Beginner Level
Intermediate Level
Advanced Level
Introduction
In computer programming, it's common to need to access files in a particular directory. In Python, there are several ways to do this, and one of the most useful is to fetch a list of all the files in a directory. This can be helpful for a range of purposes, such as organizing and managing data, automating certain tasks, or building software applications. In this tutorial, we'll cover how to fetch a list of files in a directory in Python, step by step. Whether you're a beginner or an experienced programmer, this tutorial will give you the knowledge and skills you need to get started.
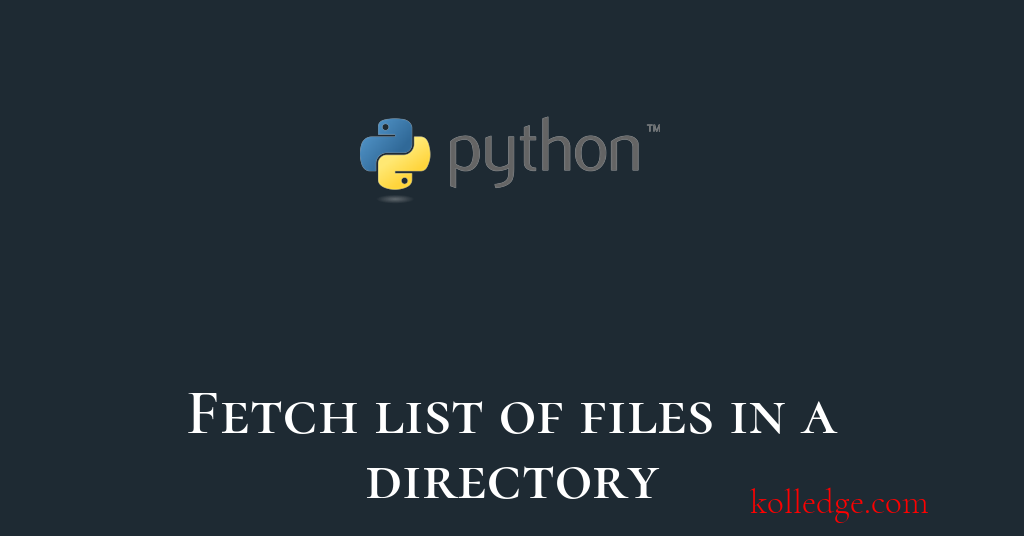
Table of Contents :
- List all files of a directory in Python
- List Files in a Directory that match a specific pattern
- List only Files from a Directory
- Using Generator Expression to List Files of a Directory
- List both Files and Directories
- Using os.walk() to List All Files in Directory and Subdirectories
- Use os.scandir() to Get Files of a Directory
- Using Glob Module to List Files of a Directory
- How to Use Pathlib Module to List Files of a Directory
List all files of a directory in Python
- Use the
os
module to list all the files of a directory. - Use the
os.listdir()
method to get a list of all files and directories in the specified directory. - Code sample :
import os
files = os.listdir("/path/to/directory")
print(files)
List Files in a Directory that match a specific pattern :
- Use the
glob
module to list all the files of a directory that match a specific pattern. - Code sample :
import glob
files = glob.glob("/path/to/directory/*.txt")
print(files)
List only Files from a Directory
- Use the
os
module to list only files from a directory. - Code sample :
import os
files = [file for file in os.listdir("/path/to/directory") if os.path.isfile(os.path.join("/path/to/directory", file))]
print(files)
Using Generator Expression to List Files of a Directory
- Use the
os
module to generate a list of files in a directory using a generator expression. - Code sample :
import os
files = (file for file in os.listdir("/path/to/directory") if os.path.isfile(os.path.join("/path/to/directory", file)))
for file in files:
print(file)
List both Files and Directories
- Use the
os
module to list both files and directories in a directory. - Code sample :
import os
files = os.listdir("/path/to/directory")
print(files)
Using os.walk() to List All Files in Directory and Subdirectories
- Use the
os
module to list all files in a directory and its subdirectories. - Code sample :
import os
for root, dirs, files in os.walk("/path/to/directory"):
for file in files:
print(os.path.join(root, file))
Use os.scandir() to Get Files of a Directory
- Use the
os
module to get files of a directory using `os.scandir()`. - Code sample :
import os
with os.scandir("/path/to/directory") as directory:
for entry in directory:
if entry.is_file():
print(entry.name)
Using Glob Module to List Files of a Directory
- Use the
glob
module to list files of a directory.
import glob
files = glob.glob("/path/to/directory/*.txt")
print(files)
How to Use Pathlib Module to List Files of a Directory
- Use the
pathlib
module to list files of a directory.
from pathlib import Path
files = [file.name for file in Path("/path/to/directory").iterdir() if file.is_file()]
print(files)
Prev. Tutorial : Directory operations
Next Tutorial : Delete Files and Directories