Beginner Level
Intermediate Level
Advanced Level
Introduction
One of the fundamental skills in Python programming is writing data to a file, which is essential for storing large amounts of data, processing and analyzing it later, and sharing information with others. Writing to a file in Python can be done using a variety of methods, and this tutorial will cover them all in detail, including opening and closing files, writing and appending to files, handling errors, and formatting output. By the end of this tutorial, you will be able to write to any type of file using Python with ease.
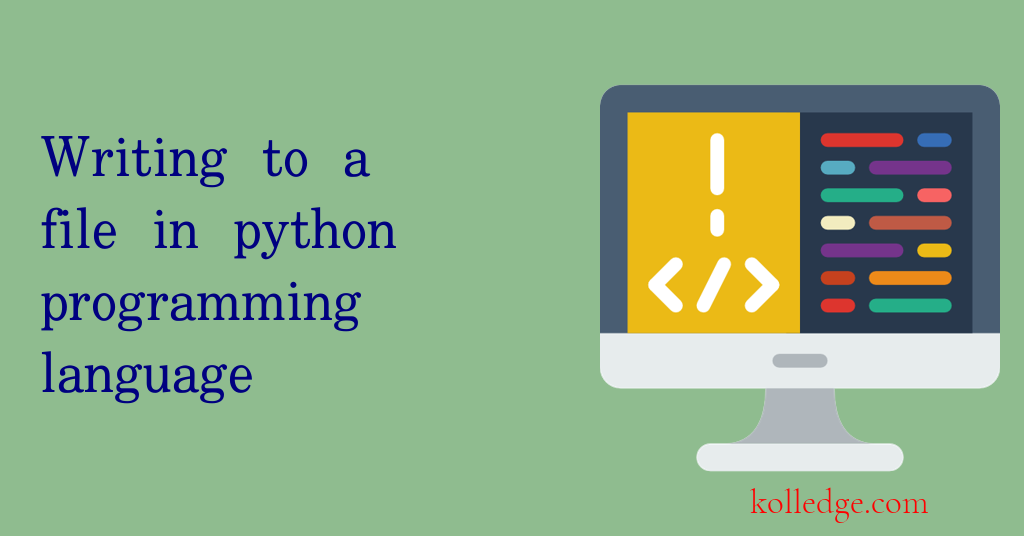
Table of Contents :
- Access Modes for Writing a file
- Different modes for Writing the file in Python
- Steps for Writing Data into a File in Python
- How to write to a Text file in Python
- How to write to an existing File in Python
- Different Write Methods in Python
- write() Method
- writelines() Method
- Using with Statement to Write a File
- How to append new Content to an Existing File
- How to append and Read on the Same File
- How to write to a Binary File in Python
Different modes for Writing the file in Python :
w | Write mode, used to open a file for writing. If the file exists, it will be truncated. |
wb | Binary write mode, used to write binary data to a file. |
a | Append mode, used to open a file for writing. If the file does not exist, it will be created. |
at | Text append mode, used to append text data to a file. |
Steps for Writing Data into a File in Python :
- Open a file in write mode, using the open() method.
- Write data to a file, using the appropriate write method.
- Close the file using the close() method.
How to write to a text file in python :
- This code writes a string of text to a file named example.txt, using the write() method.
# Writing to a text file
file = open("example.txt", "w")
file.write("Hello, world!")
file.close()
Different write methods in python :
- write() : Writes a string of characters to a file.
- writelines() : Writes a list of lines to a file.
write() Method :
- Writes a string of characters to a file.
- Sample code for using write() method
file = open("example.txt", "w")
file.write("This is a line of text.")
file.close()
writelines() Method :
- Write a list of lines to a file
- Sample code for using writelines() method
list_of_lines = ["This is line 1.", "This is line 2.", "This is line 3."]
with open("example.txt", "w") as file:
file.writelines(list_of_lines)
Using with statement to write a file :
- The with statement can also be used when writing to a file
- It will automatically close the file after the code block has finished executing.
- Example code for using with statement to write to a file
with open("example.txt", "w") as file:
file.write("This is a line of text.")
How to write to a binary file in python :
- This code writes binary data to a file named example.bin using the wb mode.
# Writing to a binary file
with open("example.bin", "wb") as file:
file.write(b"\x48\x65\x6c\x6c\x6f\x20\x57\x6f\x72\x6c\x64\x21")
# hexadecimal representation of "Hello World!"
Prev. Tutorial : Reading a file
Next Tutorial : Append to a file