Beginner Level
Intermediate Level
Advanced Level
Introduction
In Python, the None value is a special data type that represents the absence of a value. It is often used to indicate that a variable or a function does not have a value or return anything. The None value plays an essential role in many Python programs, as it can be used to test for the existence of a variable or to perform some operations conditionally. In this tutorial, we will explore the definition and the uses of the None value in Python, as well as some practical examples of how to work with this data type effectively.
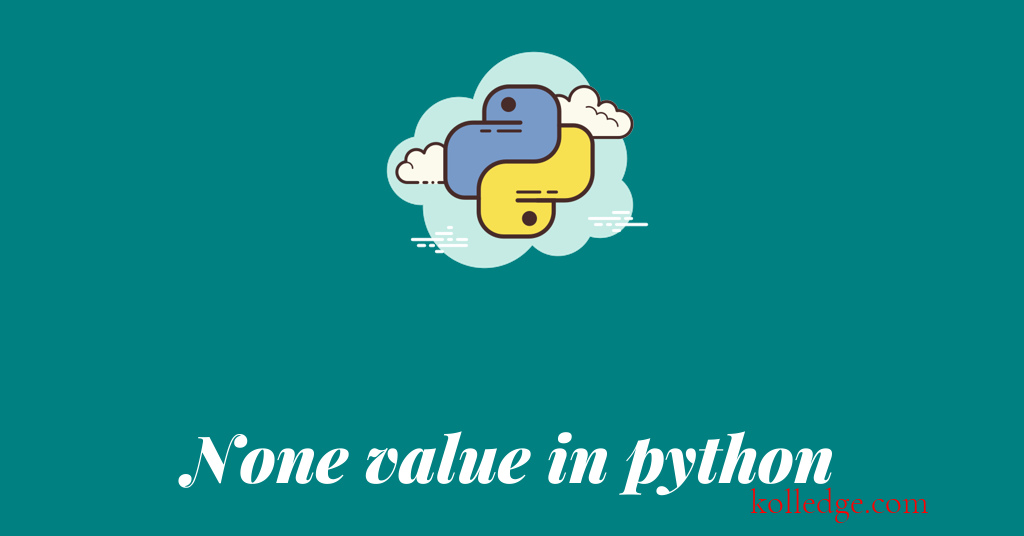
Table of Contents :
- Python None value
- Initializing variable with None value
- Mutable default argument issue
- Return value of a function
- Checking for the Python None value
Python None value :
- The Python
None
value is a built-in constant representing the absence of a value or an undefined value. - It is used to indicate the absence of a value where one is expected,
- such as the return value of a function that doesn't return anything.
Initializing variable with None value :
- We can initialize a variable with
None
value when you don't have a value to assign yet. - The syntax of initializing with none value is :
value = None
Mutable default argument issue :
- We can use the Python None Object to Fix the Mutable Default Argument Issue
- When using a mutable object as a default argument in a function, the default value is shared across all function instances.
- This leads to unexpected behavior.
- We can use
None
as a default argument and then assign a mutable object in the function body. - This ensures that each function instance gets a unique copy of the mutable object.
def add_item(item, lst=None):
if lst is None:
lst = []
lst.append(item)
return lst
Return value of a function :
- We can use the Python None Object as a Return Value of a Function.
None
is often returned from functions that perform an action or have a side effect, but don't return an actual value.- This is useful to avoid returning useless placeholder values, such as empty strings or empty lists.
def send_email(to, subject, body):
# Code to send email goes here...
return None
Checking for the Python None value :
None
is a singleton object and can be checked for using theis
operator instead of the==
operator.- This is because
is
checks if two objects are the same object in memory, - while
==
checks if two objects have the same value.
value = None
if value is None:
print("value is None")
else:
print("value is not None")
Prev. Tutorial : Mutable/Immutable Objects
Next Tutorial : Storing Integers