Beginner Level
Intermediate Level
Advanced Level
Introduction
In Python programming language, the try-except block is quite commonly used to handle exceptions and error handling in code. However, there are times when we need to execute a piece of code regardless of whether there is an exception or not. This is where the finally block comes into play. The finally block is executed after the try and except blocks but before the code continues to the next statement. In this tutorial, we will cover the basics of the finally block in Python and how to use it to handle exceptions, clean up resources, and other important use cases.
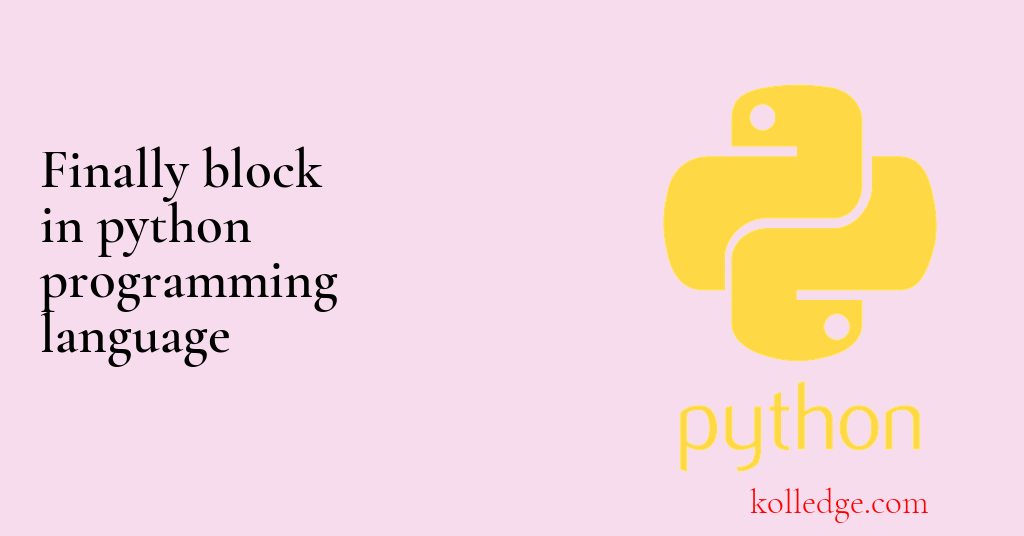
Table of Contents :
- try..except..finally construct in Python
- using finally block without except block
- Uses of finally block
try..except..finally construct in Python
- We have already read how try...except construct is used to handle exceptions in Python.
- There is an optional
finally
clause of the try..except statement. - The code in the finally block is always executed.
- The code in the
finally
block is executed, whether an exception occurs or not. - If there is no exception, the code in the
finally
block is executed after all the statements oftry
block. - If exception occurs, the code in the
finally
block is executed after the code of theexcept
block. - In the code sample below we see that the
finally
block is always executed. - Code Sample :
def my_function(x, y):
try:
print("Inside try block")
z = (x * y) / (x + y)
print("Value of z = ", z)
except Exception as e:
print("Inside except block : ", e)
finally:
print("Inside Finally block")
my_function(5, -5)
print()
my_function(4, 1)
# Output
# Inside try block
# Inside except block : division by zero
# Inside Finally block
# Inside try block
# Value of z = 0.8
# Inside Finally block
using finally block without except block
- We can omit the
except
clause if we choose to write thefinally
clause in thetry..except..finally
statement. - Even if we do not write the
except
clause, the code in thefinally
block will always be executed - If we do not write the
except
clause, exceptions will not be handled gracefully. - If we do not write the
except
clause and an exception occurs,- the code in the
finally
block will be executed and - then the code execution will halt abruptly.
- the code in the
- Code Sample :
def my_function(x, y):
try:
print("Inside try block")
z = (x * y) / (x + y)
print("Value of z = ", z)
finally:
print("Inside Finally block")
my_function(5, -5)
# Output
# Inside try block
# Inside Finally block
# ZeroDivisionError: division by zero
Uses of finally block
- The
finally
block can be used for tasks like- cleaning up resources like - end connection from database, close any open file etc.
- printing a message to user before program ends, even if there is an exception.
Prev. Tutorial : Try-except blocks
Next Tutorial : Try..except..else