Beginner Level
Intermediate Level
Advanced Level
Introduction
In Python, everything is an object and objects are either mutable or immutable. Mutable objects can be changed, while immutable objects cannot be changed once they are created. Understanding the difference between these two types of objects is essential for writing efficient and effective Python code. This tutorial will dive deeper into the concepts of mutable and immutable objects in Python and provide examples to illustrate their usage. By the end of this tutorial, you will have a solid understanding of how to work with both types of objects in Python.
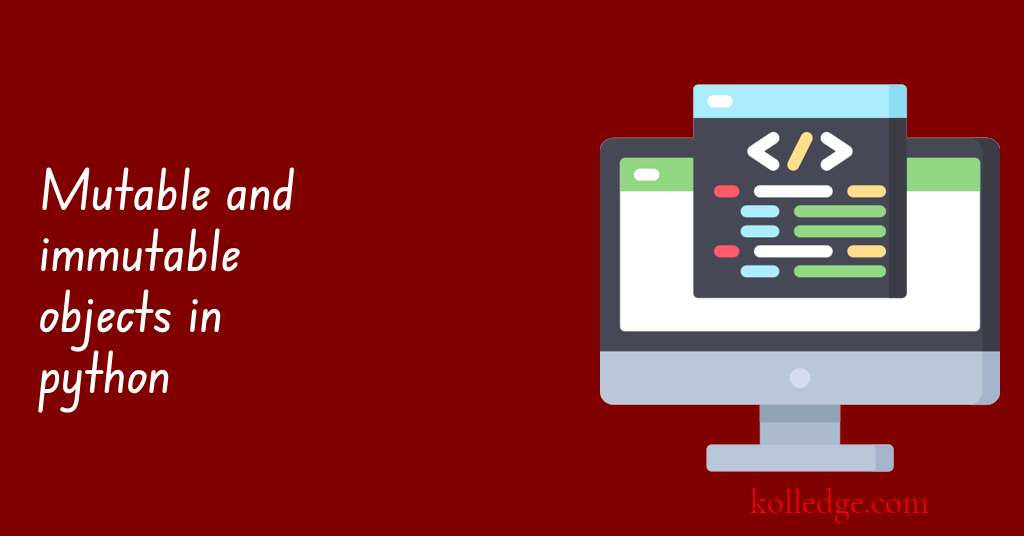
Table of Contents :
- Introduction to Mutable and Immutable Objects
- Example of Python Immutable Objects
- Example of Python mutable Objects
- Passing Arguments to Python Functions
Introduction to mutable and immutable objects
- Python data types can be categorized as mutable or immutable.
- Mutable objects can be modified while immutable objects cannot be changed once created.
- Understanding the difference between mutable and immutable objects is important for efficient programming in Python.
Example of python immutable Objects :
- Examples of immutable data types in Python include : numbers, tuples, and strings.
- Once an immutable object is created, it cannot be changed.
- Any operations on the object will return a new object rather than modifying the original.
- Code Sample :
a = 5
b = a # b is assigned the value of a
a = a + 1 # a is incremented, but b remains unchanged
print(a) # Output: 6
print(b) # Output: 5
name = "John"
new_name = name.upper() # upper() method returns a new string
print(name) # Output: John
print(new_name) # Output: JOHN
Example of Python mutable Objects :
- Examples of mutable data types in Python include : lists, sets and dictionaries.
- Mutable objects can be modified in place which means the original object can be updated directly.
- Code Sample :
students = ['John', 'Amy', 'Mike']
students.append('Sarah') # 'Sarah' added to end of list
print(students) # Output: ['John', 'Amy', 'Mike', 'Sarah']
grades = {'John': 90, 'Amy': 85, 'Mike': 95}
grades['John'] = 95 # 'John' grade updated to 95
print(grades) # Output: {'John': 95, 'Amy': 85, 'Mike': 95}
Passing Arguments to Python Functions
- Knowing the mutability of different data types is important when passing arguments to functions.
- Immutable objects are passed by value which means a copy of the object is passed to the function.
- Mutable objects are passed by reference which means the function receives a pointer to the original object.
- Code Sample :
def double(num):
num = num * 2 # num is a local copy, original value outside the function is not changed
a = 5
double(a)
print(a) # Output: 5
def append_name(students_list):
students_list.append('Sarah')
students = ['John', 'Amy', 'Mike']
append_name(students)
print(students) # Output: ['John', 'Amy', 'Mike', 'Sarah']
Prev. Tutorial : Garbage collection
Next Tutorial : None Value