Beginner Level
Intermediate Level
Advanced Level
Introduction
One common use case for Python is interacting with databases, and in this tutorial, we will focus specifically on connecting to a MySQL database using Python. MySQL is one of the most widely used relational database management systems, making it an important tool to know for anyone working with data. By the end of this tutorial, you will have a better understanding of how to establish a connection to a MySQL database using Python and be equipped with the skills to retrieve, update, and manipulate data within it.
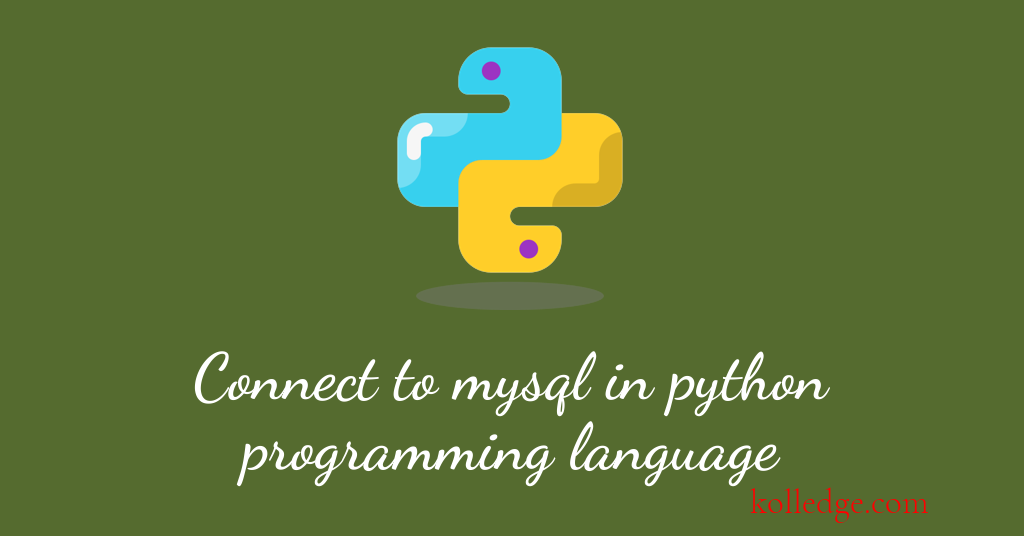
Table of Contents :
- How to connect to MySQL with Python
- Step 1: Install the required Packages
- Step 2: Establishing a Connection
- Connecting to MySQL using mysql.connector
- Connecting to MySQL using PyMySQL
How to connect to MySQL with Python :
- Follow the steps given below to connect to MySQL with python
- Step 1: Install the required Packages
- Step 2: Establishing a Connection
- We can connect to MySQL using
- mysql-connector or
- PyMySQL.
Step 1: Install the Required Packages
- The first step is to ensure that you have installed the required packages on your machine.
- The most popular packages for connecting to MySQL in Python are
- mysql-connector and
- PyMySQL.
- You can install these packages using pip through the command line.
- Code Sample :
!pip install mysql-connector-python
!pip install PyMySQL
Step 2: Connect to the MySQL Database
- To connect to a MySQL database in Python we can use either
- mysql.connector or
- PyMySQL package
- We need to provide the necessary connection parameters to establish the connection.
- These parameters normally include the host, user, password, and database name.
- We can establish a connection to the database by creating a new Connection object.
- We can pass in the following parameters to the Connection object:
- host : the hostname of the MySQL server user
- user : username for the MySQL account
- password : password for the MySQL account database
- database : Db name to connect to
Connecting to MySQL using mysql.connector :
- Here we have a code sample of connecting to MySQL using mysql.connector
import mysql.connector
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="mydatabase"
)
print(mydb)
Connecting to MySQL using PyMySQL :
- Here we have a code sample of connecting to MySQL using PyMySQL
import pymysql
mydb = pymysql.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="mydatabase",
cursorclass=pymysql.cursors.DictCursor
)
print(mydb)
Prev. Tutorial : Python for mysql
Next Tutorial : Create mysql database using Python