Beginner Level
Intermediate Level
Advanced Level
Introduction
Sets are one of the important data structures in Python that allow you to store unique and unordered values. Understanding how to perform different set operations, such as union, intersection, and difference, is essential for efficient data manipulation and analysis in Python. In this tutorial, we will be focusing on the difference operation on sets. We will explain what it is, how to implement it in Python, and provide some examples to help you better understand its applications. Let's get started!
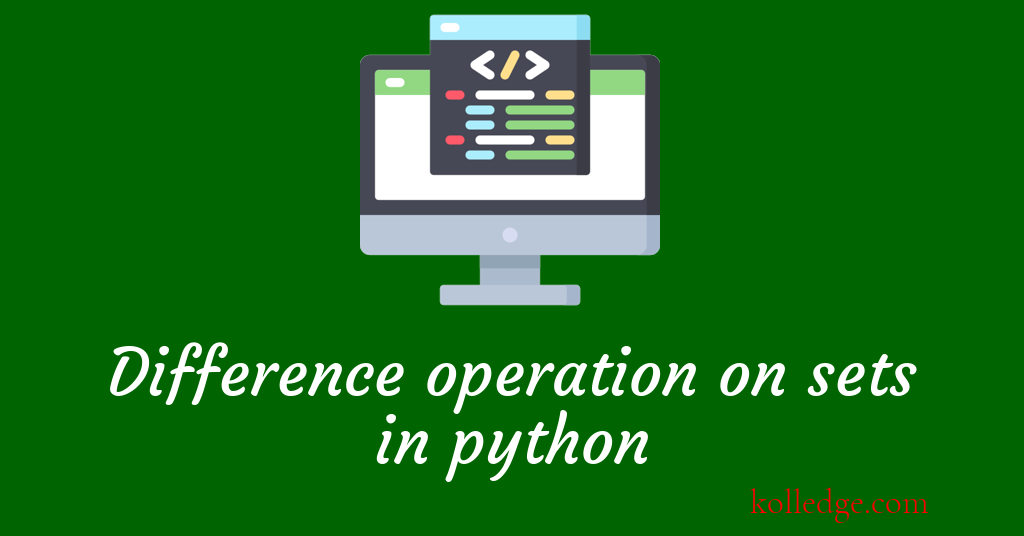
Table of Contents :
- Difference operation
- Difference operation using the difference method
- Difference operation using the set difference operator
Difference operation :
- Difference operation of two sets in Python returns a new set which contains the elements of the first set that do not exist in the second set.
- We can do difference on sets in Python using the :
- difference() method
- set difference operator '-'
Difference operation using the difference method :
- The built-in
difference()
method can be used to get difference of two sets in Python. - The basic syntax of using the
difference()
method is as follows :new_set = set_1.difference(set_2)
- The
difference()
method can also accept iterable objects as arguments. - The
difference()
method converts the iterable object into set before applying the difference operation. - Note # : The difference operation is not commutative i.e.
set_1.difference(set_2)
is not the same asset_2.difference(set_1)
- Code Sample :
set_1 = {1, 2, 3, 4}
set_2 = {2, 4, 5, 6, 7}
new_set = set_1.difference(set_2)
new_set_2 = set_2.difference(set_1)
print(f"Value of set_1 = {set_1}")
print(f"Value of set_2 = {set_2}")
print(f"Value of new_set = {new_set}")
print(f"Value of new_set_2 = {new_set_2}")
# Output
# Value of set_1 = {1, 2, 3, 4}
# Value of set_2 = {2, 4, 5, 6, 7}
# Value of new_set = {1, 3}
# Value of new_set_2 = {5, 6, 7}
Difference operation using the set difference operator '-' :
- The set difference operator
-
can be used in python to find difference of two sets. - The basic syntax of using a set difference operator is as follows :
new_set = set_1 - set_2
- The set difference operator allows only sets as operands.
- We cannot find difference of iterable objects with set difference operator.
- Code Sample :
set_1 = {1, 2, 3, 4}
set_2 = {2, 4, 5, 6, 7}
new_set = set_1 - set_2
print(f"Value of set_1 = {set_1}")
print(f"Value of set_2 = {set_2}")
print(f"Value of new_set = {new_set}")
# Output
# Value of set_1 = {1, 2, 3, 4}
# Value of set_2 = {2, 4, 5, 6, 7}
# Value of new_set = {1, 3}
Prev. Tutorial : Intersection operation on sets
Next Tutorial : Symmetric difference on sets