Beginner Level
Intermediate Level
Advanced Level
Introduction
Strings are one of the essential data types in Python that are used to represent textual data. In this tutorial, we will dive into the concept of modifying or deleting strings in Python. You will learn various techniques used to manipulate strings and how to modify or delete parts of strings using slicing and built-in string methods. Whether you're a beginner or an experienced programmer, this tutorial will provide you with valuable insights on how to work with strings in Python.
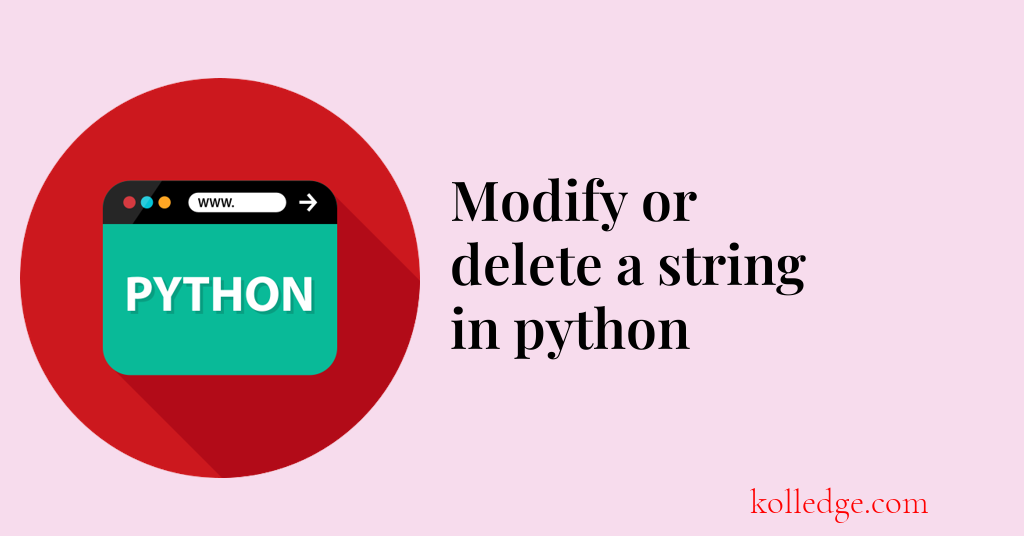
Table of Contents :
- Updating a character of a string
- Updating Entire String
- Deleting a character of a string
- Deleting Entire String
Updating a character of a string :
- Since strings are immutable in Python, we need to create a new string to update a character.
- We can use string slicing to extract the characters before and after the character we want to update,
- and then concatenate them with the updated character.
- Code Sample :
my_string = "Hello, world!"
updated_string = my_string[:7] + "W" + my_string[8:]
print(updated_string)
# Output
# Hello, World!
Updating Entire String :
- We can use the assignment operator
=
to assign a new value to the string variable. - Code Sample :
my_string = "Hello, world!"
my_string = "Hi, there!"
print(my_string)
# Output
# Hi, there!
Deleting a character of a string :
- Since strings are immutable in Python, we need to create a new string without the character we want to delete.
- The process of deleting a character from a string is as follows -
- extract sub-string before the character we want to delete,
- extract sub-string after the character we want to delete,
- and then concatenate these extracted sub-strings.
- Code Sample :
my_string = "Hello, World!"
updated_string = my_string[:5] + my_string[6:]
print(updated_string)
# Output
# Hello World!
Deleting Entire String :
- We can use the
del
keyword to delete the string variable. - Code Sample :
my_string = "Hello, world!"
del my_string
print(my_string)
# Output
# NameError: name 'my_string' is not defined
Prev. Tutorial : Concatenate strings
Next Tutorial : Escape sequencing a string