :
- CSV files offer several advantages over other file formats such as Excel files or SQL databases.
- They are lightweight and easy to share via email or cloud storage platforms.
- They are also human readable and can be opened in any text editor.
- CSV files can be used for a variety of purposes such as :
- creating data backups,
- sharing data between different software applications, and
- automating data processing tasks.
Working with CSV Files in Python :
- Python has a built in module called csv that can be used to read and write csv files.
- We can read CSV files in Python by using the
csv.reader()
method of thecsv
module.
- We can read CSV files in Python by using the
- The next tutorial in this course deals with reading csv files in python in more detail.
- To write csv files in Python we can use the csv.writer() method of the csv module.
- The tutorial ahead in this course deals with writing csv files in python in more detail.
Few things to Remember :
There are a few things to keep in mind when working with CSV files.
- First, CSV files are typically encoded using UTF8.
- This means that any special characters in the file will be encoded using this character set.
- To work with a CSV file that uses a different character set, we'll have to specify the encoding.
- For example, to open a CSV file encoded using Latin1, you would use the following code:
import csv
with open('sample.csv', encoding='latin1') as csvfile: ...
- Second, CSV files use a comma to separate values.
- If a value contains a comma, it will need to be enclosed in quotes.
- For example, the following row would be considered invalid: value1,value2,value3,value4
- To make this row valid, we would need to enclose the third value in quotes: value1,value2,"value3,value4"
- Third, CSV files may or may not have a header row.
- A header row contains the names of each column in the file.
- If a CSV file has a header row, you can use the
csv.DictReader
class to read the file. - This class works like the csv.reader class, but each row is returned as a dictionary.
- The keys of the dictionary are the values in the header row.
- For example, given the following CSV file: id,name,age 1,John,20 2,Jane,30 We could use the following code to read the file:
import csv
with open('sample.csv') as csvfile:
reader = csv.DictReader(csvfile)
for row in reader:
print(row['name'])
# This would print the name of each row in the file.
Prev. Tutorial : PrettyPrint JSON Data
Next Tutorial : Reading csv files