Beginner Level
Intermediate Level
Advanced Level
Introduction
Python is a versatile programming language that allows us to create custom data structures that fit a wide range of use cases. One such data structure is a custom sequence type, which allows us to create our own sequence of values that can be accessed using standard indexing and slicing operations. By creating our own sequence type, we can tailor it to specific needs, such as supporting custom behaviors when elements are added or removed, or implementing custom iteration patterns. In this tutorial, we will explore how to create our own custom sequence type in Python, and examine some practical use cases for it.
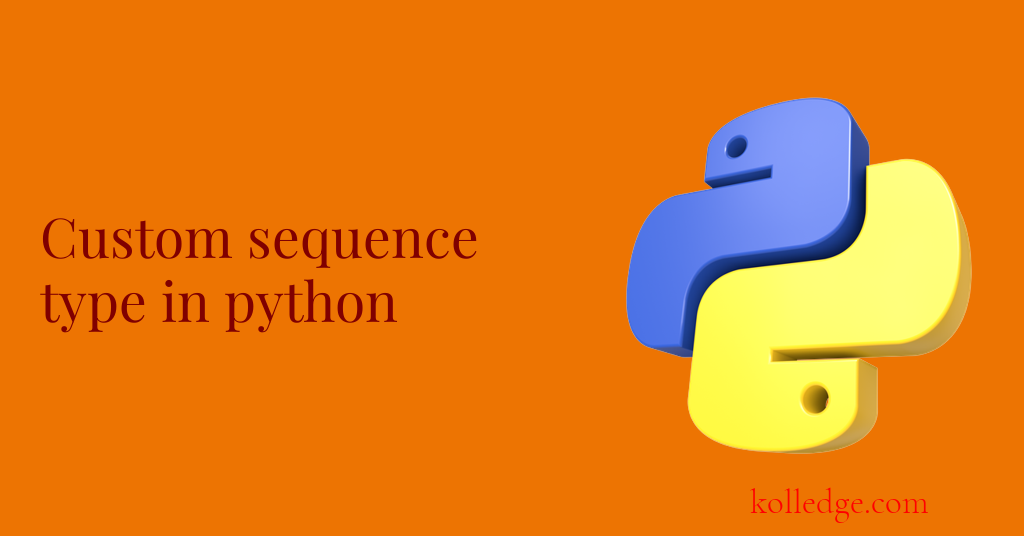
Table of Contents :
- Custom sequence type
- __getitem__ Method
- The __len__ Method
- Fibonacci Sequence
- Using Fibonacci Sequence
- Adding Slicing Support to custom sequence class
Custom sequence type :
- A sequence type is a type of object in Python that represents a sequence of values.
- A custom sequence type is a sequence type that is defined by the user.
__getitem__ Method :
- The
__getitem__()
method is used to implement the sequence indexing and slicing operations. - It takes one or two arguments: the index or slice object.
- Code Sample :
class MySequence:
def __getitem__(self, index):
# implementation of indexing and slicing operations
The __len__ Method :
- The
__len__()
method is used to implement thelen()
built-in function for the sequence. - It should return the length of the sequence.
- Code Sample :
class MySequence:
def __len__(self):
# implementation of the length method
Fibonacci Sequence :
- The Fibonacci sequence is a sequence of numbers where each number is the sum of the two preceding ones.
- The sequence starts with 0 and 1.
- To create a custom sequence class for the Fibonacci sequence, we can implement the
__getitem__()
and__len__()
methods accordingly. - Code Sample :
class Fibonacci:
def __init__(self, n):
self.n = n
def __getitem__(self, k):
if isinstance(k, slice):
start, stop, step = k.indices(self.n)
return [self[i] for i in range(start, stop, step)]
elif isinstance(k, int):
if k < 0:
k = self.n + k
if k < 0 or k >= self.n:
raise IndexError
a, b = 0, 1
for i in range(k):
a, b = b, a + b
return a
else:
raise TypeError
def __len__(self):
return self.n
Using Fibonacci Sequence
- Now that we have created the custom sequence class for the Fibonacci sequence, we can use it like any other sequence object in Python.
- Code Sample :
fib = Fibonacci(10)
print(fib[3]) # Output: 2
print(fib[:5]) # Output: [0, 1, 1, 2, 3]
print(len(fib)) # Output: 10
Adding Slicing Support to custom sequence class :
- We can add slicing support to our custom sequence class by checking if the
__getitem__()
method is called with a slice object. - We can then extract the start, stop, and step values from the slice object and return a list of the corresponding sequence values.
Prev. Tutorial : iter() function
Next Tutorial : Generator functions