Introduction
In Python, a set is an unordered collection of unique elements that provides a powerful way to work with data. Sets allow you to perform various operations like union, intersection, and difference, making them ideal for tasks such as removing duplicates, testing membership, and performing set operations. With their efficient lookup and manipulation capabilities, sets are a valuable tool in Python programming. In this tutorial, we will delve into the world of sets, exploring their creation, manipulation, and common operations, equipping you with the skills to effectively work with sets in your Python projects. Let's dive in and discover the versatility of sets in Python!
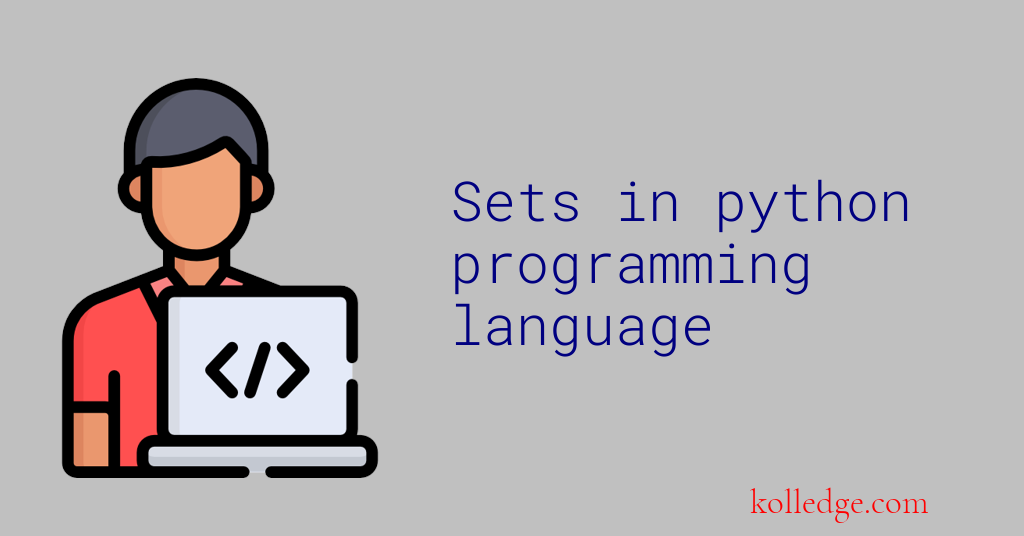
Table of Contents :
- Sets in Python
- Defining a set using curly braces
- Defining a set using set() function
- Different operations on Sets in Python
- getting the number of elements
- Adding a new element to the set
- Removing an element from the set
- Removing all elements of the set
- checking if an element is present in the set
- returning a set element
- Looping through all elements of set
- Frozen Sets
Sets in Python :
- Sets in Python are unordered collection of elements.
- The set data type is implemented through
set
class. - Sets cannot have duplicate elements.
- The elements of a Set in Python cannot be changed.
- Sets in Python are defined using curly braces
{}
. - Dictionaries also use curly braces but dictionary is made of key-value pairs.
- We can create a new set using :
- curly braces syntax
- built-in
set()
function
- The elements of the set should be of hashable type.
- Lists or dictionaries are not allowed as elements of sets.
- If we try to add a list as set element, python interpreter will raise an error :
un-hashable type: 'list'
Defining a set using curly braces :
- The basic syntax of defining a set in Python is :
new_set = {item1, item2,item3}
- We cannot define an empty set using curly braces as it will clash with defining an empty dictionary :
empty_set = {}
- Code Sample :
my_set = {1, 2, 3, 4}
print(f"Value of my_set = {my_set}")
print(f"Data Type my_set = {type(my_set)}")
# Output
# Value of my_set = {1, 2, 3, 4}
# Data Type my_set = <class 'set'>
Defining a set using set() function :
- To define an empty set we can use the built-in set() function of Python :
empty_set = set()
- A set can be created using
set()
function by passing an iterable object such as a list or a tuple as an argument. - If there are duplicate items in the iterable that we passed as argument to set function, they will be removed.
