Introduction
In Python, instance variables are variables that are defined within a class and their values are unique to each instance or object of that class. They are also sometimes referred to as object variables because their values are specific to each individual object. Instance variables can be accessed and modified by methods within the class or by the object created from the class. Understanding instance variables is essential to creating complex and dynamic Python programs. So, in this tutorial, we'll dive into the world of instance variables, explain their importance, and provide you with practical examples to help you better understand how to use them in your programming.
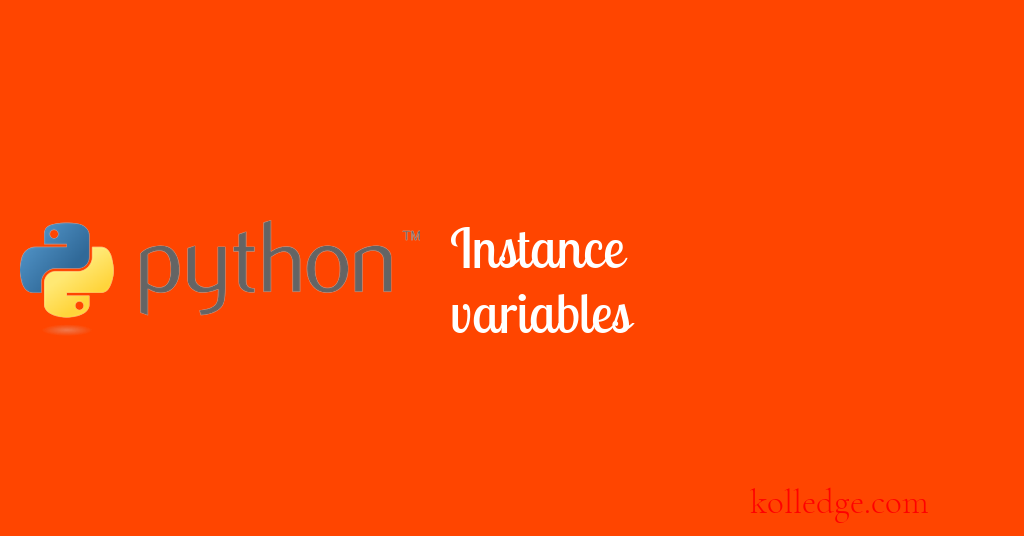
Table of Contents :
- Instance variables
- Accessing instance variables
- using the object name and dot operator.
- using the self keyword and dot operator.
- using the getattr() method.
- Modifying instance variables
- using the object name and dot operator
- using the self keyword and dot operator
- using the setattr() method
- Fetching Instance variables using __dict__ function
- Adding instance variables dynamically
- Deleting instance variables dynamically
- using del keyword
- using delattr() function
Instance variables
- The data members of the class that are attached to objects are called instance variables.
- A separate copy of instance variables is created for each object.
- The value of the instance variable is different for each object.
- Instance variables of a class are also called instance attributes.
- Instance variables are used within instance methods of the class.
- Instance variables are defined and initialized inside the constructor of the class.
- Code Sample :
class Employee:
def __init__(self, emp_name, emp_id, emp_desig):
# data members (instance variables - name, id and desig)
self.name = emp_name
self.id = emp_id
self.desig = emp_desig
Accessing instance variables :
- We can access instance variables in python using the following three methods :
- using the object name and dot operator
- using the self keyword and dot operator
- using the getattr() method
Using the object name and dot operator :
- The basic syntax of using an instance variable is :
object_name.instance_var_name
- Code Sample :
class Employee:
def __init__(self, emp_name, emp_id, emp_desig):
# data members (instance variables - name, id and desig)
self.name = emp_name
self.id = emp_id
self.desig = emp_desig
# Employee 1 object
emp_1 = Employee("Steve", "k2591", "Manager")
# Accessing instance variables for employee 1
# Using object_name and dot operator
employee_name = emp_1.name
employee_id = emp_1.id
employee_desig = emp_1.desig
# Printing details for employee 1
print(f"Name of employee = {employee_name}")
print(f"ID of employee = {employee_id}")
print(f"Designation of employee = {employee_desig}")
print()
# Output
# Name of employee 1 = Steve
# ID of employee 1 = k2591
# Designation of employee 1 = Manager
Using the self keyword and dot operator :
- Inside instance methods
self
keyword can be used to access instance variable - The syntax for accessing instance variable is :
self.instance_var_name
- Here
self
refers to the current object. - Code Sample :
class Employee:
def __init__(self, emp_name, emp_id, emp_desig):
# data members (instance variables - name, id and desig)
self.name = emp_name
self.id = emp_id
self.desig = emp_desig
# Instance method
def print_details(self):
# Accessing instance variables for employees
# Using self keyword and dot operator
print(f"Name of employee = {self.name}")
print(f"ID of employee = {self.id}")
print(f"Designation of employee = {self.desig}")
# Employee 1
emp_1 = Employee("Steve", "k2591", "Manager")
emp_1.print_details()
print()
# Employee 2
emp_2 = Employee("Rob", "k2586", "Tech Lead")
emp_2.print_details()
# Output
# Name of employee = Steve
# ID of employee = k2591
# Designation of employee = Manager
# Name of employee = Rob
# ID of employee = k2586
# Designation of employee = Tech Lead
Using the getattr() method :
- To get the value of instance variable we can use the
getattr()
method. - we can pass two arguments to the
getattr()
method- the object reference and
- the instance variable name.
