Introduction
Python is a versatile programming language renowned for its simplicity and readability. In Python, we often deal with different types of data such as numbers, strings, lists, and tuples. It is essential to understand the fundamentals of type conversion to manipulate data and perform operations effectively. Type conversion, also known as typecasting, is the process of converting an object of one data type into another type. Python offers built-in functions for type conversion, making it convenient for developers to convert data types easily. In this Python tutorial, we will explore the concept of type conversion in Python, its importance in programming, and several methods of converting data types in Python.
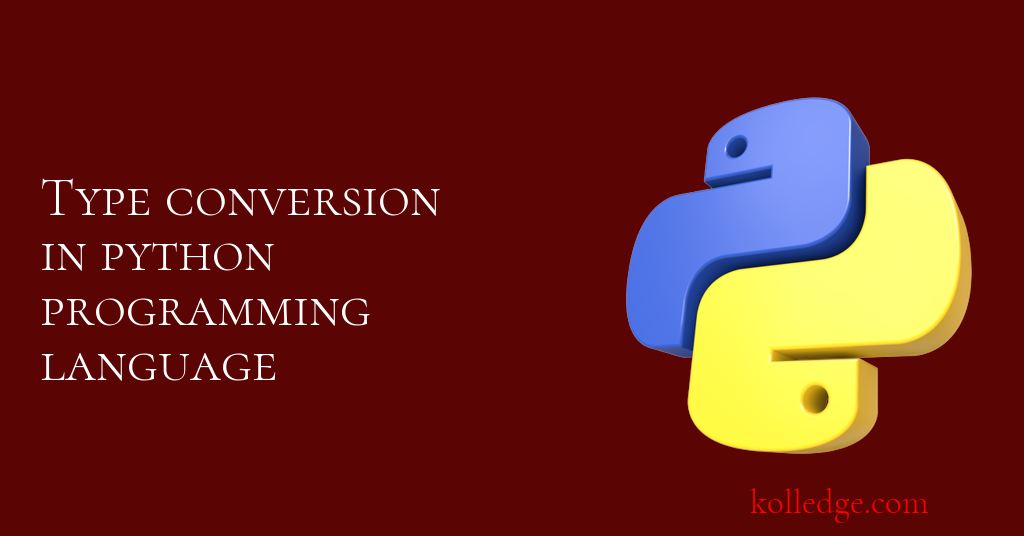
Table of Contents :
- Type conversion
- Implicit type Conversion in Python
- Explicit Type conversion in Python
Type conversion
- In Python, type conversion is the process of converting one data type to another.
- For example, Converting an integer to a string.
- There are two ways to convert data types in Python:
- Implicit Type conversion
- Explicit Type Conversion
Implicit type Conversion in Python
- This is the automatic type conversion.
- In some situations Python automatically converts one data type to another.
- In implicit type conversion lower data type is promoted to higher data type.
- This conversion is done to avoid data loss.
- a couple of examples of implicit type conversion are :
- When we add int and float - the result is float.
- or when we reassign an
int
variable withfloat
value, the data type of the variable changes.
- Implicit type conversion is not possible with strings and int.
- Code Sample :
x = 10
print("Data type of x = ", type(x))
x = 5.5
print("Data type of x = ", type(x))
# Output
# Data type of x = <class 'int'>
# Data type of x = <class 'float'>
Explicit Type conversion in Python
- This is the manual type conversion.
- In explicit type conversion built-in functions are used to convert data from one type to another.
- Explicit type conversion is also called Type casting.
- There is a risk of data loss in explicit type conversion.
- Following built-in functions are used for type casting:
- int() - Converts a value to an integer
- float() - Converts a value to a floating point number
- str() - Converts a value to a string
- bool() - Converts a value to a Boolean value (True or False)
- list() - Converts a value to a list
- tuple() - Converts a value to a tuple
- dict() - Converts a value to a dictionary
- set() - Converts a value to a set
- ord() : Converts a character to integer.
- hex() : Converts an integer to hexadecimal string.
- oct() : Converts an integer to octal string.
- complex(real,imag) : Converts real numbers to complex(real,imag) number.
- chr(number): Converts number to its corresponding ASCII character.
- Let's look at some examples of how to use these functions.
- Converting an integer to a floating point number:
int(5.5) # returns 5
float(5) # returns 5.0
- Converting a string to an integer:
int('5') # returns 5
str(5) # returns '5'
- Converting a string to a floating point number:
float('5.5') # returns 5.5
str(5.5) # returns '5.5'
- Converting a Boolean value to a string:
bool(True) # returns True
str(True) # returns 'True'
- Converting a list to a tuple:
list([1, 2, 3]) # returns [1, 2, 3]
tuple([1, 2, 3]) # returns (1, 2, 3)
- Converting a tuple to a list:
tuple((1, 2, 3)) # returns (1, 2, 3)
list((1, 2, 3)) # returns [1, 2, 3]
- Converting a dictionary to a set:
dict({1: 'one', 2: 'two', 3: 'three'})
# returns {1: 'one', 2: 'two', 3: 'three'}
set({1: 'one', 2: 'two', 3: 'three'})
# returns {1: 'three', 2: 'two', 1: 'one'}
Prev. Tutorial : Arrays in Python
Next Tutorial : Conditional statements