Introduction
In Python programming language, a string is a sequence of characters. Strings are an essential data type used for storing and manipulating textual information like words, sentences, and paragraphs. They are incredibly versatile and used in almost every Python program. String manipulation is also an essential skill for any aspiring Python developer. In this tutorial, we will explore strings in Python, including string syntax, string operations, and string formatting. By the end of this tutorial, you will have a clear understanding of the string data type concepts and how to use them effectively in Python.
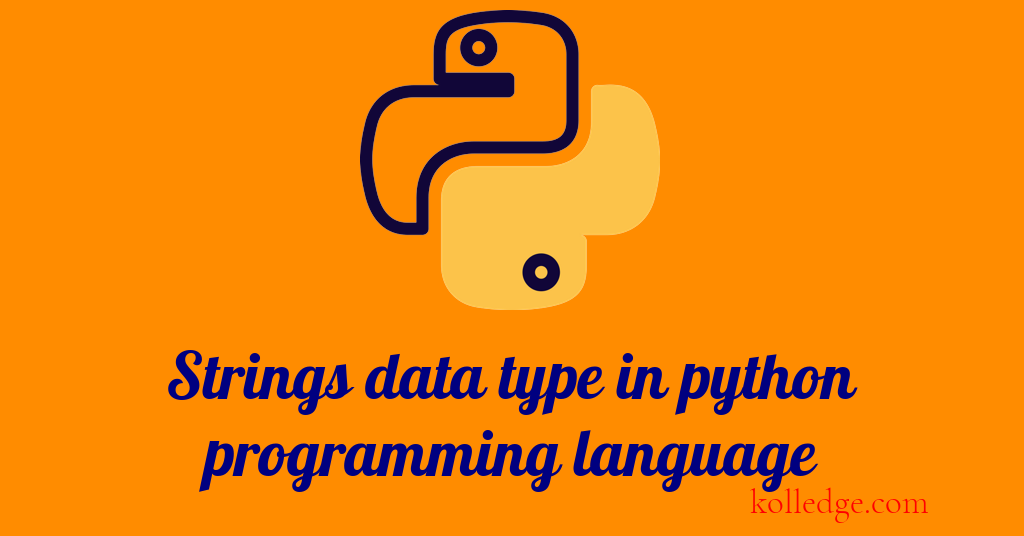
Table of Contents :
- What are Strings
- Accessing characters of a string
- Accessing a Range of characters of string
- Using negative indexes to access character of string
- Strings are immutable
- Common String Operations in Python
What are Strings
- In Python, a string is a sequence of characters.
- Strings are immutable, meaning they can't be changed once they're created.
- Example :
my_str = "Kolledge Scholars!!"
Accessing characters of a string
- We can access the characters in a string using indexes.
- String indexes start at 0. i.e.
- the first character in a string has an index of 0,
- the second character has an index of 1,
- and so on.
- To access a character at a particular index, we use square brackets after the string name.
- Code Sample :
my_str = "abcdef"
print(my_str[0])
print(my_str[1])
# Output
# a
# b
Accessing a Range of characters of string
- To get a range of characters, we can use a colon within square brackets.
- Basic syntax of accessing range of characters :
str_name[start_index : stop_index]
- The character at
stop_index
is not included in the resultant sub-string. - Code Sample :
my_str = "abcdef"
print(my_str[2:5])
# Output
# prints 'cde'
# This gets the characters from index 2 to index 4
# (not including index 5).
- omitting the
start_index
- If we omit the first number i.e
start_index
, it defaults to 0. - Code Sample :
my_str = "abcdef"
print(my_str[:5])
print(my_str[0:5])
# Output
# abcde
# abcde
# Both the statements are equivalent
- omit the
stop_index
- If we omit the second number i.e
stop_index
, it defaults to the length of the string. - Code Sample :
my_str = "abcdef"
print(my_str[2:])
print(my_str[2:len(my_str)])
# Output
# cdef
# cdef
# Both the statements are equivalent
Using negative indexes to access character of string
- We can also use negative indexes to access characters of a string.
- These count from the end of the string.
- So the last character has index -1,
- the second to last character has index -2, and so on.
- Code Sample :
my_str = "abcdef"
print(my_str[-1]) # prints 'f'
print(my_str[-2]) # prints 'e'
# Output
# f
# e
- negative indexes in range
- We can use negative indexes in ranges too
- Code Sample :
my_str = "abcdef"
print(my_str[-3:-1])
# Output
# de
# This gets the characters from index -3 to index -2.
# (not including index -1).
Strings are immutable
- Strings in python is an immutable Data type i.e. once created, strings cannot be modified.
- If you want to change a string, you need to create a new string.
- For example, if we want to replace all occurrences of 'a' with 'X', we could :
- Create a new string string having 'X' wherever there was 'a'
- Use the replace method - The replace() method returns a new string, it doesn't modify the original string.
- Let's try to understand immutability in python
- Once we have created an string literal we cannot change it's contents.
- We can assign this string literals to variable.
- We can reassign new string literals to the same variable.
- But We cannot change the individual characters within the string literal.
- Code Sample :
# Assigning a string to a variable
msg = "Kolledge Scholars!!"
print(msg)
# Output
# Kolledge Scholars!!
# Assigning new string literal to the same variable
msg = "hello Kolledge Scholars!!"
print(msg)
# Output
# hello Kolledge Scholars!!
# Accessing the characters of the string literal
c = msg[0]
print(c)
# Output
# h
# Trying to change the value of string literal
# This code gives error as strings are immutable
msg[0] = "H"
print(msg)
# Output
# TypeError: 'str' object does not support item assignment
Common String Operations in Python
Few string operations that we'll see here are -
- Concatenating Strings
- Repeat a string
- Check Sub-string
- Convert whole string to Uppercase
- Convert whole string to Lowercase
- Strip a string
Concatenating Strings in Python
- If we want to concatenate strings, we can use the Addition operator (+ operator ) :
- The
+
operator here is called the string concatenation operator. - Code Sample :
string1 = "Hello!!!"
string2 = "Kolledge Scholars"
string3 = string1 + " " + string2
print(string3)
# Output
# Hello!!! Kolledge Scholars
Repeating a string in Python
- If we want to repeat a string, we can use the
*
operator: - Code Sample :
string1 = "Hello_"
string2 = string1 * 3
print(string2)
# Output
# Hello_Hello_Hello_
Check Sub-strings in Python
- We can check if a string contains a particular character or sub-string using the
in
operator : - Code Sample :
string1 = "Hello"
p = 'e' in string1
q = 'x' in string1
print (p)
print (q)
# Output
# True
# False
Convert Strings to Uppercase or Lowercase
- We can convert a string to all upper case or all lower case using the upper() and lower() methods:
- Code Sample :
string1 = "Hello"
string2 = "WORLD"
string3 = string1.upper()
string4 = string2.lower()
print(string3)
print(string4)
# Output
# HELLO
# world
Strip a string in Python
- We can strip leading and trailing white-spaces from a string using the strip() method:
- Code Sample :
string1 = " abcdef "
string2 = string1.strip()
print(string2)
# Output
# abcdef
Prev. Tutorial : Complex Data Type
Next Tutorial : List data type