Introduction
Python is a powerful programming language that offers a wide range of functionalities for various applications. One of the most common operations in Python programming is splitting a string. Splitting a string enables one to break a string into smaller parts based on a specific delimiter or criteria. Python provides a straightforward and efficient method for performing string splitting tasks. In this tutorial, we will be exploring how to split a string in Python and the different approaches and string functions that can be used to achieve this. Whether you are a beginner or an experienced Python developer, this tutorial will provide you with insights on how to split strings in Python effectively.
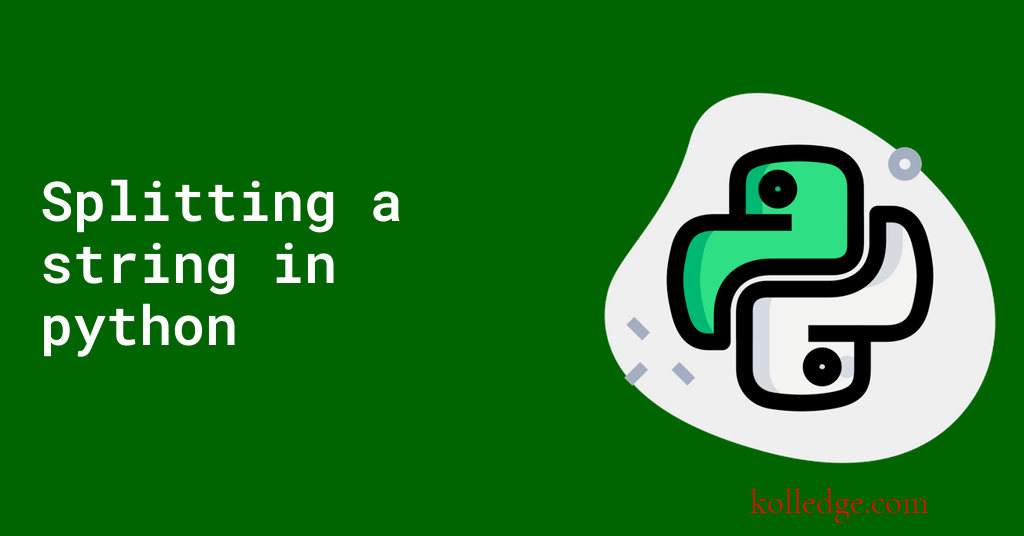
Table of Contents :
- Different methods of splitting a string in Python :
- Using Iteration
- Using regular expressions
- Using split method
- Using Itertools
- Using the list() method
- Removing Whitespace with split()
Different methods of splitting a string in Python :
- In python we can split a string using different methods, these include :
- Using Iteration
- Using regular expressions
- Using split method
- Using Itertools
- Using the list() method
Splitting a string using Iteration:
- To split a string into words using iteration :
- Code Sample :
my_string = "Kolledge, Scholars"
delimiter = ", "
substrings = []
current_substring = ""
for character in my_string:
if character == delimiter:
substrings.append(current_substring)
current_substring = ""
else:
current_substring += character
substrings.append(current_substring)
print(substrings)
# Output
# ['Kolledge, Scholars']
Splitting a string using regular expressions :
- We can use the
re.split()
method, which uses regular expressions to split a string based on a pattern. - We need to import the
re
module to use there.split()
method. - Code Sample :
import re
my_string = "Kolledge, Scholars"
delimiter = ", "
substrings = re.split(delimiter, my_string)
print(substrings)
# Output
# ['Kolledge', 'Scholars']
Splitting a string using split method :
- We can use the
split()
method to split a string based on a separator. - Code Sample :
my_string = "Kolledge, Scholars"
delimiter = ", "
substrings = my_string.split(delimiter)
print(substrings)
# Output
# ['Kolledge', 'Scholars']
Splitting a string Using Itertools:
- We can use the
itertools.groupby()
method to group adjacent characters based on a condition. - The
groupby()
method takes a parameterkey
.- We can pass a lambda function to this parameter.
- This lambda function returns False when the delimiter is encountered or the end of the string is reached.
- We need to import the
itertools
module to use this method. - Code Sample :
import itertools
my_string = "Kolledge, Scholars"
delimiter = ", "
substrings = [''.join(group) for is_delimiter, group in itertools.groupby(my_string, key=lambda x: x != delimiter) if is_delimiter]
print(substrings)
# Output
# ['Kolledge', 'Scholars']
Splitting a string Into a List of Characters:
- We can use the
list()
method to convert a string into a list of characters. - Code Sample :
my_string = "Kolledge"
character_list = list(my_string)
print(character_list)
# Output
# ['K', 'o', 'l', 'l', 'e', 'd', 'g', 'e']
Removing White-space with split():
- We can use the
split()
method without any arguments to split a string by white-space characters. - Code Sample :
my_string = " Kolledge, Scholars "
substrings = my_string.split()
print(substrings)
# Output
# ['Kolledge,', 'Scholars']
Prev. Tutorial : Slicing a string
Next Tutorial : Getting substring