Beginner Level
Intermediate Level
Advanced Level
Introduction
Regular expressions, or regex, are powerful tools for pattern matching and text manipulation. Alternation allows us to match multiple patterns and choose the one that occurs first. Whether you're a beginner or an experienced Python developer, understanding alternation in regex will expand your toolkit for string manipulation and enable you to solve complex text-related problems with ease. So let's dive in and explore the wonders of regex alternation in Python!
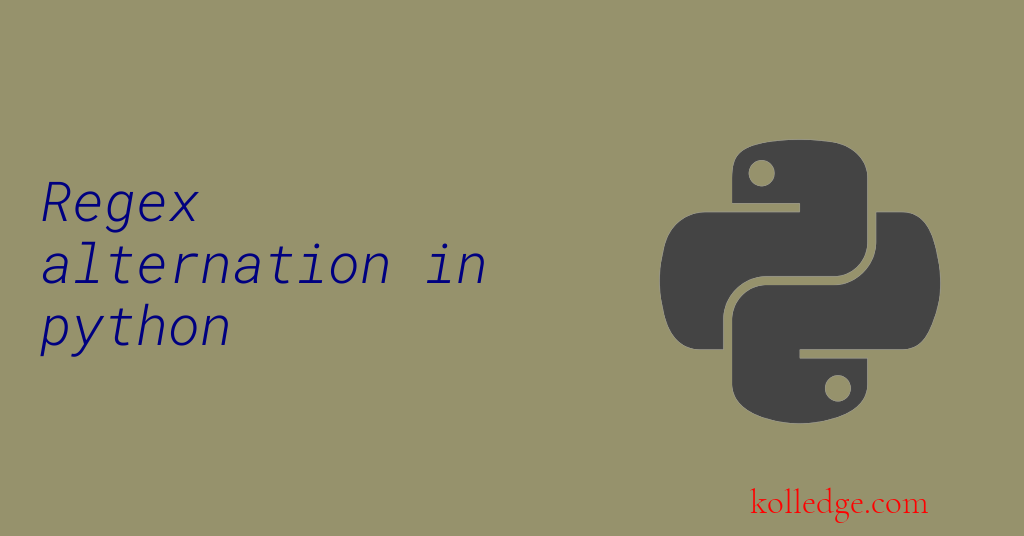
Table of Contents :
- Python Regex Alternation
- Match Time in hh:mm format using Regex Alternation
Python Regex Alternation :
- Alternation is a feature of Python's regular expression syntax that allows you to specify multiple patterns, any of which can match the target string.
- Alternation is denoted by the pipe character
|
, which separates the alternative patterns to be matched. - Code Sample :
import re
# Alternation example
text = "The quick brown fox jumps over the lazy dog."
pattern = r"quick|lazy"
result = re.findall(pattern, text)
print(result)
# Output:
['quick', 'lazy']
Match Time in hh:mm format using Regex Alternation :
- Alternation can also be used to match time in
hh:mm
format. - To match time in this format, you can use a regular expression pattern that specifies alternative formats for the hours and minutes.
- The hours can be matched using the pattern
0?\d|1[0-2]
, which matches either a single digit (optionally preceded by a leading zero), or the numbers10
through12
. - The minutes can be matched using the pattern
[0-5]\d
, which matches any two digits between00
and59
. - Code Sample :
import re
# Alternation example to match time in hh:mm format
text = "The meeting will start at 10:30 AM."
pattern = r"((0?\d|1[0-2]):([0-5]\d)\s([AP]M))"
result = re.findall(pattern, text)
print(result)
# Output:
[('10:30 AM', '10', '30', 'AM')]
Prev. Tutorial : Backreferences
Next Tutorial : Non-capturing groups