Introduction
One of the fundamental data types in Python is integers. Understanding how data types are stored in Python is crucial for efficient programming and avoiding common errors. In this tutorial, we will dive deep into the concepts of integer representation and storage in Python, providing you with a comprehensive understanding of how integers are stored, and how to work with them effectively. By the end of this tutorial, you will have a solid grasp on the intricacies of integer storage in Python, allowing you to write efficient and error-free code.
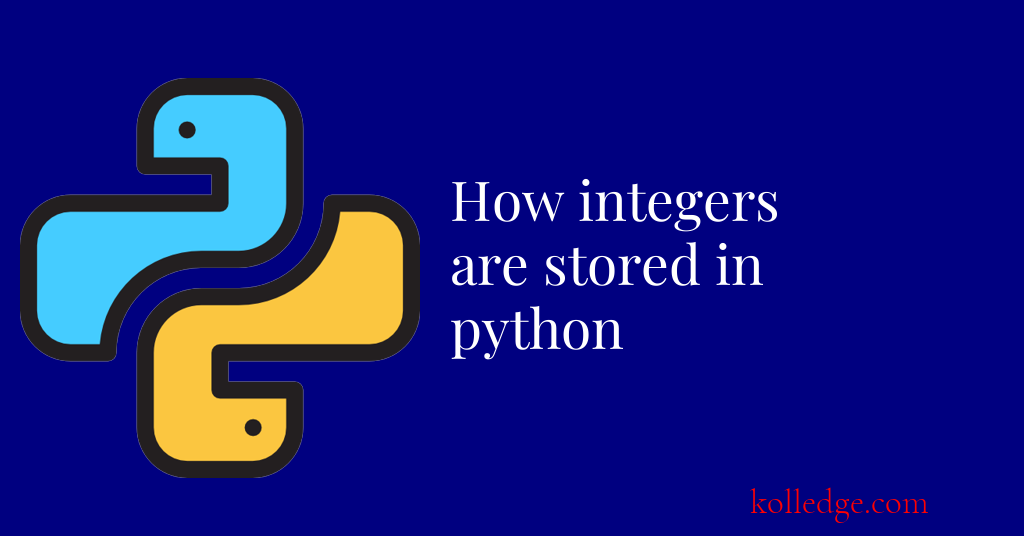
Table of Contents :
- What are Integers
- How Integers are stored in computers
- Storing unsigned Integers
- Storing Signed Integers
- Storing Signed Integers using MSB
- Storing Signed Integers using One's complement
- Storing Signed Integers using Two's complement
- How Integers are represented in Python
- Getting the type of an Integer variable in Python
- Getting the size of an Integer in Python
What are Integers
We have already read in our tutorial on integer Data type in Python that :
- Integers are whole numbers like : 14, 10, 100, 200, 0, 4, 6, 30
- Python implements the Integer data type using an int class.
- All integers are objects of this int class in Python.
How Integers are stored in computers :
In this section we'll see how computers store integers in the memory
- We know that computers can store data only in form of 0s and 1s.
- Same is true for integers as well.
- Computers save integers in the form of 0s and 1s.
- Examples :
- Computers store the Integer 6 as 110
- Computers store the Integer 9 as 1001
Storing unsigned Integers
- We can see that integer 6 is stored using 3 bits
- Integer 9 is stored using 4 bits.
- If we have 8 bits available we can store 256 unsigned integers i.e.
0 to 255
- If we have 16 bits available we can store 65536 unsigned integers i.e.
0 to 65535
- If we have
k
bits available we can store2k
unsigned integers i.e.0 to (2k) - 1
Storing Signed Integers
- An integer can be positive or negative.
- One bit of the binary number is used to represent the sign of the integer.
- This bit is called the Most significant bit (MSB)
- If the integer is positive, MSB has a value of 0,
- If the number is negative, MSB is 1.
- The entire binary representation of the integer is called a signed binary number.
Storing Signed Integers using MSB
- Suppose we want to represent integers 30 and 30 in binary using MSB method
- The first bit is used to represent the sign of the number.
- One shortcoming of this method is that the range of the positive integers that can be represented is reduced.
- This happens because
- now we have only
n - 1
bits to represent the value of the integer. - one bit is used to represent the sign of the integer
- now we have only
- The value range is represented by the formula :
( 2(n-1) - 1 ) to ( 2(n-1) - 1 )
- Another shortcoming of this method is that it generates a positive zero and a negative zero i.e. +0 and 0.
- This obviously does not exists.
Storing Signed Integers using One's complement
- In this method negative integers are represented by inverting all the bits of a positive integer.
- The sign bit of positive binary number is 0.
- When inversion of bits is done this sign bit is also inverted to 1 giving us an negative binary number.
- This method can represent one more integer than the MSB method.
- The value range is represented by the formula :
( 2(n-1) ) to ( 2(n-1) - 1 )
- But this method also generates negative zero
Storing Signed Integers using Two's complement
- In this method +1 is added to the 1's complement to represent a negative number.
- The sign bit indicates whether the number is negative or positive.
- The value range of integers obtained from this method is the same as the obtained from one’s complement method
( 2(n-1) ) to ( 2(n-1) - 1 )
- With this method negative 0 is not obtained.
- Apart from this another advantage of this method is that subtraction (or addition) of negative numbers can be done just by pretending the numbers as unsigned and adding (or subtract) them.
How Integers are represented in Python
- Unlike some other programming languages Python doesn’t use a fixed number of bits to store integers.
- Programming languages like Java and C# use a fixed number of bits to store integers.
- The range of integers that can be represented in these programming languages is fixed.
- In python this range is also not fixed.
- The largest integer number that can be represented in Python depends on the available memory.
- Since in python integers are also objects, some extra bytes are used to represent the object notation of integers.
Getting the type of an Integer variable in Python
- In the example given below we first define an integer variable
- Then we use the
type()
function to check the type of the variable. - The output clearly shows that an integer is an instance of the int class.
index = 100
print(type(index))
# Output
# <class 'int'>
Getting the size of an Integer in Python
- We can use the
getsizeof()
function to get the size of an integer. getsizeof()
function is defined in thesys
module.- The number of bytes that python uses to represent the variable are returned by the
getsizeof()
function. - Code Sample :
from sys import getsizeof
num_1 = 0
num_2 = 200
num_3 = 2 ** 32
size_num_1 = getsizeof(num_1)
size_num_2 = getsizeof(num_2)
size_num_3 = getsizeof(num_3)
print("Size of num_1 : ", size_num_1)
print("Size of num_2 : ", size_num_2)
print("Size of num_3 : ", size_num_3)
# Output
# Size of num_1 : 24
# Size of num_2 : 28
# Size of num_3 : 32
Prev. Tutorial : None Value
Next Tutorial : Storing float