- The original order of the elements in the iterable object may or may not be preserved in the new set.
- Code Sample :
my_set = set([1, 2, 3, 4])
print(f"Value of my_set = {my_set}")
# Output
# Value of my_set = {1, 2, 3, 4}
# Passing a tuple
my_set = set((10, 20, 30, 40))
print(f"Value of my_set = {my_set}")
# Output
# Value of my_set = {40, 10, 20, 30}
# Passing duplicates
my_set = set([1, 2, 3, 4, 2, 3])
print(f"Value of my_set = {my_set}")
# Output
# Value of my_set = {1, 2, 3, 4}
Different operations on Sets in Python :
Different operations possible with sets in python are :
- Getting the number of elements
- Fetch individual elements of a set.
- Looping through all elements of set
- Adding a new element to the set
- Removing an element from the set
- Removing all elements of the set
- checking if an element is present in the set
- returning a set element
Getting the number of elements of a set :
- The built-in
len()
function is used to get the length of the set. - length of the set means the number of elements present in the set.
- The syntax of using the
len()
method is :set_len = len(set_name)
- Code Sample :
my_set = {10, 20, 30, 40}
print(f"Value of my_set = {my_set}")
print(f"Length of my_set = {len(my_set)}")
# Output
# Value of my_set = {40, 10, 20, 30}
# Length of my_set = 4
Fetching individual elements of a set :
- We cannot perform indexing in case of sets in python.
- If we try to access set elements using index python interpreter will give an error :
TypeError: 'set' object is not subscriptable
- To access individual elements using indexing we'll have to convert set into a list.
- To convert set into a list we can use the in-built
list()
method. - Code Sample :
my_set = {1, 2, 3, 4}
print(f"Value of my_set = {my_set}")
print(f"Element at index 0 = {list(my_set)[0]}")
print(f"Element at index 1 = {list(my_set)[1]}")
print(f"Element at index 2 = {list(my_set)[2]}")
# Output
# Value of my_set = {1, 2, 3, 4}
# Element at index 0 = 1
# Element at index 1 = 2
# Element at index 2 = 3
# Getting Last element of the set
print(f"Last Element of the set = {list(my_set)[-1]}")
# Output
# Last Element of the set = 4
- Note # : We can also use the built-in iter() method in conjunction with the next() method to access individual elements of a set.
- Code Sample :
my_set = {1, 2, 3, 4}
print(f"Value of my_set = {my_set}")
print(f"First element of the set = {next(iter(my_set))}")
# Output
# Value of my_set = {1, 2, 3, 4}
# First element of the set = 1
Adding a new element to the set :
- The built-in
add()
method is used to add a new element to a set. - The syntax of using the add method is :
orig_set.add(new_element)
- Code Sample :
my_set = {10, 20, 30, 40}
print(f"Value of my_set = {my_set}")
# Adding new element to set
my_set.add(50)
print(f"Value of my_set = {my_set}")
# Output
# Value of my_set = {40, 10, 20, 30}
# Value of my_set = {40, 10, 50, 20, 30}
Removing an element from the set :
- We can remove an element from a set using :
remove()
methoddiscard()
method
- The built-in
remove()
method is used to remove an element from a set. - The syntax of using the remove method is :
orig_set.remove(set_element)
- If we try to remove an element that is not present in the set, then interpreter raises an error.
- It is a good practice to use the in operator to check if the element is present in the set or not before trying to remove it.
