Beginner Level
Intermediate Level
Advanced Level
Introduction
Conditional statements are an important concept in programming as they allow for decision-making based on certain conditions being met. In Python, the If...elif...else statement is a powerful tool that enables developers to control the flow of their code based on a series of conditions. Through this tutorial, we will explore the syntax and usage of the If...elif...else statement in Python, along with some practical examples that demonstrate how it can be used to create efficient and effective code. Let's get started!
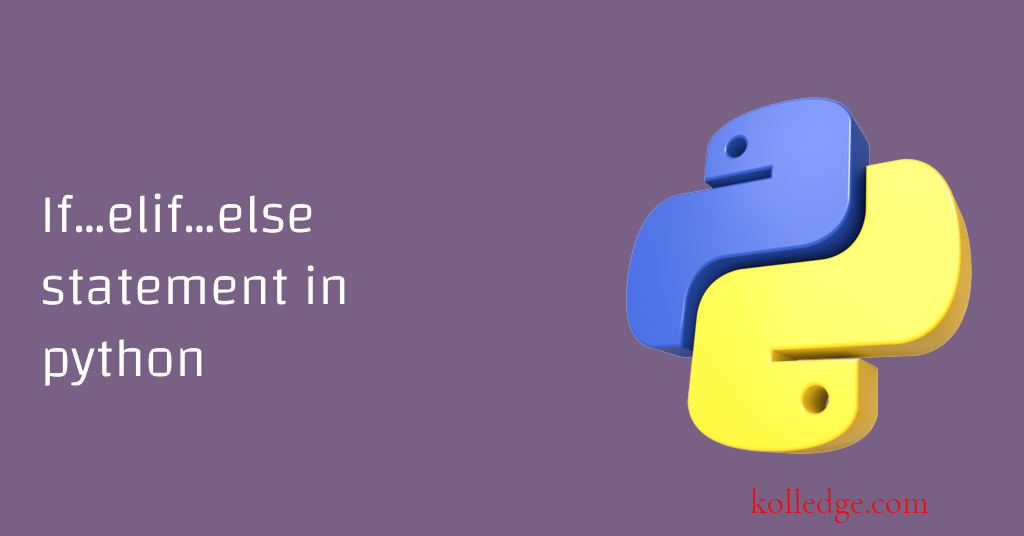
Table of Contents :
- Python if…elif…else statement
- Control flow for if...elif...else statement
Python if…elif…else statement
- Sometimes while writing a code need arises to check multiple conditions one after another.
- We might need to perform a task if certain condition is True and another task some other condition is True.
- This functionality is implemented using the if…elif…else statement.
- elif stands for else if
- Here is the syntax if the if...elif...else statement in Python:
if If-condition:
code-block-If
elif Elif-condition1:
code-block-Elif-1
elif Elif-condition2:
code-block-Elif-2
...
else:
code-block-Else
outer-code-block
Control flow for if...elif...else statement :
- The python interpreter evaluates the logical-conditions of if...elif...else statement one by one.
- The conditions are evaluated in the order that they appear in the statement - If-condition, Elif-condition1, Elif-condition2 and so on.
- This process goes on till the interpreter finds a condition that is True.
- When the interpreter finds a condition that is true, the code-block that follows this condition is executed.
- Once a True condition is found the remaining conditions are skipped and not evaluated. The control jumps directly to the outer block.
- If no condition evaluates to True, interpreter executes the code-block in the else branch.
- The else branch is not mandatory and can be omitted.
- If all the conditions evaluate to False and there is no else block, the whole if...elif statement does nothing and control jumps directly to the outer-code-block.
- The outer-code-block is always executed.
- Code Sample :
x = 15
if x > 25:
print("I am the code-block-If")
print("Logical-condition-if is True")
print()
elif x > 20:
print("I am the code-block-Elif-1")
print("Logical-condition-if is False")
print("Logical-condition-elif-1 is True")
print()
elif x > 10:
print("I am the code-block-Elif-2")
print("Logical-condition-if is False")
print("Logical-condition-elif-1 is False")
print("Logical-condition-elif-2 is True")
print()
else:
print("I am the code-block-Else")
print("Logical-condition-if is False")
print("Logical-condition-elif-1 is False")
print("Logical-condition-elif-2 is False")
print()
print("I am the outer-code-block.")
# Output
# I am the code-block-Elif-2
# Logical-condition-if is False
# Logical-condition-elif-1 is False
# Logical-condition-elif-2 is True
# I am the outer-code-block.
Prev. Tutorial : If..else Statement
Next Tutorial : Ternary Operator in Python