Beginner Level
Intermediate Level
Advanced Level
Introduction
One of the most crucial features in Python that allows you to handle these errors is the 'try-except' block. In this Python tutorial, we will explore the try-except block, which is a fundamental tool that helps a programmer to handle errors and exceptional cases in their code execution. We will dive into the basics of try-except in Python programming language, understand its syntax, usage, and explore some of the real-world scenarios where it can be most helpful. So let's get started and understand how to use try-except block in Python programming.
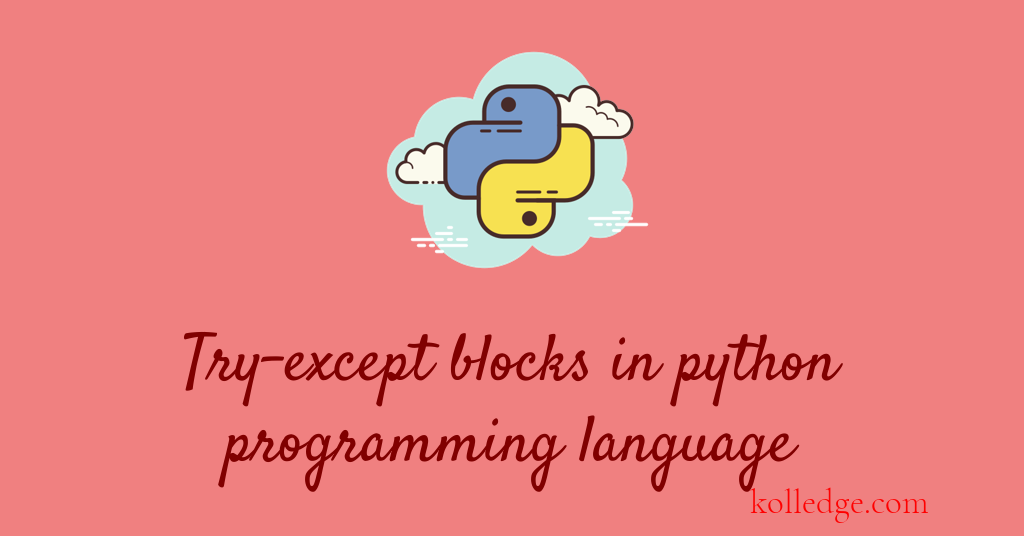
Table of Contents :
- try...except in Python
- Working of try...except block
- Code Sample for try…except block
- Catching specific exceptions
- Catching Multiple Exceptions
- Grouping Exceptions in Python
try...except in Python :
- A try...except block is used to implement exception handling in Python.
- The basic syntax of a try...except block in python is as follows :
try:
# Exception prone code
except:
# Code for handling exception
Working of try...except block
- The code that we think can cause an exception is wrapped within a try block.
- If an exception does not occur then all the statements of the try block are executed normally and the except block is ignored.
- If an exception occurs while the statements in the try block are getting executed :
- the try block stops executing
- the rest of the statements of the try block are not executed.
- the control jumps directly to the except block
- The code in except block is executed, allowing us to handle the error.
- We can also use the else keyword to specify a block of code that should only be executed if no errors occur.
- We have discussed the try..except...else construct in detail in an upcoming tutorial.
Code Sample for try…except block
- If we add a try except construct to the code sample from our previous tutorial.
- We'll see that the execution of the program completes gracefully even when there is an exception in the code.
- In the code sample below we see that
- the statements before the exceptions are executed normally.
- After the exception occurs the remaining statements within the try block are ignored.
- After the exception occurs the control jumps directly to the except block.
- Code Sample :
def my_function(x, y):
print("Inside my_function")
try:
print("Inside try block")
z = (x * y)/ (x + y)
print("Value of z = ", z)
except Exception as e:
print()
print("Inside except block")
print("An exception has occurred")
print("Terminating the program execution gracefully.")
my_function(10, -10)
# Output
# Inside my_function
# Inside try block
# Inside except block
# An exception has occurred
# Terminating the program execution gracefully.
Catching specific exceptions :
- There are many reasons that can cause exceptions.
- For example a code can have a
- valueError,
- ZeroDivisionError,
- TypeError,
- Keyerror etc.
- Python allows us to catch specific type of exception if we know what kind of exception that can occur in our code.
- There is also a provision of catching a generic exception if we are not sure what type of exception can occur.
- All exceptions are caught by this generic exception.
- Code Sample :
def my_function(x, y):
print("Inside my_function")
try:
print("Inside try block")
z = (x * y)/ (x + y)
print("Value of z = ", z)
except ZeroDivisionError as e:
print("Inside except block : ", e)
my_function(10, -10)
# Output
# Inside my_function
# Inside try block
# Inside except block : division by zero
Catching Multiple Exceptions :
- It is possible that multiple type of exceptions can occur in a single piece of code under different scenarios.
- Our code should be robust enough to handle all types of exceptions differently.
- Python allows us to handle multiple types of exceptions simultaneously.
- It is advised that we place a generic except block at the end.
- This generic except block will handle any exception that has not been handled separately in our code.
- Basic syntax of handling multiple exceptions is :
try:
# Vulnerable code
except ExceptionType1 as e1:
# Handle ExceptionType1
except ExceptionType2 as e2:
# Handle ExceptionType2
except ExceptionType3 as e3:
# Handle ExceptionType3
except Exception as e:
# Handle Generic Exception
- Code Sample :
def my_function(x, y, string):
print("Inside my_function")
try:
print("Inside try block")
z = (x * y) / (x + y)
print("Value of z = ", z)
index = string[10]
print("Character at index 10 : ", index)
except TypeError as te:
print("Inside TypeError except block : ")
print(te)
except ZeroDivisionError as ze:
print("Inside ZeroDivisionError except block : ")
print(ze)
except Exception as e :
print("Inside generic Exception except block : ")
print(e)
my_function("10", -10, "Hello")
print()
my_function(5,-5, "Hello")
print()
my_function(4, 1, "Hello")
# Output
# Inside my_function
# Inside try block
# Inside TypeError except block :
# can only concatenate str (not "int") to str
# Inside my_function
# Inside try block
# Inside ZeroDivisionError except block :
# division by zero
# Inside my_function
# Inside try block
# Value of z = 0.8
# Inside generic Exception except block :
# string index out of range
Grouping Exceptions in Python :
- We can group different exceptions if we want same response to multiple exceptions.
- The syntax of grouping exceptions is as follows :
try:
# Vulnerable code
except (Exception1, Exception2):
# handle exception1 an exception2
- Code Sample :
def my_function(x, y):
print("Inside my_function")
try:
print("Inside try block")
z = (x * y) / (x + y)
print("Value of z = ", z)
except (TypeError, ZeroDivisionError) as e:
print("Inside Group except block : ")
print(e)
print("Please check the input data")
except Exception as e:
print("Inside generic Exception except block : ")
print(e)
my_function("10", -10)
print()
my_function(5, -5)
# Output
# Inside my_function
# Inside try block
# Inside Group except block :
# can only concatenate str (not "int") to str
# Please check the input data
# Inside my_function
# Inside try block
# Inside Group except block :
# division by zero
# Please check the input data
Prev. Tutorial : What is exception handling
Next Tutorial : Finally block