Introduction
If you are looking to work with databases in Python programming language, then it's crucial for you to understand the use of the SQL LIMIT clause. This clause limits the number of records retrieved from a table, which can help you improve the performance of your program by reducing the amount of data that needs to be processed. In this tutorial, we will learn about using the SQL LIMIT clause in Python code. We will also explore some real-world scenarios where using the LIMIT clause can be beneficial. By the end of this tutorial, you will have a good understanding of how to use this clause to optimize your database queries in Python.
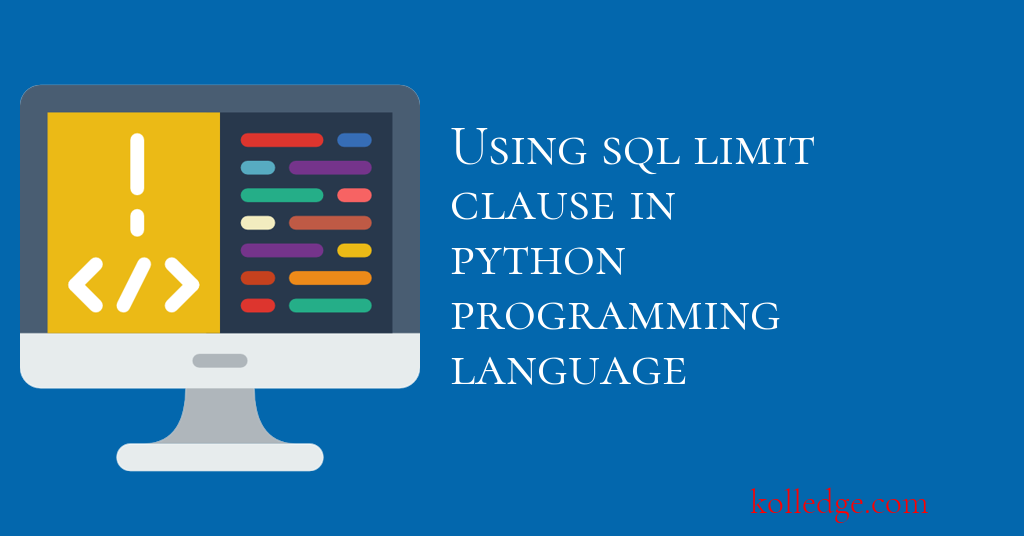
Table of Contents :
- What is SQL Limit clause
- SQL Limit clause with offset
- skip a certain number of rows before returning the results.
- paginate the results of a SQL query
- paginate the sorted results of a SQL query
- How to use limit clause in MySQL with Python
- Step 1: Install the required Packages
- Step 2: Establishing a Connection to the MySQL Server
- Step 3: Create a Cursor Object
- Step 4: use limit clause
What is SQL Limit clause :
- The SQL LIMIT clause is used to limit the number of rows returned in a SQL query.
- For example, if we want to retrieve only the first 10 rows from a table, we can use the LIMIT clause as :
SELECT * FROM table_name LIMIT 10;
SQL Limit clause with offset :
- We can also use the limit clause with the OFFSET keyword to
- skip a certain number of rows before returning the results.
- paginate the results of a SQL query
- paginate the sorted results of a SQL query
- Skip a certain number of rows :
- For example, if we want to retrieve the rows from 11 to 20 from a table, we can use the following query:
SELECT * FROM table_name LIMIT 10 OFFSET 10;
- paginate the results of a SQL query :
- We can also use the limit and offset clauses together to paginate the results of a SQL query.
- For example, if we want to retrieve the results of a query in pages of 10 rows each, we can use the following SQL query:
SELECT * FROM table_name LIMIT 10 OFFSET 10 * (page_number - 1);
- paginate the sorted results of a SQL query
- We can also use the limit and offset clauses together with the ORDER BY clause to paginate the sorted results of a SQL query.
- For example, if we want to retrieve the results of a query in pages of 10 rows each, sorted by the value of the id column in ascending order, we can use the following SQL query :
SELECT * FROM table_name ORDER BY id LIMIT 10 OFFSET 10 * (page_number - 1);
How to use limit clause in MySQL with Python :
- Follow the steps given below to use limit clause in MySQL with python
- Step 1: Install the required Packages
- Step 2: Establishing a Connection to the MySQL Server
- Step 3: Create a Cursor Object
- Step 4: use limit clause
Step 1: Install the Required Packages
- The first step is to ensure that you have installed the required packages on your machine.
- The most popular packages for connecting to MySQL in Python are
- mysql-connector and
- PyMySQL.
- You can install these packages using
pip
through the command line. - Code Sample :
!pip install mysql-connector-python
!pip install PyMySQL
Step 2: Connect to the MySQL Database
- To create a new database in MySQL using Python, we must have a connection to the MySQL server.
- To connect to a MySQL server in Python we can use either
- mysql.connector or
- PyMySQL package
- We need to provide the necessary connection parameters to establish the connection.
- We need to pass the following parameters to the Connection object:
- host : the hostname of the MySQL server user
- user : username for the MySQL account
- password : password for the MySQL account database
- database : The name of the database to connect to
- To study package specific code for connecting to MySQL read our tutorial on connecting to MySQL server.
Step 3: Create a Cursor Object
- After establishing a connection to the MySQL server, your next step is to create a cursor object.
- This is done using the
cursor()
method provided bymysql.connector
orPyMySQL
. - Example:
cursor = mydb.cursor()
Step 4: use limit clause
- To limit the number of rows returned by a
SELECT
statement in a MySQL table using Python, use theLIMIT
clause. - Use the
execute()
method on the cursor object to execute the query. - Code Sample :
query = "SELECT * FROM employees LIMIT 5"
cursor.execute(query)
result = cursor.fetchall()
for row in result:
print(row)
Prev. Tutorial : Update table in mysql
Next Tutorial : Using sql join clause