- Code Sample :
class Employee:
def __init__(self, emp_name, emp_id, emp_desig):
# data members (instance variables - name, id and desig)
self.name = emp_name
self.id = emp_id
self.desig = emp_desig
# Employee 1 object
emp_1 = Employee("Steve", "k2591", "Manager")
# Accessing instance variables for employee 1
# Using getattr method and dot operator
employee_name = getattr(emp_1,'name')
employee_id = getattr(emp_1,'id')
employee_desig = getattr(emp_1,'desig')
# Printing details for employee 1
print(f"Name of employee = {employee_name}")
print(f"ID of employee = {employee_id}")
print(f"Designation of employee = {employee_desig}")
print()
# Output
# Name of employee = Steve
# ID of employee = k2591
# Designation of employee = Manager
Modifying instance variables :
- We can modify the value of an instance variable using the following methods in Python :
- using the object name and dot operator
- using the self keyword and dot operator
- using the setattr() method
- As all objects have their separate copy of instance variables, when we modify the value of an instance variable for an object, the change is not replicated for other objects.
Using the object name and dot operator
- We can modify the value of an instance variable for an object using the dot operator and object name
- The basic syntax of modifying instance variable is :
object_name.instance_var_name = new_value
- Code Sample :
class Employee:
def __init__(self, emp_name, emp_id, emp_desig):
# data members (instance variables - name, id and desig)
self.name = emp_name
self.id = emp_id
self.desig = emp_desig
# Employee 1 object
emp_1 = Employee("Steve", "k2591", "Manager")
# Printing Initial details for employee 1
print(f"Name of employee = {emp_1.name}")
print(f"ID of employee = {emp_1.id}")
print(f"Designation of employee = {emp_1.desig}")
print()
# Modifying the value of desig instance variable
emp_1.desig = "Sr. Manager"
# Printing Modified details for employee 1
print(f"Name of employee = {emp_1.name}")
print(f"ID of employee = {emp_1.id}")
print(f"Designation of employee = {emp_1.desig}")
print()
# Output
# Name of employee = Steve
# ID of employee = k2591
# Designation of employee = Manager
# Name of employee = Steve
# ID of employee = k2591
# Designation of employee = Sr. Manager
using the self keyword and dot operator
- Inside the instance method we can use the
self
keyword to modify the value of instance variables. - The syntax of modifying instance variable using self keyword is :
self.instance_var_name = new_value
- Code Sample :
class Employee:
def __init__(self, emp_name, emp_id, emp_desig):
# data members (instance variables - name, id and desig)
self.name = emp_name
self.id = emp_id
self.desig = emp_desig
def print_details(self):
print(f"Name of employee = {self.name}")
print(f"ID of employee = {self.id}")
print(f"Designation of employee = {self.desig}")
print()
def modify_designation(self,new_value):
# Modifying the value of desig instance variable
# Using self keyword
self.desig = new_value
# Employee 1 object
emp_1 = Employee("Steve", "k2591", "Manager")
# Printing Initial details for employee 1
emp_1.print_details()
emp_1.modify_designation("Sr. Manager")
# Printing Modified details for employee 1
emp_1.print_details()
# Output
# Name of employee = Steve
# ID of employee = k2591
# Designation of employee = Manager
# Name of employee = Steve
# ID of employee = k2591
# Designation of employee = Sr. Manager
Using the setattr() Method :
- To modify the value of instance variables in python we can use the
setattr()
method. - we can pass three arguments to the
setattr()
method- the object reference,
- the instance variable name, and
- the new value of the instance variable
- Code Sample :
class Employee:
def __init__(self, emp_name, emp_id, emp_desig):
# data members (instance variables - name, id and desig)
self.name = emp_name
self.id = emp_id
self.desig = emp_desig
def print_details(self):
print(f"Name of employee = {self.name}")
print(f"ID of employee = {self.id}")
print(f"Designation of employee = {self.desig}")
print()
# Employee 1 object
emp_1 = Employee("Steve", "k2591", "Manager")
# Printing Initial details for employee 1
emp_1.print_details()
# Modifying the value of desig instance variable
# Using setattr method
setattr(emp_1,"desig","Sr. Manager")
# Printing Modified details for employee 1
emp_1.print_details()
# Output
# Name of employee = Steve
# ID of employee = k2591
# Designation of employee = Manager
# Name of employee = Steve
# ID of employee = k2591
# Designation of employee = Sr. Manager
Fetching Instance variables using __dict__ function :
- If we need to fetch the names and values of all the instance variables of an object, we can use the
__dict__
function of that object. - The __dict__function returns a dictionary where :
- the keys of the dictionary are the names of the instance variables.
