Introduction
In concurrent programming, where multiple tasks need to be completed simultaneously, multiprocessing plays a vital role in achieving high-performance computing. And one of the ways of implementing multiprocessing in Python is through the use of Process Pools. A Process Pool is a group of worker processes that are created to handle a pool of tasks. The use of Process Pools can significantly speed up the processing time of tasks that would otherwise take a considerable amount of time when executed sequentially. In this tutorial, we will learn about implementing Process Pools in Python and how to use them to handle intensive computational tasks more efficiently. So, let's dive in!
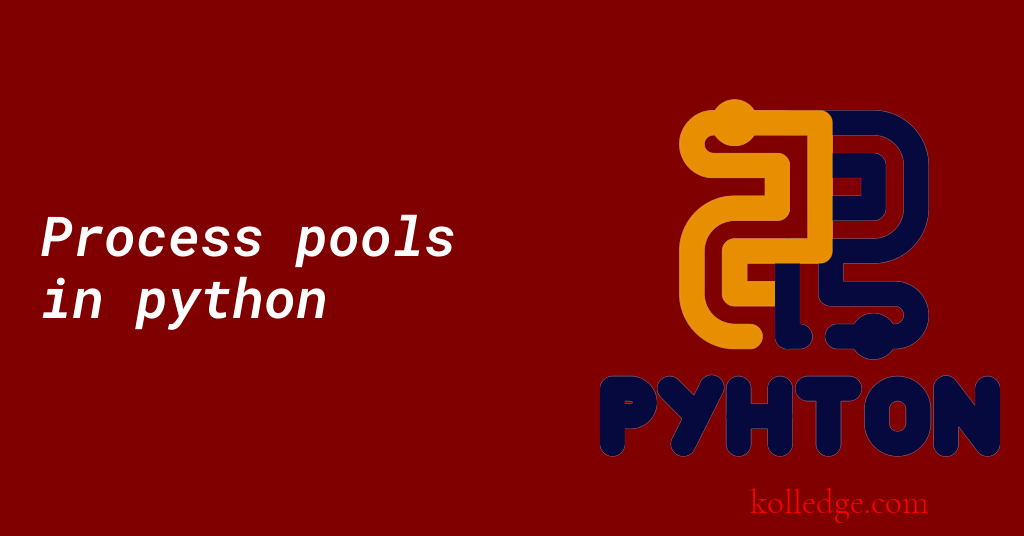
Table of Contents :
- ProcessPoolExecutor class in Python
- How to use the ProcessPoolExecutor Class
ProcessPoolExecutor class in Python :
- The
ProcessPoolExecutor
class is a part of theconcurrent.futures
module in Python. - It provides a simple way to create and manage a pool of worker processes in Python.
ProcessPoolExecutor
can be useful for executing CPU intensive tasks in parallel to speed up the execution time.- Here's an example of how to use the
ProcessPoolExecutor
class to execute CPU bound tasks in parallel. - Code Sample :
import concurrent.futures
def square(num):
return num * num
if __name__ == '__main__':
with concurrent.futures.ProcessPoolExecutor() as executor:
numbers = [1, 2, 3, 4, 5]
results = executor.map(square, numbers)
for result in results:
print(result)
How to use the ProcessPoolExecutor Class :
- To use the
ProcessPoolExecutor
class, we need to follow these steps:- Import the
concurrent.futures
module - Create an instance of the
ProcessPoolExecutor
class - Use the
submit
method to submit tasks to the pool of workers - Use the
map
method to execute the tasks and retrieve the results
- Import the
- Code Sample :
import concurrent.futures
def square(num):
return num * num
if __name__ == '__main__':
with concurrent.futures.ProcessPoolExecutor() as executor:
# submit tasks to the pool of workers
futures = [executor.submit(square, num) for num in range(1, 6)]
# execute the tasks and retrieve the results
for future in concurrent.futures.as_completed(futures):
print(future.result())
- In the above example, the submit method is used to submit tasks to the pool of workers.
- The as_completed method is used to execute the tasks and retrieve the results as they become available.
Prev. Tutorial : Multiprocessing in Python
Next Tutorial : Event loop