Beginner Level
Intermediate Level
Advanced Level
Introduction
The continue statement is a powerful tool in Python that allows you to skip over certain items or iterations in a loop. This means that you can optimize your code and increase its efficiency by ignoring certain conditions or values that are not important. In this tutorial, we'll cover what the continue statement is, how it works, and how to use it effectively in your code. Whether you're a beginner or an experienced programmer, understanding the continue statement in Python is an essential skill to have in your toolbox. So, let's dive in and explore it together!
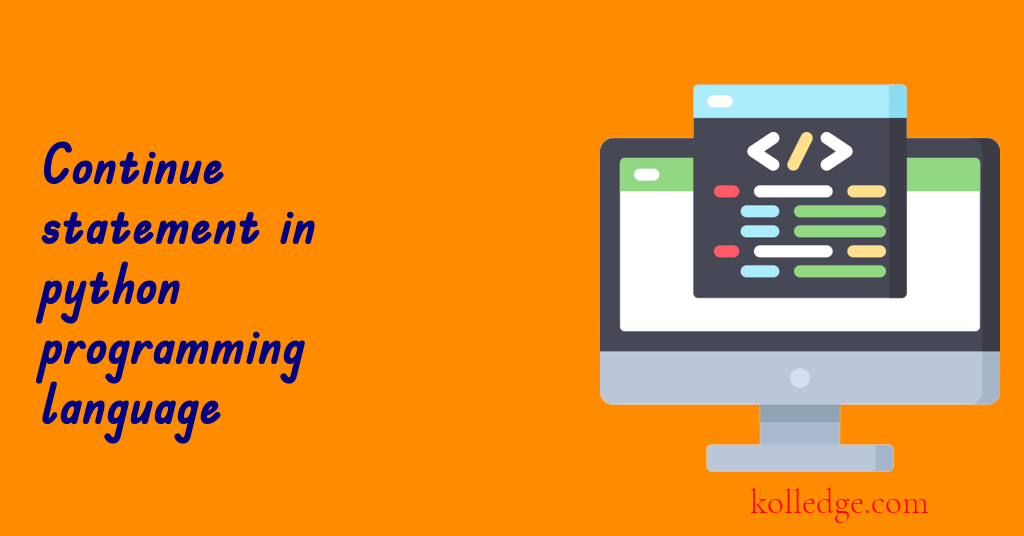
Table of Contents :
- Python Continue statement
- Using continue statement with for loop
- Using continue statement with while loop
- Using continue statement with nested loops
Python Continue statement
- The continue statement is be used inside a loop - a for loop or while loop.
- The main purpose of the continue statement is to skip the current iteration and directly start the next iteration.
- The statements after the continue statement are skipped for the current iteration and next iteration starts directly.
- Usually the continue statement is used with an if statement as we would like to skip an iteration only if some condition is met.
Using continue statement with for loop
- The syntax of the continue statement with for loop is as follows :
for cnt in range(n):
inner-code-block
if Continue-condition:
continue
outer-code-block
- Code Sample : Using continue statement with for loop
for index in range(5):
if index % 2 == 1:
print()
print(f"skipping index value {index}")
index += 1
continue
sqr = index ** 2
print()
print(f"Value of index = {index}")
print(f"Square of index = {sqr}")
print()
print("Coming out of for loop")
print(f"Value of index = {index}")
# Output
# Value of index = 0
# Square of index = 0
# skipping index value 1
# Value of index = 2
# Square of index = 4
# skipping index value 3
# Value of index = 4
# Square of index = 16
# Coming out of for loop
# Value of index = 4
Using continue statement with while loop
- The syntax of the continue statement with while loop is as follows :
while condition:
inner-code-block
if Continue-condition:
continue
outer-code-block
- Code Sample : Using continue statement with while loop
index = 0
while index < 5:
if index % 2 == 1:
print()
print(f"skipping index value {index}")
index += 1
continue
sqr = index ** 2
print()
print(f"Value of index = {index}")
print(f"Square of index = {sqr}")
index += 1
print()
print("Coming out of while loop")
print(f"Value of index = {index}")
# Output
# Value of index = 0
# Square of index = 0
# skipping index value 1
# Value of index = 2
# Square of index = 4
# skipping index value 3
# Value of index = 4
# Square of index = 16
# Coming out of while loop
# Value of index = 5
Using continue statement with nested loops
- In case of nested loops, the continue statement skips the current iteration of the inner loop.
- The syntax of the continue statement with nested loop is as follows :
for cnt in range(n):
for index in range(x):
inner-code-block
if Continue-condition:
continue
outer-code-block
- Code Sample : Using continue statement in nested loops
for index in range(2):
print("-------------------------------------")
print("Outer Loop")
print(f"Value of index = {index}")
print("Inner Loop")
for cnt in range(4):
print(f"Value of cnt = {cnt}")
if cnt % 3 == 1:
print("continue condition True.")
continue
print()
print("Coming out of outer for loop")
print(f"Value of index = {index}")
# Output
# -------------------------------------
# Outer Loop
# Value of index = 0
# Inner Loop
# Value of cnt = 0
# Value of cnt = 1
# continue condition True.
# Value of cnt = 2
# Value of cnt = 3
# -------------------------------------
# Outer Loop
# Value of index = 1
# Inner Loop
# Value of cnt = 0
# Value of cnt = 1
# continue condition True.
# Value of cnt = 2
# Value of cnt = 3
# Coming out of outer for loop
# Value of index = 1
Prev. Tutorial : Break statement
Next Tutorial : Pass statement