- the values of the dictionary are the values of the variables.
- Code Sample :
class Employee:
def __init__(self, emp_name, emp_id, emp_desig):
# data members (instance variables - name, id and desig)
self.name = emp_name
self.id = emp_id
self.desig = emp_desig
emp_1 = Employee("Steve", "k2591", "Manager")
inst_variables = emp_1.__dict__
print(inst_variables)
# Output
# {'name': 'Steve', 'id': 'k2591', 'desig': 'Manager'}
Adding instance variables dynamically
- We can add new instance variables to an object using dot operator.
- We cannot add an instance variable directly to the class dynamically.
- When we add a new instance variable to an object, this new instance variable is not added to the remaining objects.
- In the code sample given below we can see that the new instance variable
mail
is added only toemp_1
object and the details ofemp_2
object remain the same. - Code Sample :
class Employee:
def __init__(self, emp_name, emp_id, emp_desig):
# data members (instance variables - name, id and desig)
self.name = emp_name
self.id = emp_id
self.desig = emp_desig
emp_1 = Employee("Steve", "k2591", "Manager")
emp_2 = Employee("Rob", "k2586", "Tech Lead")
print(emp_1.__dict__) # Print initial details of employee 1
print(emp_2.__dict__) # Print initial details of employee 2
print()
emp_1.email = "steve@mail.com" # Add new instance variable to employee 1
print(emp_1.__dict__) # Print new details of employee 1
print(emp_2.__dict__) # Print new details of employee 2
# Output
# {'name': 'Steve', 'id': 'k2591', 'desig': 'Manager'}
# {'name': 'Rob', 'id': 'k2586', 'desig': 'Tech Lead'}
# {'name': 'Steve', 'id': 'k2591', 'desig': 'Manager', 'email': 'steve@mail.com'}
# {'name': 'Rob', 'id': 'k2586', 'desig': 'Tech Lead'}
Deleting instance variables dynamically
- We can delete an instance variable of an object using :
- del keyword
- delattr() function
- When we delete an instance variable of an object, the instance variables of the remaining objects remain the same.
- In the code samples given below we can see that the instance variable
desig
is deleted only fromemp_1
object and the details ofemp_2
object remain the same.
Using del keyword :
- We can delete an instance variable of an object using the del keyword.
- Code Samples :
class Employee:
def __init__(self, emp_name, emp_id, emp_desig):
# data members (instance variables - name, id and desig)
self.name = emp_name
self.id = emp_id
self.desig = emp_desig
emp_1 = Employee("Steve", "k2591", "Manager")
emp_2 = Employee("Rob", "k2586", "Tech Lead")
print(emp_1.__dict__) # Print initial details of employee 1
print(emp_2.__dict__) # Print initial details of employee 2
print()
del emp_1.desig # Delete instance variable desig of employee 1
print(emp_1.__dict__) # Print new details of employee 1
print(emp_2.__dict__) # Print new details of employee 2
# Output
# {'name': 'Steve', 'id': 'k2591', 'desig': 'Manager'}
# {'name': 'Rob', 'id': 'k2586', 'desig': 'Tech Lead'}
# {'name': 'Steve', 'id': 'k2591'}
# {'name': 'Rob', 'id': 'k2586', 'desig': 'Tech Lead'}
Using delattr() function :
- We can delete an instance variable of an object using the
delattr()
function. - The
delattr()
function takes two arguments :- the object whose attribute needs to be deleted
- the name of the instance variable that we want to delete
- Code Samples :
class Employee:
def __init__(self, emp_name, emp_id, emp_desig):
# data members (instance variables - name, id and desig)
self.name = emp_name
self.id = emp_id
self.desig = emp_desig
emp_1 = Employee("Steve", "k2591", "Manager")
emp_2 = Employee("Rob", "k2586", "Tech Lead")
print(emp_1.__dict__) # Print initial details of employee 1
print(emp_2.__dict__) # Print initial details of employee 2
print()
delattr(emp_1, "desig") # Delete instance variable desig of employee 1
print(emp_1.__dict__) # Print new details of employee 1
print(emp_2.__dict__) # Print new details of employee 2
# Output
# {'name': 'Steve', 'id': 'k2591', 'desig': 'Manager'}
# {'name': 'Rob', 'id': 'k2586', 'desig': 'Tech Lead'}
# {'name': 'Steve', 'id': 'k2591'}
# {'name': 'Rob', 'id': 'k2586', 'desig': 'Tech Lead'}
Prev. Tutorial : Destructors
Next Tutorial : Class variables