Introduction
Method Overriding is an important concept in object-oriented programming, and is a technique used to modify the behavior of a method defined in the parent class in the child class. This allows for greater flexibility and customization of code, as well as the ability to extend and enhance the functionality of existing programs without having to start from scratch. In Python, method overriding is a relatively simple process, and can be accomplished with just a few lines of code. In this tutorial, we will explore the basics of method overriding in Python, and how you can use it to create more powerful and flexible classes and objects.
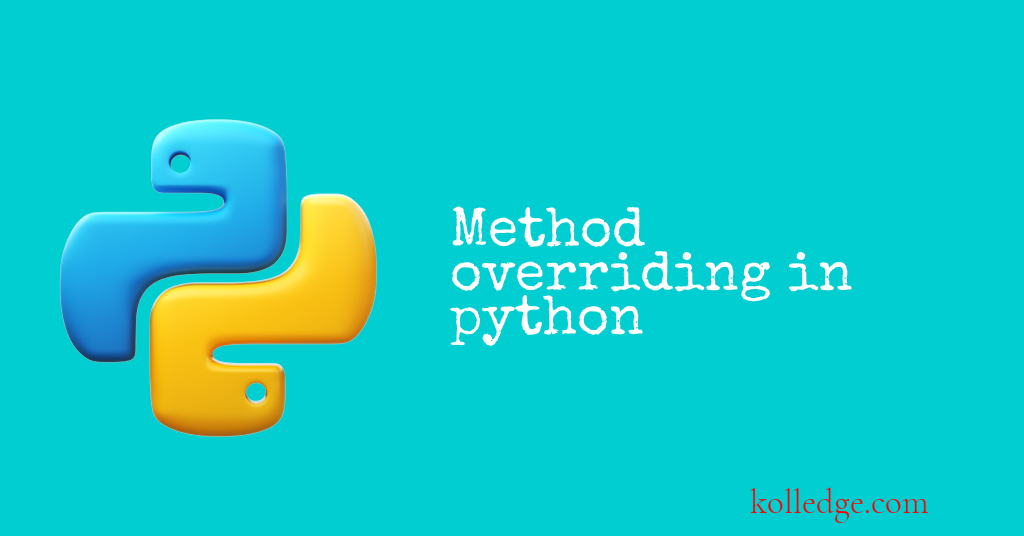
Table of Contents :
- What is Method overriding?
- Syntax
What is Method overriding?
- Method overriding in Python is a feature that allows a subclass to implement a method with the same name as that of the superclass.
- The method in the subclass will override the method in the superclass.
- Method overriding is used to modify the behavior of a method in the subclass without changing the behavior of the same method in the superclass.
Syntax:
- To override a method in a subclass, the method in the superclass must be defined first.
- The syntax to override a method in a subclass is as follows:
class Superclass:
def method_name(self, params):
# code
class Subclass(Superclass): # inheritance from superclass
def method_name(self, params): # overriding the superclass method
# modified code
Example: Method Overriding
class Animal:
def speak(self):
print("This animal speaks.")
class Dog(Animal):
def speak(self):
print("This dog barks.")
class Cat(Animal):
def speak(self):
print("This cat meows.")
a = Animal()
a.speak()
# Output: This animal speaks.
d = Dog()
d.speak()
# Output: This dog barks.
c = Cat()
c.speak()
# Output: This cat meows.
Example: Calling the Superclass Method
class Animal:
def speak(self):
print("This animal speaks.")
class Dog(Animal):
def speak(self):
super().speak()
print("This dog barks.")
class Cat(Animal):
def speak(self):
super().speak()
print("This cat meows.")
d = Dog()
d.speak()
# Output: This animal speaks. \n This dog barks.
c = Cat()
c.speak()
# Output: This animal speaks. \n This cat meows.
Example: Accessing the Superclass Method
class Animal:
def __init__(self, species):
self.species = species
class Dog(Animal):
def __init__(self, name, breed):
self.name = name
self.breed = breed
super().__init__("Dog")
def speak(self):
print("This dog barks.")
d = Dog("Rover", "Labrador")
print(d.species)
# Output: Dog
Prev. Tutorial : Super() function
Next Tutorial : Method Resolution Order