Beginner Level
Intermediate Level
Advanced Level
Introduction
Bitwise operators in Python programming language are a set of operators that manipulate the individual bits of a value. These operators are used to perform low-level operations with efficiency and precision. Learning the concepts of bitwise operators in Python can help programmers build high-performance, scalable programs, particularly in fields like machine learning, cryptography, and network programming. In this comprehensive tutorial, we will explore the fundamentals of bitwise operations in Python, their applications, and their syntax.
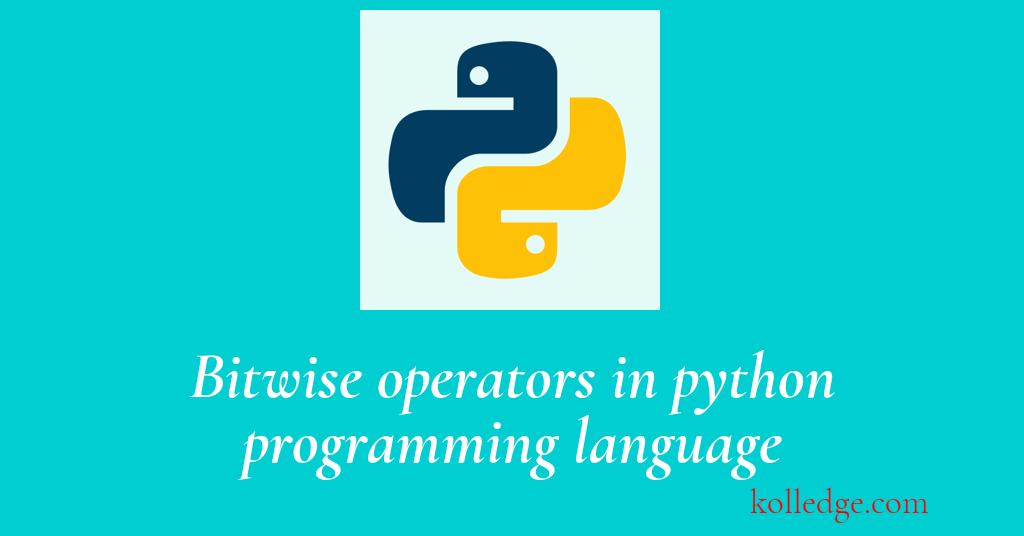
Table of Contents :
- What are Bitwise Operators?
- Different Bitwise operators in Python
- Bitwise and operator
- Bitwise or operator
- Bitwise xor operator
- Bitwise 1’s complement operator
- Bitwise left-shift operator
- Bitwise right-shift operator
What are Bitwise Operators?
- Bitwise operators are used to perform bitwise operations on integers.
- Integer values are first converted to binary (0s and 1s) before performing bitwise operations.
- This operator operates on operands bit by bit hence the name Bitwise.
- Bitwise operator returns the result in decimal format.
Different Bitwise operators in Python
- There are 6 bitwise operators in Python
- Bitwise and operator (&)
- Bitwise or operator (|)
- Bitwise xor operator (^)
- Bitwise not operator (~)
- Bitwise left-shift operator (<<)
- Bitwise right-shift operator (>>)
Bitwise and operator in python '&' :
- Bitwise and operator is denoted by the ampersand sign
&
in Python. - It performs logical AND operation on individual bits of the integer operands.
- It converts an integer to equivalent binary value.
- Then it performs the AND operation on corresponding bits of the operands.
- Return value of bitwise and :
- it returns
True
if both the bits areTrue
. - it returns
False
if any one of the bit isFalse
.
- it returns
- Return value of bitwise and in terms of binary :
- it returns
1
if both the bits are1
. - it returns
0
if any one of the bits is0
.
- it returns
- It returns the final result as a decimal value.
- Example :
- Let us perform bitwise and
&
of two operands10
and22
. - First 10 is converted to binary i.e.
01010
. - Then 22 is converted to binary i.e.
10110
. and
operation is performed on corresponding bits of the two operands.- 0 & 1 = 0
- 1 & 0 = 0
- 0 & 1 = 0
- 1 & 1 = 1
- 0 & 0 = 0
- Hence result =
00010
- The final result is converted back to decimal, therefore we get 2 as answer.
- Let us perform bitwise and
- Code Sample :
x = 10
y = 22
res = x & y
print(res)
# Output
# 2
Bitwise or operator in python '|' :
- Bitwise or operator is denoted by the pipe symbol
|
in Python. - It performs logical OR operation on individual bits of the integer operands.
- It converts an integer to equivalent binary value.
- Then it performs the OR operation on corresponding bits of the operands.
- Return value of bitwise or :
- it returns
False
if both the bits areFalse
. - it returns
True
if any one of the bit isTrue
.
- it returns
- Return value of bitwise or in terms of binary :
- it returns
0
if both the bits are0
. - it returns
1
if any one of the bits is1
.
- it returns
- It returns the final result as a decimal value.
- Example :
- Let us perform bitwise or
|
of two operands10
and22
. - First 10 is converted to binary i.e.
01010
. - Then 22 is converted to binary i.e.
