Beginner Level
Intermediate Level
Advanced Level
Introduction
In the world of programming, Boolean values are fundamental as they are used to represent true or false conditions. The Boolean data type is particularly important in Python as it is used extensively in decision-making statements, loops, and logical operations. Understanding and properly utilizing Booleans is paramount for effective programming, and this tutorial will equip you with the knowledge and skills you need to master this important data type in Python. By the end of this tutorial, you will be able to confidently work with Booleans in Python and use them efficiently in your codes. So let's get started!
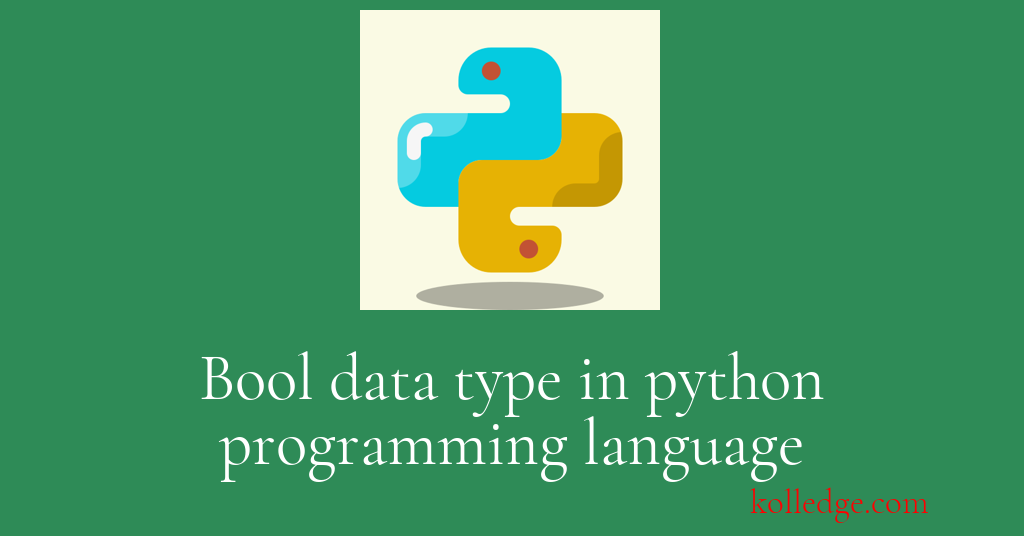
Table of Contents :
- Bool data type in Python
- Boolean Variables
Bool data type in Python
- Python has a Boolean data type, which can take on one of two values:
- True
- False
- The
bool
data type in Python is used to represent boolean values. - The bool data type is implemented using a
bool
class in Python. - Code Sample :
b = True
print(f"value of b = {b}")
print("Type of b = ", type(b))
# Output
# value of b = True
# Type of b = <class 'bool'>
- Boolean data type are used as values of expressions that have answers either yes or no e.g. comparison expressions.
if a > b:
print("a is greater")
else:
print("b is greater")
- We can use the
bool()
function to convert other data types to Boolean values. - For example, we can convert an integer to a Boolean value like this:
bool_var = bool(1)
# The bool() function will return True if the value is anything other than zero,
# and False if the value is zero.
Boolean Variables
- A Boolean variable can have only two possible values -
True
orFalse
. - We can create a Boolean variable by assigning the value True or False to it :
bool_var = True
- We can use boolean variables in logical expressions and if statements, such as this one:
bool_var = True
if bool_var:
print("bool_var is True")
else:
print("bool_var is False")
# Output
# bool_var is True
- We can also use Boolean variables with the 'and' and 'or' operators:
bool_var1 = True
bool_var2 = False
if bool_var1 and bool_var2:
print("Both bool_var1 and bool_var2 are True")
if bool_var1 or bool_var2:
print("At least one of bool_var1 or bool_var2 is True")
# Output
# At least one of bool_var1 or bool_var2 is True
- We can use the 'not' operator to invert a Boolean variable:
bool_var = True
if not bool_var:
print("bool_var is False")
else:
print("bool_var is True")
# Output
# bool_var is True
Prev. Tutorial : Memoryview data type
Next Tutorial : Arrays in Python