Beginner Level
Intermediate Level
Advanced Level
Introduction
In programming, working with files is a common task, and Python provides us with an easy and efficient way to handle files. Creating a file allows us to store data, text, or other information permanently to be accessed later. In this tutorial, you will learn how to create a file in Python. We will cover the basics of file handling, which is an important concept in Python programming, and we will provide practical examples to help you understand the process better. So, let's get started!
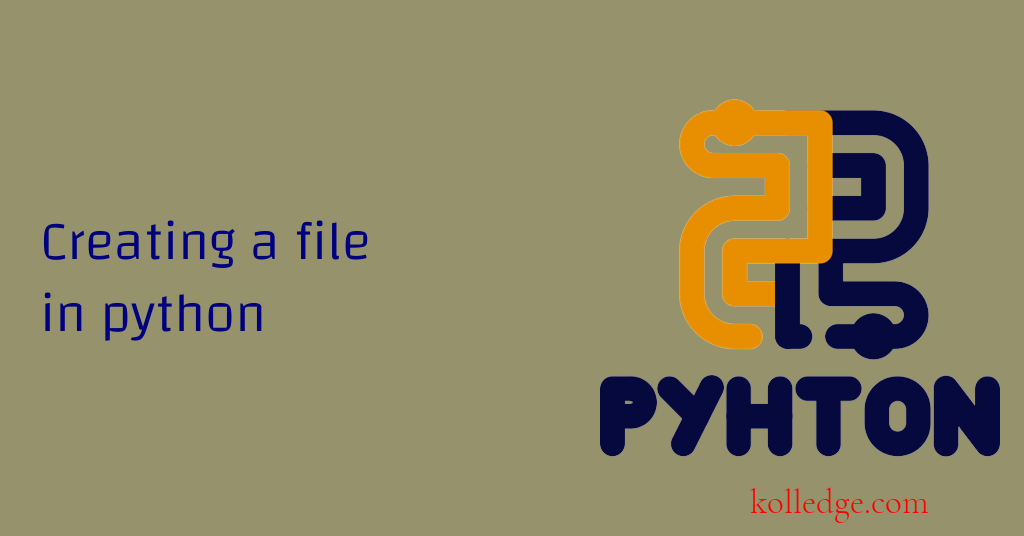
Table of Contents :
- How to create a text file in python
- List of access modes for creating a file
- Different scenarios of creating file in python
- create a file in a specific directory
- create a file if it does not exists
- create a new file using with statement
- create file with a datetime
- create a file with permission
How to create a text file in python
- We can create a file in python by using the open() function.
- We pass the filename as an argument in
open()
function. - The second argument of the open function is one of access modes.
- A list of possible access modes for creating a file is given in the next section.
- If the file does not already exist, it will be created.
- Code Sample :
file = open("example.txt", "w")
file.close()
List of access modes for creating a file
Mode | Description |
---|---|
w | Write mode, truncates the file if it exists or creates a new file if it does not exist |
x | Exclusive creation mode. If the file already exists, the open() method call will fail |
a | Append mode, opens the file in append mode. If the file does not exist, a new file will be created |
t | Text mode |
b | Binary mode |
Different scenarios of creating file in python
- There are several scenarios in which we might have to create a file in python.
- In this section we'll discuss some of these scenarios :
- create a file in a specific directory
- create a file if it does not exists
- create file with a datetime
- create a file with permission
How to create a file in a specific directory
- We can create a file in a particular directory by getting the full path to the file.
- This full path is passed as an argument to the
open()
function. - We can get the full path to the file by joining the filename at the end of full path to the directory.
- We can join the filename to the directory path using the
os
module of python. - Code Sample :
import os
directory = r'C:\Users\MyUser\Documents'
filename = 'example.txt'
filepath = os.path.join(directory, filename)
file = open(filepath, "w")
file.close()
How to create a file if it does not exists
- We can check if a file exists or not before creating the file.
- If the file does not exists we create a new file.
- We can check if the file already exists by using the
os
module. - the
exists()
function can be used to check if the file exists or not. - The syntax of checking if file exists is :
os.path.exists(filepath)
- Code Sample :
import os
filepath = 'example.txt'
if not os.path.exists(filepath):
file = open(filepath, 'w')
file.close()
How to create a new file using with statement
- The
with
statement can be used to create a new file and write data to it. - Code Sample :
with open("newfile.txt", "w") as file:
file.write("This is a new file.")
How to create file with a datetime
- We can use f-strings to insert the datetime within the filename.
- Once the current date and time is inserted within the filename, we can create the file as we do normally.
- Code Sample :
import datetime
current_time = datetime.datetime.now()
formatted_time = current_time.strftime("%Y-%m-%d_%H-%M-%S")
filename = f"example_{formatted_time}.txt"
file = open(filename, 'w')
file.close()
How to create a file with permission
- We can set file permissions using the numeric form of the
chmod()
method. - Read, Write, Execute permissions are represented as `r`, `w`, and `x` respectively.
- We can use the
mknod()
function ofos
module to create a file system node. - File system node can be a file, a device special file or a named pipe.
- Code Sample :
import os
filename = "example.txt"
# read and write permission for owner, group, and others
permission = 0o666
os.mknod(filename, mode=permission)
Prev. Tutorial : Intro to file handling
Next Tutorial : Opening a file