Introduction
In object-oriented programming, methods are defined within classes to perform specific operations on the class' data members. However, not all methods depend on specific instances of the class and can be called independent of any object or instance. These are known as static methods. In Python, static methods are defined using the `@staticmethod` decorator and can be accessed using the class name itself. In this tutorial, we will explore the concept of static methods in Python programming language, their syntax, uses, and limitations with some practical examples to help you understand how they can be used to make your code more efficient and organized.
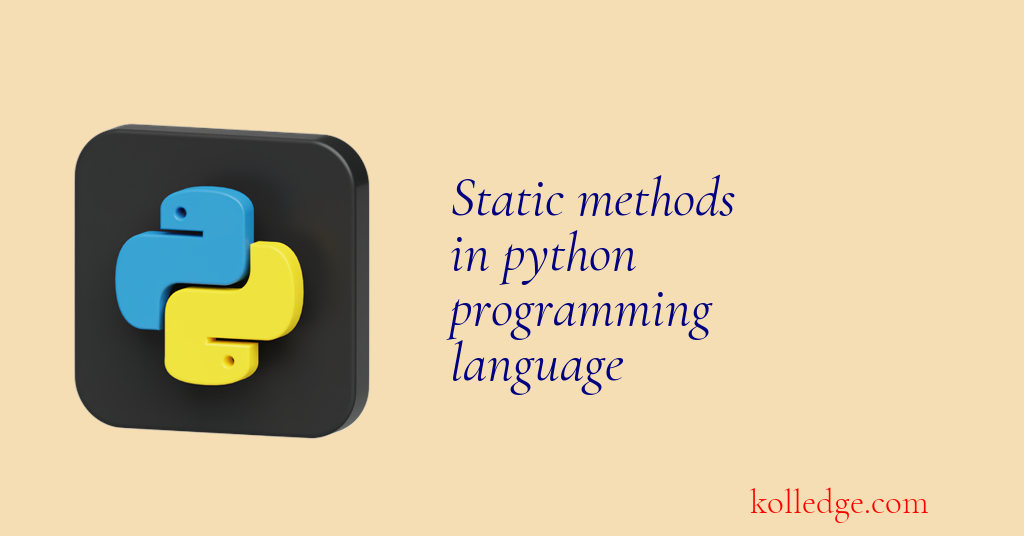
Table of Contents :
- Static methods in Python
- Defining a static method
- Defining static methods by using the @staticmethod decorator
- Defining static methods by using the staticmethod() function
- Accessing a static method
Static methods in Python
- Static methods are utility methods that are written to perform a particular task.
- Static methods are attached to the class itself.
- Static methods do not have access to class and instance variables.
- Static methods cannot modify class state or object state. They just perform some utility actions.
- Static methods do not take
cls
orself
as the first parameter. - Static methods take less memory than instance methods as only one copy of static method is created for all objects.
Defining a static method :
- The syntax of defining a static method is same as defining regular functions.
- The basic syntax of defining class methods is :
def method_name(arg1, arg2,...):
# method body
- By default, any method created inside a class is an instance method.
- If we want to define a static method, we need to explicitly indicate this in two ways. By using :
- @staticmethod decorator
- staticmethod() function
Defining static methods by using the @staticmethod decorator :
- By default, any method created inside a class is an instance method.
- We can tell python interpreter that the function inside the class is a static method by adding a @staticmethod decorator before the function definition
- Code Sample :
class Employee:
def __init__(self, emp_name, emp_id, emp_desig):
self.name = emp_name
self.id = emp_id
self.desig = emp_desig
@staticmethod
def show_company(cmp_name):
print(f"The name of the company is : {cmp_name}")
def print_details(self):
print(f"Name of employee = {self.name}")
print(f"ID of employee = {self.id}")
print(f"Designation of employee = {self.desig}")
print()
emp_1 = Employee("Steve", "k2591", "Manager")
emp_1.print_details()
# Calling the static method
Employee.show_company("ABC Inc.")
# Output
# Name of employee = Steve
# ID of employee = k2591
# Designation of employee = Manager
# The name of the company is : ABC Inc.
Defining static methods by using the staticmethod() function :
- This is the older method of creating static methods.
- We can convert a normal function into a static method by using the built-in
staticmethod()
function. - The basic syntax of using
staticmethod()
function is :staticmethod(function_name)
- Code Sample :
class Employee:
def __init__(self, emp_name, emp_id, emp_desig):
# data members (instance variables - name, id and desig)
self.name = emp_name
self.id = emp_id
self.desig = emp_desig
def show_company(cmp_name):
print(f"The name of the company is : {cmp_name}")
def print_details(self):
print(f"Name of employee = {self.name}")
print(f"ID of employee = {self.id}")
print(f"Designation of employee = {self.desig}")
print()
emp_1 = Employee("Steve", "k2591", "Manager")
emp_1.print_details()
# Creating a static method
Employee.show_company = staticmethod(Employee.show_company)
# Calling the static method
Employee.show_company("ABC Inc.")
# Output
# Name of employee = Steve
# ID of employee = k2591
# Designation of employee = Manager
# The name of the company is : ABC Inc.
Accessing a static method :
- static methods can be accessed :
- using the class name and dot operator.
- using the object name and dot operator.
- The syntax of accessing a static method using class name is :
class_name.static_method_name()
- The syntax of accessing a static method using object name is :
object_name.static_method_name()
- Code Sample :
class Employee:
def __init__(self, emp_name, emp_id, emp_desig):
self.name = emp_name
self.id = emp_id
self.desig = emp_desig
@staticmethod
def show_company(cmp_name):
print(f"The name of the company is : {cmp_name}")
print()
emp_1 = Employee("Steve", "k2591", "Manager")
# Calling the static method using class name
Employee.show_company("ABC Inc.")
# Calling the static method using object name
emp_1.show_company("ABC Inc.")
# Output
# The name of the company is : ABC Inc.
# The name of the company is : ABC Inc.
Prev. Tutorial : Class methods
Next Tutorial : Class vs. Static vs. Instance