Beginner Level
Intermediate Level
Advanced Level
Introduction
Inheritance is one of the key concepts in object-oriented programming. It allows programmers to create new classes based on existing ones, thereby promoting code reusability, flexibility, and scalability. Inheritance plays a vital role in coding since it helps to reduce the code complexity and enhance the readability. Understanding how inheritance works in programming is essential for every developer who wants to write efficient and maintainable code. In this tutorial, we will explore the basics of inheritance and how to implement it in your code.
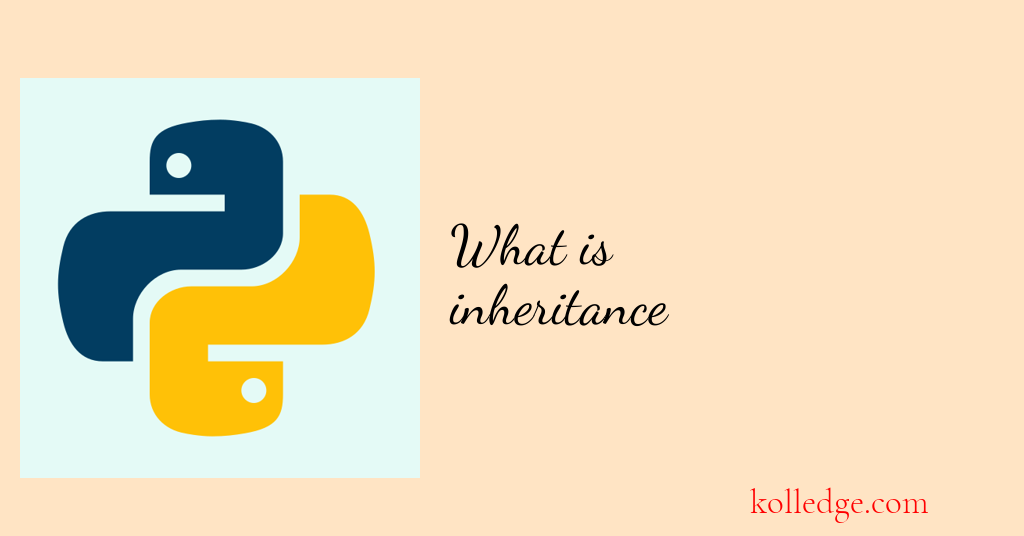
Table of Contents :
- OOPs Concepts - Inheritance
- Real world example of Inheritance
OOPs Concepts - Inheritance
- Inheritance is an object-oriented programming concept in which one class can derive characteristics from another class.
- If class A need to include some properties that are already present in class B, then class A can inherit from class B rather than writing those properties all over again.
- Inheritance is an important tool of ensuring code reuse.
- The class that inherits is called the child class.
- The class from which properties are inherited is called parent class.
- There are different types of inheritance -
- Single inheritance - If child class has only one parent class
- Multiple inheritance - If child class has more than one parent classes
- Multilevel inheritance - If a class inherits from a class which itself inherits from another class.
- Hierarchical inheritance - If one parent class has many child classes.
- Hybrid inheritance - A combination of multiple and multilevel inheritance.
Real world example of Inheritance
- Suppose we have a class
Employee
. -
Employee
class has following data members :
Employee :
Name of the Employee
Employee Id
Designation
experience
email-Id
Address
Attendance
Perks
- Suppose we want to create a class
Contract_Employee
: -
Contract_Employee
will have all the data members of the employee class. - In addition to these,
Contract_Employee
will also have a data membercontract_period
. - So instead of writing the above data members all over again -
-
Contract_Employee
class will inherit fromEmployee
class - then it will add the new data member
contract_period
in its definition.
-
- The
Contract_Employee
class will hence have the following data members :
Contract_Employee :
Name of the Employee
Employee Id
Designation
experience
email-Id
Address
Attendance
Perks
contract_period
Prev. Tutorial : What is Data Abstraction
Next Tutorial : What is Polymorphism