Introduction
In the world of programming, variables are one of the most fundamental concepts that must be understood by any aspiring developer. Variables in Python can either be global or local, and it is crucial to know the difference between the two. In this tutorial, we will explore the topic of global and local variables in Python programming language. We will look at what each type of variable is, how they differ, and when to use them. This tutorial is intended for beginners who are just getting started with Python and want to gain a better understanding of how variables work in this powerful language.
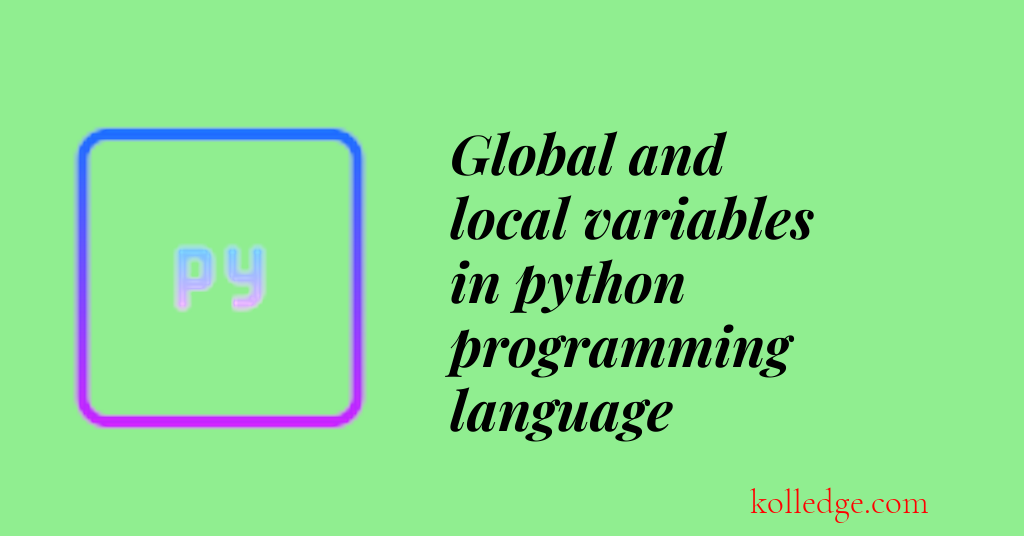
Table of Contents :
- Variable Scope
- Global Variables in Python
- Creating global variables in Python
- Local Variables in Python
- Creating local variables in Python
- Using local variables outside a function
- Local Variables Vs. Global Variables
Variable Scope :
- In the tutorial on Namespaces in Python we have read about variable scope.
- Here is a quick reminder of variable scope before we delve into the main topic.
- The scope of a variable is the lifespan of that variable.
- Variable scope is that section of code in which the variable is meaningful.
- Depending on their scope, variables are divided into :
- Global variables
- Local variables
Global Variables in Python :
- Global variables in Python are those variables which are defined outside any function.
- Global variables have a global scope.
- Global variables can be accessed from all sections of the program.
- Global variables are accessible from all the functions of the code.
Creating global variables in Python
- Lets take a look at how to define and access global variables in python.
def my_func():
print("Accessing global variable from inside : ", glb)
# This is the Global space
glb = "I am global."
print("Accessing global variable from outside : ", glb)
my_func()
# Output
# Accessing global variable from outside : I am global.
# Accessing global variable from inside : I am global.
Local Variables in Python
- Local variables in Python are those variables which are defined within a function.
- Local variables belong only to the function in which it was defined.
- The scope of a local variable is limited to the function in which it is defined.
- Local variables can be accessed inside that function only in which it was defined.
- Local variables are not accessible from anywhere outside that function.
Creating local variables in Python
- Below is the code to show how to define and access local variables in python.
def my_func():
lcl = "I am local."
print("Accessing local variable from inside : ", lcl)
# This is the Global space
my_func()
# Output
# Accessing local variable from inside : I am local.
Using local variables outside a function
- We know that local variable cannot be accessed outside the function in which they were defined.
- Let's try to access local variables from outside the function.
- Note # : When we try to access local variable from outside
- We get a
NameError
because the namelcl
is not known in global space.
def my_func():
lcl = "I am local."
print("Accessing local variable from inside : ", lcl)
# This is the Global space
my_func()
print("Accessing local variable from outside : ", lcl)
# Output
# Accessing local variable from inside : I am local.
# NameError: name 'lcl' is not defined
Local Variables Vs. Global Variables :
- Below is a tabular summary of differences between local and global variables in Python.
Basis | Python Global Variables | Python Local Variables |
Definition | Global variable are declared outside any function. | Local variables are declared within the functions |
Life span | created when the execution of the whole program begins. destroyed when the program execution ends. | created when execution of the function begins. destroyed when execution of the function ends. |
Variable Scope | Accessible from all parts of the code | Accessible only from within the function in which they are defined. |
Value | A change in the value is reflected throughout the code. | A change in the value can be reflected only within the function. |
Parameter Passing | parameter passing is not necessary in case of global variables | parameter passing is necessary in case of local variables |
Storage | Usually stored in the heap section of the memory. | Usually stored in stack section of the memory |
Data Sharing | Global variables support data Sharing | Local variables do not support data Sharing |
Prev. Tutorial : Default parameters
Next Tutorial : global keyword in Python