Beginner Level
Intermediate Level
Advanced Level
Introduction
If you are new to programming or just getting started with Python, you have probably encountered some errors and exceptions along the way. These errors can cause your program to stop running or produce unexpected results. However, with exception handling, you can gracefully handle these errors and ensure that your program continues to run smoothly. In this tutorial, we will explore what exception handling is and how it works in Python programming language.
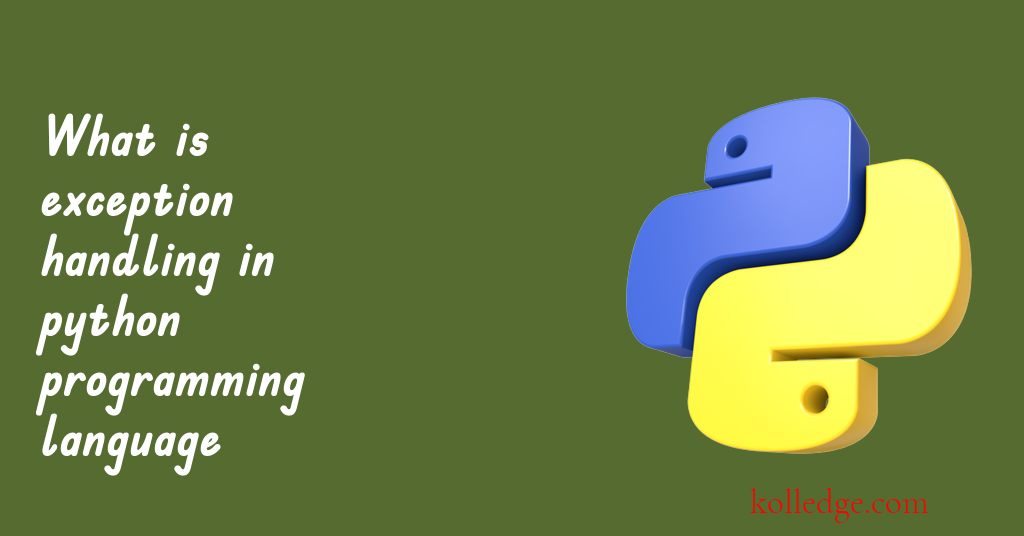
Table of Contents :
- Exception handling in Python
- What are syntax errors
- What are Exceptions
- What is Exception handling
Exception handling in Python
- In a programming language there are two types of errors -
- Syntax Error
- Exceptions
What are syntax errors :
- Syntax error are the errors that occur due to writing invalid code.
- Some examples of syntax errors can be
- if we forget to put a parenthesis after function call.
- if we forget to put a colon after if statement in python.
- if we do not indent the code properly in python.
- As the name suggests these errors occur due to some mistake in the syntax of the code.
- Python interpreter catches syntax errors before executing the program.
- Code Sample :
# There is no semi-colon after function definition
# This will produce syntax error before executing the program
def my_function(val_1)
print("Value 1 = ", val_1)
my_function(10)
# Output
# def my_function(val_1)
^
# SyntaxError: invalid syntax
What are Exceptions :
- Exceptions are the errors that occur during the execution of the program.
- There can be many reasons that can cause exceptions :
- these can be resource issues like some file that we want to access in our code is missing.
- environment issues like database is not establishing connection with the code.
- network issues can cause exceptions as we cannot connect to some remote resource.
- logical errors can cause exceptions e.g. during the execution we end up dividing a number by zero.
- Exceptions are not handled automatically by python interpreter.
- When an exception occurs the code execution halts abruptly and an error message is shown by the interpreter.
- When an exception occurs the rest of the code of the program is not executed by the interpreter.
- In the code sample below we can see that the execution of the code starts normally and the line
Inside my_function
is printed normally. - After that an exception occurs and the rest of the code is not executed.
- Code Sample :
def my_function(x, y):
print("Inside my_function")
# This statement can produce exception
# If the denominator resutls to zero
z = (x * y)/ (x + y)
print("Value of z = ", z)
my_function(10, -10)
# Output
# Inside my_function
# ZeroDivisionError: division by zero
What is Exception handling
- Ideally when an exception occurs a message should be displayed to the user and the rest of the code should continue to executed smoothly.
- This is what exception handling is used for in any programming language.
- When we implement exception handling - whenever an exception occurs, a message is shown to the user and the code continue to execute normally for the rest part.
- Also exception handling helps us in displaying error messages which are more readable and informative so that user can fix the fault easily.
- Exception handling is done in two ways in Python:
- Using try and except statements
- Using the raise statement
Prev. Tutorial : Issubclass() function
Next Tutorial : Try-except blocks