Beginner Level
Intermediate Level
Advanced Level
Introduction
Python provides basic string manipulation functions that can be used by programmers to perform a wide range of sub-string operations. Strings are one of the most commonly used data types in Python, and understanding how to extract sub-strings from them is a key aspect of working with strings. In this tutorial, we will explore various methods of extracting sub-strings from strings using Python.
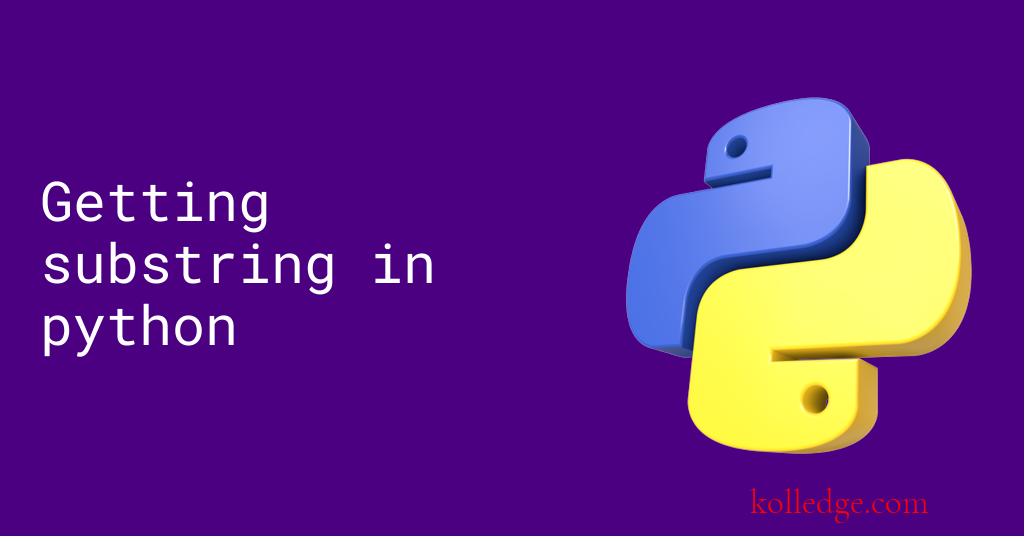
Table of Contents :
- Different ways of getting sub-string in Python :
- Basic sub-string slicing
- Negative index slicing
- Using String Methods
- Using the split() method
- Using Regular Expressions
- Concatenating Sub-strings
Different ways of getting sub-string in Python :
- In python we can get sub-strings from a string using different methods, these include :
- Basic sub-string slicing
- Negative index slicing
- Using String Methods
- Using the split() method
- Using Regular Expressions
Basic sub-string slicing :
- We can use the slicing technique to get a sub-string from a string in python.
- We can use square brackets
[ ]
and index numbers to access a sub-string of characters within a string. - Separate the start and end indices with a colon
:
to slice a part of the string.
- We can use square brackets
- Code Sample :
my_string = "Hello, Kolledge!"
substring = my_string[11:15]
print(substring)
# Output
# edge
Negative index slicing:
- We can use negative index numbers to access characters from the end of the string.
- Code Sample :
my_string = "Hello, Kolledge!"
substring = my_string[-9:-1]
print(substring)
# Output
# Kolledge
Using String Methods:
- We can use string methods such as find(), index(), and count() to find a sub-string within a string.
- the start index and length of the sub-string are used to slice the original string.
- Code Sample :
my_string = "Hello, Kolledge!"
start_index = my_string.find("Kolledge")
length = len("Kolledge")
substring = my_string[start_index:start_index+length]
print(substring)
# Output
# Kolledge
Split the Original String:
- We can use the
split()
method to separate the original string into an array of sub-strings. - we can then access the desired sub-string by its index.
- Code Sample :
my_string = "Hello, Kolledge!"
substrings = my_string.split(", ")
substring = substrings[1]
print(substring)
# Output
# Kolledge!
Using Regular Expressions:
- We can use the regular expressions to search for a pattern within a string.
- We can use the
re.search()
method ofre
module for this purpose. - Extract the matched sub-string using the
group()
method or by slicing the original string based on the match indices. - Code Sample :
import re
my_string = "Hello 123 Kolledge Scholars."
pattern = r"\d+ \w+ \w+\."
match = re.search(pattern, my_string)
substring = match.group()
print(substring)
# Output
# 123 Kolledge Scholars.
Concatenating Sub-strings:
- We can use the concatenation operator to concatenate multiple sub-strings together into a final string.
- Code Sample :
my_string = "Hello, Kolledge!"
substring1 = my_string[0:5]
substring2 = my_string[8:]
substring3 = "Python"
final_string = substring1 + " " + substring3
print(final_string)
# Output
# Hello Python
Prev. Tutorial : Splitting a string
Next Tutorial : Concatenate strings