Introduction
Namespaces in Python programming language are a fundamental concept that every Python developer should be familiar with. A namespace is simply a system that allows Python variables and objects to be assigned names that can be used to uniquely identify them within a program. When working with complex Python applications, namespaces are crucial for avoiding naming conflicts and keeping code organized. This Python tutorial will explore namespaces in depth, providing practical examples and explanations that will help readers gain a solid understanding of how namespaces work in Python. Whether you are a beginner or an experienced developer, this tutorial is a valuable resource for mastering this crucial aspect of Python programming.
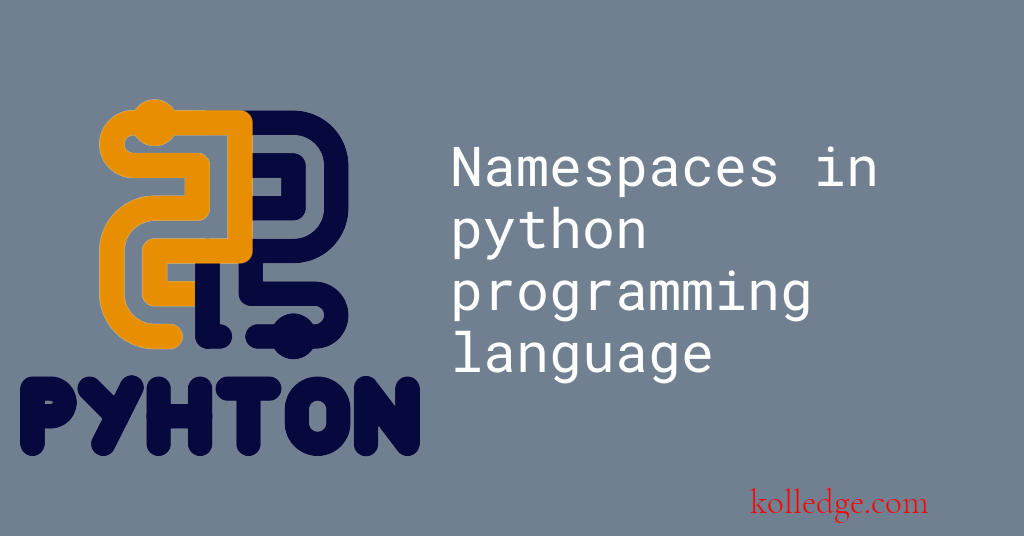
Table of Contents :
- What is Namespace in Python?
- How Namespaces Work in Python
- Types of Namespaces in Python
- Local
- Enclosing
- Global
- Built-in
- Variable Scope
What is Namespace in Python?
- In Python practically everything that we create or use is an object.
- Be it a literal, variable, function, module etc. everything in python is an object.
- We assign symbolic names to these objects so that we can refer to them in our code.
- For example an assignment statement creates a variable name that is used to refer to an object.
By the statementindex = 10
a variable name index is created and it refers to an integer object with value 10. - When we define a function
def my_function()
we create a symbolic name my_function.
- For example an assignment statement creates a variable name that is used to refer to an object.
- When we work on large projects we end up creating thousands of such symbolic names each of which point to some object.
- In a project with thousands of lines of code and several code files there is a high probability that many files have objects with same name.
- Namespace is the technique which enables python to discriminate between different objects even if they are referenced by same name.
- In other words namespace allows python to maintain a unique name for every object.
- Namespace is the collection of symbolic names currently defined.
- Namespace also contains information about the objects that are referred to by these names.
- In python namespaces can be thought of as dictionaries where
- keys are the symbolic names and
- values are the objects they refer to.
How Namespaces Work in Python
To better understand how namespaces work we take a practical example of computer directories
- Suppose we want to save a large number of files assorted from different sources in our computer.
- There is a high probability that there can be a lot of files with same name.
- If we try to keep all files in the same directory (or folder) then we'll get an error as many files will be having same name.
- But if we create different folders and arrange files in such a manner that files with same name are in different directories then we can easily save all the files.
- In the same manner python can keep objects with same names as long as they are in different namespaces.
Types of Namespaces in Python
- There are four types of namespaces in python
- Local
- Enclosing
- Global
- Built-in
- There are several built-in namespaces in Python and we can create our own namespaces as well.
- Python interpreter creates namespaces at runtime as and when necessary.
- When a namespace is no longer needed it is deleted.
Built-in Namespace in Python -
- This is the namespace where Python stores all the names of the built-in objects.
- These names are available at all times while python interpreter is running.
- This namespace is created when python interpreter starts up.
- Built-in namespace remains in existence till the python interpreter stops.
Global Namespace in Python -
- This is the namespace where Python stores the names of all the objects that are defined at the level of main program.
- This namespace is created when the main program body starts getting executed by the interpreter.
- Global namespace remains in existence till the python interpreter stops.
- There can be more than one global namespaces at a time.
- Python interpreter creates global namespace for any module that is loaded through import Statement.
Local Namespace in Python -
- This namespace is created when a function is executed.
- Local namespace stores the names of all the objects that are local to that function.
- Local namespace remains in existence till that function terminates.
Enclosing Namespace in Python -
- In Python we can define one function inside another function.
- The inner function is called the enclosed function.
- The outer function is called the enclosing function.
- The namespace for the inner function is local namespace.
- The namespace for outer function is enclosing namespace.
- These namespace exist till their respective functions are not terminated.
Variable Scope :
- We know that different objects can have same name as long as they are in different namespaces.
- But how does Python interpreter decide which exact object is referred to by a symbolic name if there are a lot of objects with that name.
- This is where variable scope comes into play.
- scope of a symbolic name is that region of the code in which this symbolic name is meaningful.
- The region where a particular name has meaning, is decided by the interpreter based on the location where the definition of this name occurs in the code.
- We have already seen that there are four types of namespaces. Suppose we refer to an object with name xyz in our code and the name xyz exists in several namespaces then the python interpreter searches for the name as per the LEGB rule
- Local: the interpreter first looks in the local namespace.
- Enclosing: if the identifier is not found in the local namespace then the interpreter searches in the enclosing namespace
- Global: If the identifier is still not found the interpreter looks in the global namespace.
- Built-in: If the identifier is not found in the global namespace either, the interpreter looks in the built-in namespace.
- If the identifier is not found in any of these namespaces, Python raises a NameError exception.
Prev. Tutorial : Statements and indentation
Next Tutorial : Comments