Beginner Level
Intermediate Level
Advanced Level
Introduction
Python modules and packages are essential concepts for understanding how to structure and organize your Python code. Though often used interchangeably, modules and packages are actually distinct entities with different purposes. In this tutorial, we'll explore the difference between modules and packages in Python. Whether you're a beginner or an experienced Python developer, mastering these concepts will help you write cleaner, more efficient, and more scalable code. So, let's dive in and explore the world of modules and packages in Python!
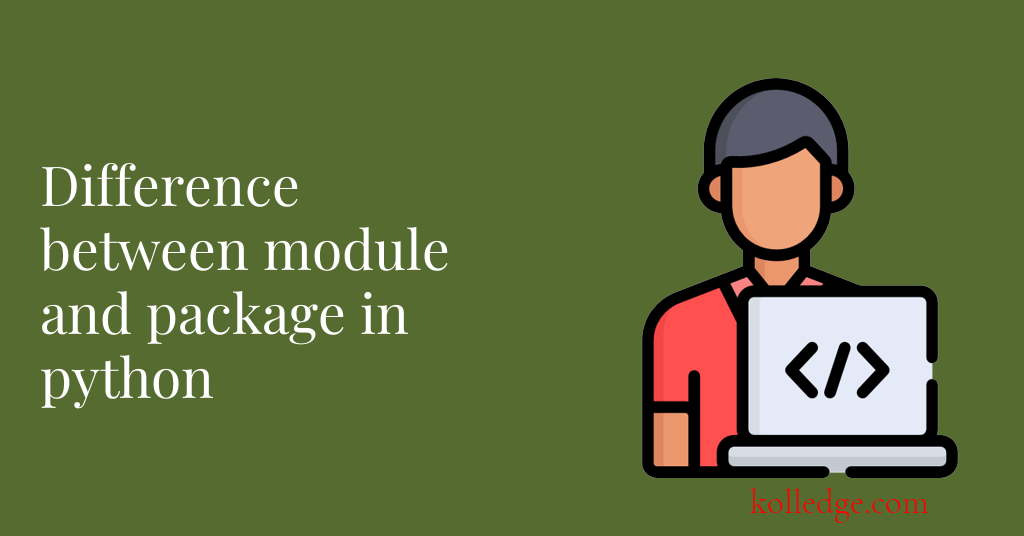
Table of Contents :
- Modules in Python
- Packages in Python
- Difference between Modules and Packages in Python
Modules in Python :
- A module is a single file that contains Python definitions, functions, and statements.
- It is used to break up large programs into smaller, more manageable parts.
- Modules are imported using the
import
statement followed by the module name. - Example:
# module_example.py
def greeting(name):
print("Hello, " + name)
def farewell(name):
print("Goodbye, " + name)
# main.py
import module_example
module_example.greeting("John")
module_example.farewell("John")
Packages in Python :
- A package is a way to organize related modules into a single parent directory/folder.
- It can contain one or more sub-packages, which can also contain other sub-packages.
- Packages are created by adding a special file called
__init__.py
to a directory. - Example:
# my_package/__init__.py
from . import module1
from .sub_package import module2
__all__ = ["module1", "module2"]
# main.py
import my_package.module1
from my_package.sub_package import module2
my_package.module1.greeting("John")
module2.farewell("John")
Difference between Modules and Packages in Python :
- A module is a single file, while a package is a directory that can contain multiple modules.
- Modules are loaded into memory when they are imported, while packages need to be initialized by executing the
__init__.py
file. - Modules cannot have sub-modules, while packages can have one or more sub-packages.
- Modules are imported using the
import
statement, packages are also imported using theimport
statement, but the package name is followed by module name separated by a dot.
Prev. Tutorial : Creating packages
Next Tutorial : Datetime module