Beginner Level
Intermediate Level
Advanced Level
Introduction
Daemon threads are threads that run in the background of a program and do not require explicit termination. This tutorial will delve into the topic of daemon threads in Python, providing a comprehensive overview of what they are and how they work. Readers will learn how to create and manage daemon threads, as well as understand their importance in various programming contexts. Whether you are a beginner or an experienced Python developer, this tutorial is a valuable resource for mastering the mechanics of daemon threads.
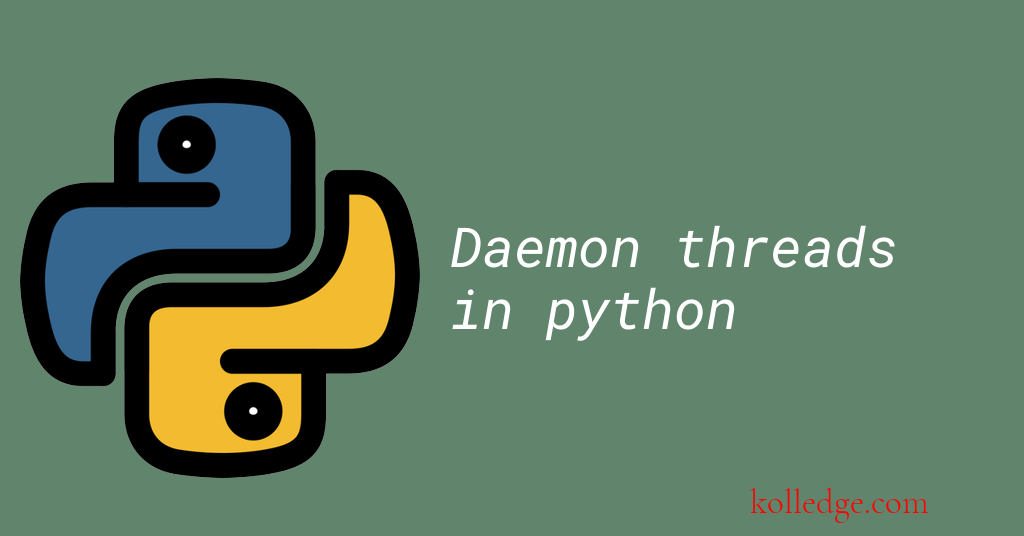
Table of Contents :
- What are Daemon Threads in python
- Creating a Daemon Thread in python
- Daemon Threads vs. Non-Daemon Threads
What are Daemon Threads in python :
- In Python, daemon threads are a type of thread that runs in the background
- daemon threads are used to perform tasks that do not require a user's intervention.
- A daemon thread automatically stops running when all other non-daemon threads have completed.
- Daemon threads can be useful for performing background tasks, such as system monitoring, log file processing, and other similar tasks.
Creating a Daemon Thread in python :
- In Python, you can create a daemon thread by setting the
daemon
attribute of the thread toTrue
before starting it. - A daemon thread can also be created by subclassing the
threading.Thread
class and implementing therun()
method as usual. - Code Sample :
import threading
import time
def daemon():
while True:
print("Daemon thread is running.")
time.sleep(1)
# Creating a daemon thread using the setDaemon() method
d = threading.Thread(target=daemon)
d.setDaemon(True)
d.start()
# Wait for user input
input("Press any key to exit.\n")
Explanation :
- Here, we create a daemon thread
d
using thethreading.Thread
class and - the
daemon()
function as its target. - We then set the
daemon
attribute toTrue
using thesetDaemon()
method and - start the thread using the
start()
method. - The program waits for user input before terminating, allowing the daemon thread to continue running in the background.
Daemon Threads vs. Non-Daemon Threads :
- When a Python program exits, all non-daemon threads are stopped, even if they are still running.
- Daemon threads, on the other hand, are automatically stopped when the program exits, regardless of whether they have completed running or not.
- Daemon threads can also be used to ensure that background tasks are terminated if the main program encounters an error or is otherwise terminated unexpectedly.
Prev. Tutorial : Stopping a thread
Next Tutorial : Thread-safe Queue