- Code Sample :
my_set = {10, 20, 30, 40}
print(f"Value of my_set = {my_set}")
# Deleting an element to set
my_set.remove(30)
print(f"Value of my_set = {my_set}")
# Output
# Value of my_set = {40, 10, 20, 30}
# Value of my_set = {40, 10, 20}
# Deleting element that is not present
my_set.remove(100)
print(f"Value of my_set = {my_set}")
# Output
# KeyError: 100
- The built-in
discard()
method can also be used to remove elements from a set. - The
discard()
method does not raise an error even if we try to remove an element that is not present in the set. - The syntax of using the discard method is :
orig_set.discard(set_element)
- Code Sample :
my_set = {10, 20, 30, 40}
print(f"Value of my_set = {my_set}")
# Deleting an element to set
my_set.remove(30)
print(f"Value of my_set = {my_set}")
# Deleting element that is not present
my_set.remove(100)
print(f"Value of my_set = {my_set}")
# Output
# Value of my_set = {40, 10, 20, 30}
# Value of my_set = {40, 10, 20}
# Value of my_set = {40, 10, 20}
Removing all elements of the set :
- The built-in
clear()
method is used to remove all elements of a set. - The syntax of using the clear method is :
orig_set.clear()
- Code Sample :
my_set = {10, 20, 30, 40}
print(f"Value of my_set = {my_set}")
# Deleting all elements of set
my_set.clear()
print(f"Value of my_set = {my_set}")
# Output
# Value of my_set = {40, 10, 20, 30}
# Value of my_set = set()
Checking if an element is present in the set :
- We can use the in operator to check if an element is present in the set or not.
- in operator is usually used along with a conditional statement.
- The syntax of using the in operator is as follows -
if element in set_name:
# process logic
- The in operator returns a boolean value -
- True if the element is present in the set.
- False if the element is not present in the set.
- Code Sample :
my_set = {1, 2, 3, 4}
print(f"Value of my_set = {my_set}")
k = 3
if k in my_set:
print(f"Element {k} is present in my_set")
else:
print(f"Element {k} is not present in my_set")
# Output
# Value of my_set = {1, 2, 3, 4}
# Element 3 is present in my_set
Returning an element from a set :
- The built-in
pop()
method is used to return an element. - The
pop()
method removes the element from the set and then returns it. - The syntax of using the pop() method is :
element = orig_set.pop()
- The
pop()
function can return any element of the set as there is no fixed order of the elements is sets. - Code Sample :
my_set = {1, 2, 3, 4}
print(f"Value of my_set = {my_set}")
k = my_set.pop()
print(f"Element {k} was popped from my_set")
print(f"Value of my_set = {my_set}")
# Output
# Value of my_set = {1, 2, 3, 4}
# Element 1 was popped from my_set
# Value of my_set = {2, 3, 4}
Looping through all elements of set :
- Set in Python is an iterable object.
- A for loop can be used to iterate a set.
- If we need to get the index value of the element inside the loop body we can use the enumerate method.
- We can pass a second argument to the enumerate method to specify the starting index.
- The order in which the set elements are iterated is not defined.
- The order can be different every time we iterate over the set.
- Code Sample :
my_set = {1, 2, 3, 4}
print(f"Value of my_set = {my_set}")
print()
print("Iterating through set elements")
for i in my_set:
print(f"Value of element = {i}")
# Output
# Value of my_set = {1, 2, 3, 4}
# Iterating through set elements
# Value of element = 1
# Value of element = 2
# Value of element = 3
# Value of element = 4
print("Getting index of elements")
for index, item in enumerate(my_set):
print(f"Element at index {index} = {item}")
# Output
# Getting index of elements
# Element at index 0 = 1
# Element at index 1 = 2
# Element at index 2 = 3
# Element at index 3 = 4
Frozen Sets :
- Frozen sets are immutable sets.
- In other words, the elements of a frozen set cannot be changed once defined.
- The built-in
frozenset()
method is used to create an immutable set from an existing mutable set. - The syntax of using the frozenset method is :
immutable_set = frozeset(mutable_set)
- Code Sample :
my_set = {1, 2, 3, 4}
print(f"Value of my_set = {my_set}")
print(f"Data Type of my_set = {type(my_set)}")
print()
my_frozen_set = frozenset(my_set)
print(f"Value of my_frozen_set = {my_frozen_set}")
print(f"Data Type of my_frozen_set = {type(my_frozen_set)}")
# Output
# Value of my_set = {1, 2, 3, 4}
# Data Type of my_set = <class 'set'>
# Value of my_frozen_set = frozenset({1, 2, 3, 4})
# Data Type of my_frozen_set = <class 'frozenset'>
Prev. Tutorial : Dictionary comprehension
Next Tutorial : Set operations