Introduction
In programming Arrays are used to store a collection of elements of the same type in a contiguous memory location. They offer a convenient and efficient way to manipulate large amounts of data, making them an essential tool for any programmer. In this tutorial, we will explore how we can implement arrays in Python, including how to create and manipulate arrays, perform various operations on them, and more. So, whether you are a beginner in Python or an experienced programmer, this tutorial will help you master the concept of arrays in Python programming language.
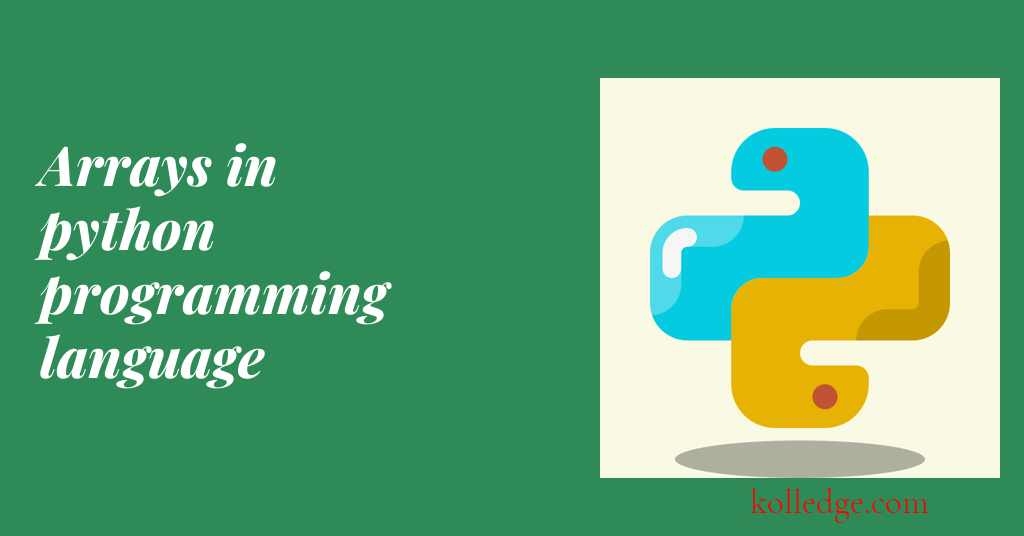
Table of Contents :
- What are Arrays in Programming
- Arrays in Python
- Creating arrays in Python
- Different Array Operations in Python
- Accessing elements of array
- Change the value of an element
- Add elements to array
- Remove an element from array
- Find the length of array
What are Arrays in Programming
- An array is a data structure that stores a collection of elements.
- Each element in the array has a specific index that can be used to access it.
- Arrays are often used in programming to store data such as lists of numbers or strings.
- We can access the elements of an array using its index.
Arrays in Python
- In Python we do not have a built-in support for Arrays.
- We can implement Array functionality in Python using the built-in List data type
- Difference between Arrays and List -
- Lists can store elements of various data types at a time whereas Arrays can store elements of a single data type at a time.
- Lists cannot directly handle arithmetic operations whereas Arrays can directly handle arithmetic operations.
Creating arrays in Python
- To create an array in python, we beed to use the
array
module. - The
array
module consists of anarray()
function that can be used to create arrays in python - array function takes a list of values and returns an array object
- Syntax of array function :
array_name = array(typecode, [list_elements])
- array_name is the name of the array that we'll get
- typecode specifies the type of the elements that will be stored in the array e.g. int, float etc.
- [list_elements] is the list that will be converted to array.
- typecodes for array function : Below is the list of typecodes that we can use in the array function.
Typecode | C type | Python Type | Size |
---|---|---|---|
'b' | signed char | int | 1 |
'B' | unsigned char | int | 1 |
'u' | wchar_t | Unicode character | 2 |
'h' | signed short | int | 2 |
'H' | unsigned short | int | 2 |
'i' | signed int | int | 2 |
'I' | unsigned int | int | 2 |
'l' | signed long | int | 4 |
'L' | unsigned long | int | 4 |
'q' | signed long long | int | 8 |
'Q' | unsigned long long | int | 8 |
'f' | float | float | 4 |
'd' | double | float | 8 |
- Example of creating array in Python
from array import *
my_list = [1, 2, 3, 4]
my_array = array('i',my_list)
my_array2 = array('i',[5, 6, 7, 8])
print(my_array)
print(my_array2)
# Output
# array('i', [1, 2, 3, 4])
# array('i', [5, 6, 7, 8])
Different Array Operations in Python
Different array operations possible in Python are -
- Accessing elements of array
- Change the value of an element
- Add elements to array
- Remove an element from array
- Find the length of array
Accessing elements of array
- We can access the elements of an array using their index.
- The first element has an index of 0, the second element has an index of 1, and so on.
- We can also use negative indexes to access elements from the end of the array.
from array import *
my_list = [1, 2, 3, 4]
my_array = array('i',my_list)
print(my_array[0]) # prints 1
print(my_array[1]) # prints 2
print(my_array[2]) # prints 3
# Output
# 1
# 2
# 3
from array import *
my_list = [1, 2, 3, 4]
my_array = array('i', my_list)
print(my_array[-1]) # prints 4
print(my_array[-2]) # prints 3
Change the value of an element
- To change the value of an element in an array, we can use its index.
from array import *
my_list = [1, 2, 3, 4]
my_array = array('i', my_list)
my_array[0] = 10
print(my_array)
# Output
# array('i', [10, 2, 3, 4])
Add elements to array
- We can also use the append() method to add elements to an array:
from array import *
my_list = [1, 2, 3, 4]
my_array = array('i', my_list)
my_array.append(5)
print(my_array)
# Output
# array('i', [1, 2, 3, 4, 5])
Remove an element from array
- The remove() method can be used to remove an element from an array.
- The pop() method can be used to remove an element from an array and return it.
from array import *
my_list = [1, 2, 3, 4]
my_array = array('i', my_list)
my_array.remove(3)
print(my_array)
# Output
# array('i', [1, 2, 4])
from array import *
my_list = [1, 2, 3, 4]
my_array = array('i', my_list)
my_element = my_array.pop(0)
print(my_element)
print(my_array)
# Output
# 1
# array('i', [2, 3, 4])
Find the length of array
- We can use the len() function to get the length of an array
from array import *
my_list = [1, 2, 3, 4]
my_array = array('i', my_list)
l = len(my_array)
print(l)
# Output
# 4
Prev. Tutorial : Bool Data type
Next Tutorial : Type conversion in Python