Introduction
A class is a blueprint or a template for creating objects that share the same attributes and methods. Classes forms the backbone of object-oriented programming and are essential for writing complex and scalable applications. With the help of classes, we can organize our code better, make it more modular, and reduce code redundancy. In this tutorial, we will cover the basics of classes. So, let's dive in and learn about this essential programming concept!
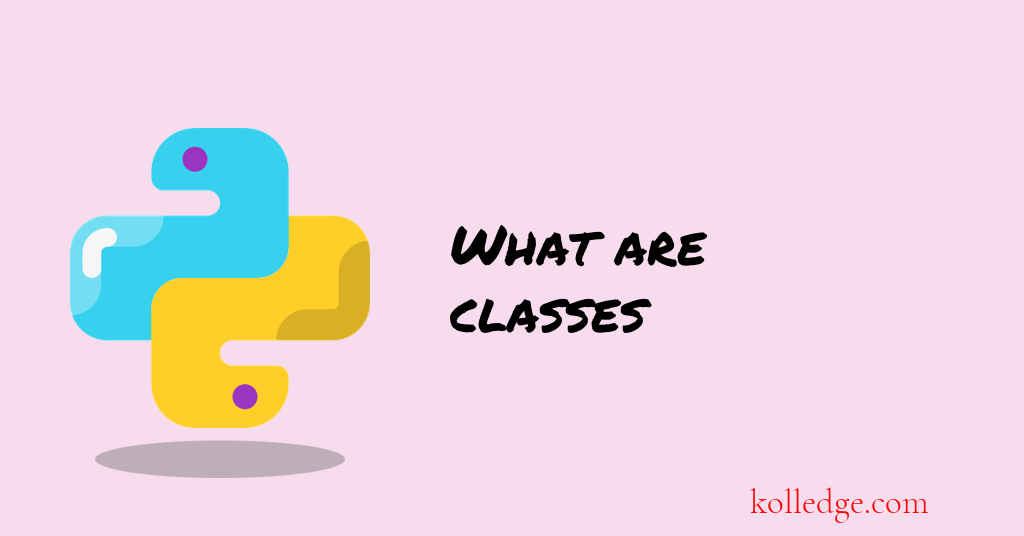
Table of Contents :
- OOPs Concepts - Classes
- Real World Example of Class
- Programming World Example of Class
- Creating objects using the Student class
OOPs Concepts - Classes
- A class is a template for creating objects.
- A class binds data and functions together.
- A class is actually a user defined data type.
- A class defines the set of data and functions that are common to all its objects.
- A class contains :
- data members - The data of the objects
- member functions - The functions that operate on that data.
- The data members and member functions can be accessed by instantiating a class i.e. by creating objects of the class.
Real World Example of Class
If we take a simple real world example of a class. Lets treat Vehicles as a class.
- Vehicles is a class
- Car, Scooter, bi-cycle, bus are all vehicles hence they are objects of Vehicles class.
- The class can have data like :
- number of tires
- price
- Mileage
- top-speed
- Each object of the vehicles class will have some number of tires but it may be different for different objects.
- e.g. Car will have four tires whereas scooter will have two tires.
- Each object of the vehicles class will have some price but it will be different for all objects.
- Each object of the vehicles class will have some top-speed but it will be different for all objects.
- The Vehicles class can have functions like :
- start the vehicle
- run the vehicle on top-speed
- refuel the vehicle
- These functions can be performed on each object of the vehicles class.
Programming World Example of Class
If we take a simple programming world example of a class. Lets take a class Student.
Student
is a class.- Rob, Stephen, Angela, Smith are all students.
- Hence
Rob
,Stephen
,Angela
,Smith
are all instances of theStudent
class. - In other words they are the objects of the
Student
class. - The state of the students is defined by the data of the
Student
class. - The behavior of the students is defined by the functions of the
Student
class. - The
Student
class can have data like :- Name
- Roll Number
- Age
- Attendance
- Marks
- Each object of the
Student
class i.e. each student will have some roll number, age, attendance, marks. - These values will be different for all students.
- The
Student
class can have behavior functions like :- Attend prayer meeting
- Read each subject
- Eat lunch in Recess.
- These functions can be performed by each object of the
Student
class.
Creating objects using the Student class :
Using the Student
class discussed above we can create different objects for each student like :
Object 1 :
State :
Name : Stephen
Roll No. : 47
Marks : 92%
Behavior :
Attend prayer meeting - Stephen attended the prayer meeting for 90% of the days.
Read each subject - Stephen read each subject 85% of the days.
Object 2 :
State :
Name : Angela
Roll No. : 12
Marks : 85%
Behavior :
Attend prayer meeting - Angela attended the prayer meeting for 80% of the days.
Read each subject - Angela read each subject 67% of the days.
Prev. Tutorial : OOPS concepts
Next Tutorial : What are Objects