10110
. or
operation is performed on corresponding bits of the two operands.- 0 | 1 = 1
- 1 | 0 = 1
- 0 | 1 = 1
- 1 | 1 = 1
- 0 | 0 = 0
- Hence result =
11110
- The final result is converted back to decimal, therefore we get 30 as answer.
- Let us perform bitwise or
- Code Sample :
x = 10
y = 22
res = x | y
print(res)
# Output
# 30
Bitwise xor operator in python '^' :
- Bitwise xor operator is denoted by the caret symbol
^
in Python. - It performs logical XOR operation on individual bits of the integer operands.
- The word XOR stands for exclusive or.
- XOR operation means that -
True
is returned only if one operand isFalse
and one operand isTrue
.
- It converts an integer to equivalent binary value.
- Then it performs the XOR operation on corresponding bits of the operands.
- Return value of bitwise xor :
- it returns
False
if both the bits areFalse
. - it returns
False
if both the bits areTrue
. - it returns
True
if any one of the bit isTrue
and one bit isFalse
.
- it returns
- Return value of bitwise or in terms of binary :
- it returns
0
if both the bits are0
. - it returns
0
if both the bits are1
. - it returns
1
if any one of the bits is1
and one bit is0
- it returns
- It returns the final result as a decimal value.
- Example :
- Let us perform bitwise xor
^
of two operands10
and22
. - First 10 is converted to binary i.e.
01010
. - Then 22 is converted to binary i.e.
10110
. xor
operation is performed on corresponding bits of the two operands.- 0 ^ 1 = 1
- 1 ^ 0 = 1
- 0 ^ 1 = 1
- 1 ^ 1 = 0
- 0 ^ 0 = 0
- Hence result =
11100
- The final result is converted back to decimal, therefore we get 28 as answer.
- Let us perform bitwise xor
- Code Sample :
x = 10
y = 22
res = x ^ y
print(res)
# Output
# 28
Bitwise not operator in python '~' :
-
Bitwise not operator is denoted by the tilde symbol
~
in Python. - Bitwise not operator takes only one operand.
- It converts the integer operand to equivalent binary value.
- Then inverts each bit of the binary value.
- In other words it turns 0s to 1s and 1s to 0s.
- It returns the bitwise negation of the value as a result.
-
Example :
-
Let us perform bitwise not
~
of22
. -
First 22 is converted to binary i.e.
10110
. -
not
operation is performed on individual bits of the operand.- ~1 = 0
- ~0 = 1
- ~1 = 0
- ~1 = 0
- ~0 = 1
-
Hence result =
01001
- The final result is converted back to decimal.
-
Let us perform bitwise not
- In case of NOT operator we get a different result from expected result.
- Instead of the expected (9) 10 we get a negative number -23.
- This is because of the way python stores integers.
- Python stores integers as signed integers.
- Code Sample :
y = 22
res = ~y
print(res)
# Output
# -23
Bitwise left-shift operator in python '<<' :
- Bitwise left shift operator takes two operands.
- first it converts operand-1 to binary.
- Then it shifts the bits of operand-1 to the left by the number of places specified by operand-2.
- A gap is created at the right bits of operand-1 due to shifting.
- This gap is filled with zeros.
- It returns the decimal representation of the resulting binary number.
- Change in value of a number on shifting the bits to the left -
- Shifting the bits of any number to the left by one place doubles its value.
- Shifting the bits of any number to the left by two places multiplies its value by 2 2.
- Shifting the bits of any number to the left by three places multiplies its value by 2 3.
n << k = n * 2 k
- Example :
- Let us perform bitwise left shift
<<
with two operands5
and2
. - First 5 is converted to binary i.e.
101
. - bits of the binary number is shifted by
2
places as operand-2 is 2 - The gap created is filled with zeros.
- 101 << 2 = 10100
- Hence result =
10100
- The final result is converted back to decimal, therefore we get 20 as answer.
- Let us perform bitwise left shift
- Code Sample :
x = 5
y = 2
res = x << y
print(res)
# Output
# 20
Bitwise right-shift operator in python '>>' :
- Bitwise right shift operator takes two operands.
- first it converts operand-1 to binary.
- Then it shifts the bits of operand-1 to the right by the number of places specified by operand-2.
- A gap is created at the left bits of operand-1 due to shifting.
- This gap is filled with zeros.
- The rightmost bits are always dropped.
- It returns the decimal representation of the resulting binary number.
- Change in value of a number on shifting the bits to the right -
- Shifting the bits of any number to the right by one place halves its value.
- Shifting the bits of any number to the right by two places divides its value by 2 2.
- Shifting the bits of any number to the right by three places divides its value by 2 3.
n >> k = n / 2 k
- Example :
- Let us perform bitwise right shift
>>
with two operands50
and2
. - First 50 is converted to binary i.e.
110010
. - bits of the binary number is shifted by
2
places as operand-2 is 2 - The gap created is filled with zeros.
- 110010 >> 2 = 001100
- Hence result =
001100
- The final result is converted back to decimal, therefore we get 12 as answer.
- Let us perform bitwise right shift
- Code Sample :
x = 50
y = 2
res = x >> y
print(res)
# Output
# 12
Prev. Tutorial : Identity operators
Next Tutorial : Operator Precedence