Beginner Level
Intermediate Level
Advanced Level
Introduction
Moving files and directories in Python is a common task in file management tasks, and it can be accomplished easily and efficiently with the os module. In this tutorial, we will explore the different ways in which we can move files and directories using Python. We will learn about the os module, its different methods, and how to use them to move files and directories. We will also look at some practical examples to understand the concepts better. So, let's dive into the world of Python file handling and learn how to move files and directories using Python.
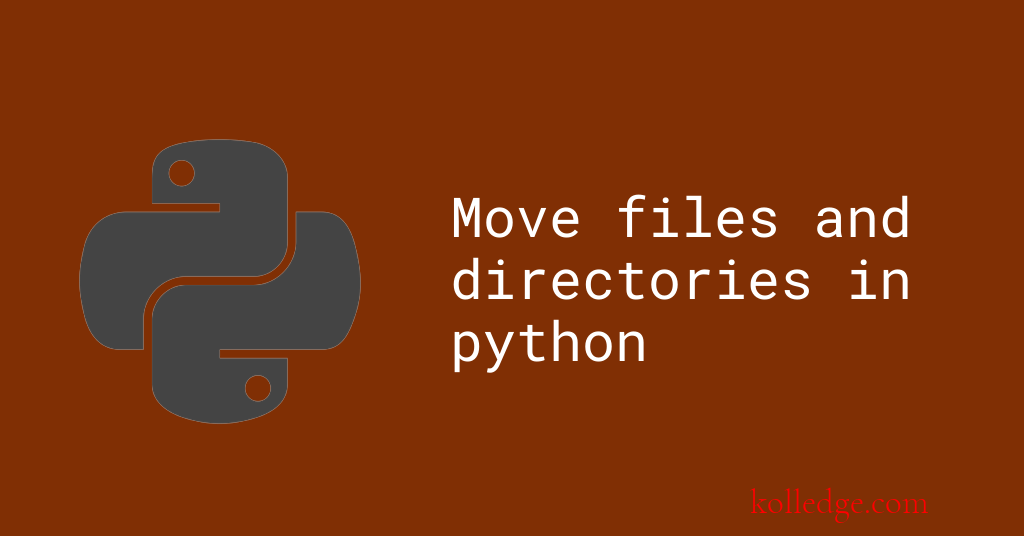
Table of Contents :
- Moving a file in Python
- How to move multiple files in Python
- How to move all files from a directory in Python
- How to move files matching a pattern
- How to move files based on file extension
- How to move files based on filename
- How to move file and rename in Python
Moving a file in Python
- Use the
os
module'srename()
method to move a file from a source path to a destination path. - Code Sample :
import os
source_file = "/path/to/source/file"
destination_file = "/path/to/destination/file"
os.rename(source_file, destination_file)
How to move multiple files in Python
- Use the
shutil
module'smove()
method to move multiple files from a source directory to a destination directory. - Code Sample :
import shutil
source_directory = "/path/to/source/directory"
destination_directory = "/path/to/destination/directory"
for file_name in os.listdir(source_directory):
source = os.path.join(source_directory, file_name)
destination = os.path.join(destination_directory, file_name)
shutil.move(source, destination)
How to move all files from a directory in Python :
- Use the
shutil
module'smove()
method to move all files from a source directory to a destination directory. - Code Sample :
import os
import shutil
source_directory = "/path/to/source/directory"
destination_directory = "/path/to/destination/directory"
for file_name in os.listdir(source_directory):
source = os.path.join(source_directory, file_name)
destination = os.path.join(destination_directory, file_name)
if os.path.isfile(source):
shutil.move(source, destination)
How to move files matching a pattern (Wildcard) :
- Use the
glob
module's to move files from a source directory to a destination directory that match a specific pattern (wildcard). - Code Sample :
import glob
import os
source_directory = "/path/to/source/directory"
destination_directory = "/path/to/destination/directory"
for file_name in glob.glob(os.path.join(source_directory, "*.txt")):
shutil.move(file_name, destination_directory)
How to move files based on file extension
- Use the
shutil
module'smove()
method to move files from a source directory to a destination directory based on their file extensions. - Code Sample :
import os
import shutil
source_directory = "/path/to/source/directory"
destination_directory = "/path/to/destination/directory"
for file_name in os.listdir(source_directory):
if file_name.endswith(".txt"):
source = os.path.join(source_directory, file_name)
destination = os.path.join(destination_directory, file_name)
shutil.move(source, destination)
How to move files based on filename
- Use the
shutil
module'smove()
method to move files based on their filename from a source directory to a destination directory. - Code Sample :
import os
import shutil
source_directory = "/path/to/source/directory"
destination_directory = "/path/to/destination/directory"
for file_name in os.listdir(source_directory):
if "sample" in file_name:
source = os.path.join(source_directory, file_name)
destination = os.path.join(destination_directory, file_name)
shutil.move(source, destination)
How to move file and rename in Python :
- Use the
os
module'srename()
method to move a file from a source path to a destination path and rename the file in the process. - Code Sample :
import os
source_file = "/path/to/source/file"
destination_file = "/path/to/destination/file2"
os.rename(source_file, destination_file)
Prev. Tutorial : Copy Files and Dir.
Next Tutorial : What is json