Introduction
One of the essential features of Python is its capability to format output when printing out variables or values. Output formatting allows for clear and concise presentation of data and results. In this Python tutorial, we will explore the string modulo operator, which is one of the standard ways of formatting output in Python. We will cover the basics of output formatting with string modulo, including the syntax, format codes, and common use cases for this powerful formatting tool. By the end of this tutorial, you will have a firm understanding of how output formatting works in Python and be able to apply it to your programs with confidence.
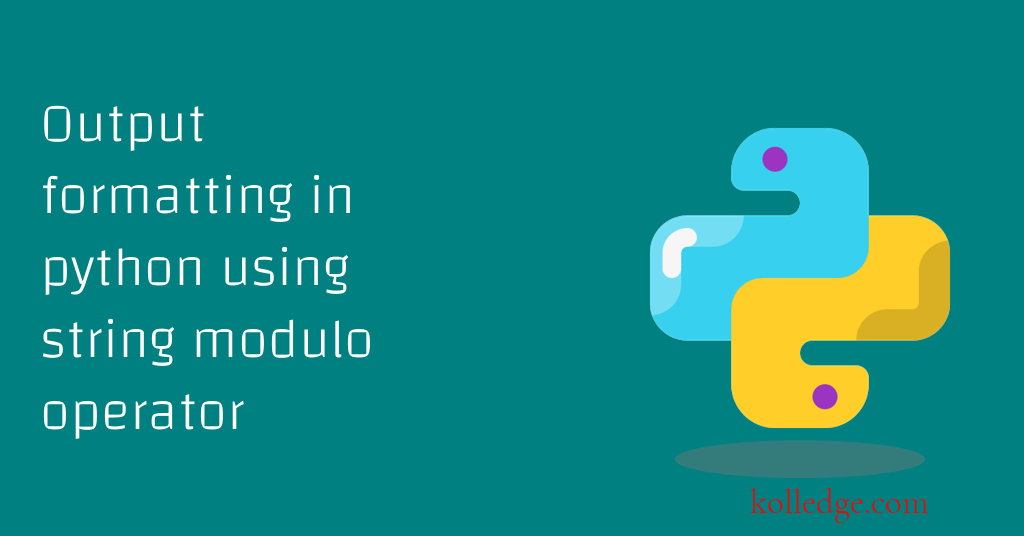
Table of Contents :
- Output Formatting using String modulo operator (%)
- Conversion Specifier
- Conversion types
Output Formatting using String modulo operator (%)
- This is the older way of formatting strings though it still works with Python 3.
- This method was used before f-strings were introduced in Python 3.6
- The overloaded modulo operator can be used to perform string formatting.
- The modulo operator % is overloaded by the string class of Python.
- This is the reason it is also called string modulo operator.
- The syntax of the string modulo operator is:
<format_string> % <values>
- where
<format_string>
is the string containing conversion specifiers.<values>
are the actual values that replace the conversion specifiers in the string.
- If there are more than one values to be replaced, they should be written in a Tuple.
- Single values can be written as it is.
- Each value from the Tuple of is converted to string by Python interpreter.
- The resultant string is then inserted into the format string.
- String modulo operator is not just for printing. It can be used for formatting strings and assigning to variable.
Conversion Specifier
- A conversion specifier indicates the location in the format string where the corresponding values will be inserted.
- A conversion specifier begins with a % sign.
- There are different components of a conversion specifier that appear in a certain order.
- The components of the conversion specifier determine how the values will be formatted before they are inserted into the format string.
- The general syntax of conversion specifier is :
%[flags][width][.precision]<type>
- the % character and the
<type>
component are required components. - the flags, width and precision are optional components.
- the % character and the
- Below is the functioning of each component of a conversion specifier :
- % => Marks the beginning of the conversion specifier
- <flags> => Mentions the flags that are used for better formatting
- <width> => Determines the minimum width of the formatted result.
- .<precision> => Length and precision of floating-point or string output.
- <type> => This component tells the type of conversion to be performed.
- Example :
print("%d %s weigh %.2f Pounds" % (10, "apples", 2.567))
# Output
# 10 apples weigh 2.57 Pounds
Conversion types:
The <type>
component indicates the type of conversion that will be applied to the corresponding value before it is inserted in the format string. The table below lists the possible type conversions :
d, i, u | Decimal integer |
x, X | Hexadecimal integer |
o | Octal integer |
f, F | Floating-point |
e, E | E notation |
g, G | Floating-point or E notation |
c | Single character |
s, r, a | String |
% | Single '%' character |
Prev. Tutorial : Using f-strings
Next Tutorial : Using format method