Beginner Level
Intermediate Level
Advanced Level
Introduction
In Python, functions are an essential part of the language, and they allow you to create reusable blocks of code that can be called multiple times with different arguments. However, sometimes we may want to create a new function that's based on an existing one but with some of its arguments already set to specific values. This is where Partial Functions come in handy. By using Partial Functions, you can create new functions that are tailored to specific use cases while still leveraging the power and flexibility of the original function.
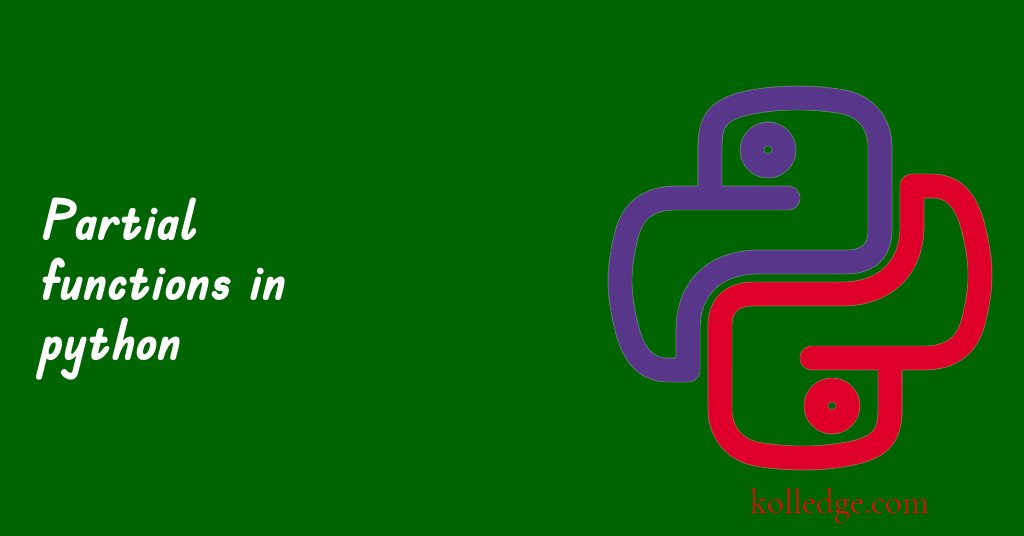
Table of Contents :
- Partial Functions in Python
- Using *args with partial functions
- Using **kwargs with partial functions
Partial Functions in Python :
- Python function is a mechanism in Python that can be used to reduce the complexity any function signature.
- The signature of any function is simplified by reducing the number of arguments that need to be passed to the function call.
- Many a times there are some arguments of a function that remain same for several function calls.
- These function arguments can be excluded using partial functions.
- partial functions assigns a default value to the arguments that won't change and returns a partial object.
- This partial object can be used to call the original function but with default values.
- The name of this partial object can be used to call the original function.
- The function can then be called by this new name without passing the arguments that won't change.
- Partial functions are available in the functools standard module.
- The syntax of import statement for using partial functions is :
from functools import partial
- The basic syntax of using partial functions is :
from functools import partial
# using partial function
partial_object = partial(origianl_fn, var=default_value)
- If we pass a value for the argument with default value, even while calling with partial object - the new value will be used and not the default value.
- Code Sample :
def my_func(a, b, c, d):
print("Value of a = ", a)
print("Value of b = ", b)
print("Value of c = ", c)
print("Value of d = ", d)
partial_my_func = partial(my_func,10,20,30)
partial_my_func(100)
# Output
# Value of a = 10
# Value of b = 20
# Value of c = 30
# Value of d = 100
Using *args with partial functions
- If we pass additional arguments to a partial object - they are appended to *args
- Code Sample :
def my_func(a, b, *args):
print("Value of a = ", a)
print("Value of b = ", b)
print("Value of args = ", args)
partial_my_func = partial(my_func,10,20,30,40,50)
partial_my_func()
# Output
# Value of a = 10
# Value of b = 20
# Value of args = (30, 40, 50)
Using **kwargs with partial functions
- If we pass additional keyword arguments to a partial object - they are appended to **kwargs
- Code Sample :
def my_func(a, b, **kwargs):
print("Value of a = ", a)
print("Value of b = ", b)
print("Value of kwargs = ", kwargs)
partial_my_func = partial(my_func,10,20,first=40,second=50)
partial_my_func()
# Output
# Value of a = 10
# Value of b = 20
# Value of kwargs = {'first': 40, 'second': 50}
Prev. Tutorial : Keyword Arguments
Next Tutorial : Recursion