Introduction
Python is a powerful programming language that provides a multitude of tools for developers to tackle various tasks. One of the most useful tools in Python is list comprehensions. List comprehensions provide a concise and readable way to create lists based on existing lists or other iterable objects. They are extremely efficient and can make your code more readable and maintainable. In this tutorial, we will cover the fundamentals of list comprehensions in Python and explore how they can be used to simplify your code and increase your productivity. Whether you're a seasoned Python developer or just starting out, this tutorial will equip you with the necessary skills to use list comprehensions in your projects.
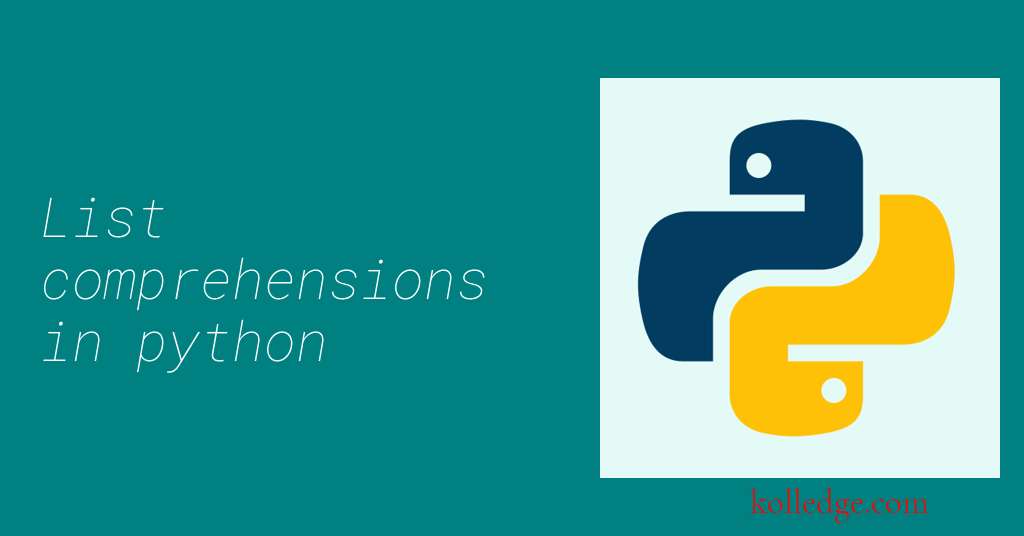
Table of Contents :
- List comprehensions in Python
- Using List comprehensions to filter elements in List
List comprehensions in Python :
- Sometimes we need to apply some logic to each element of the list.
- We have already read about the map function in Python that does this job.
- List comprehensions is a more concise and elegant way of doing this job.
- the basic syntax for list comprehension is :
new_list = [output_expression for list_item in orig_list]
- Here -
- orig_list is the input list for list comprehension.
- list_item represents each element of the list as it is iterated.
- output_expression is the transformation logic that we want to apply on each element of the list.
- new_list is the final list returned after applying the logic.
- Suppose we want to multiply each element of the list by 5, then list comprehension will be -
new_list = [list_item * 5 for list_item in orig_list]
Using List comprehensions to filter elements in List :
- Sometimes we need to filter out some elements of the list based on some criteria.
- We have already read about the filter function in Python that does this job.
- List comprehensions is a more concise and elegant way of doing this job.
- the basic syntax for list comprehension with if clause is :
new_list = [list_item for list_item in orig_list if condition]
- Here :
- orig_list is the input list for list comprehension.
- list_item represents each element of the list as it is iterated.
- new_list is the final list returned after filtering the elements.
- Suppose we want to filter elements greater than 5, then list comprehension will be :
new_list = [list_item for list_item in orig_list if list_item > 5]
- Code Sample :
my_list = [10, 20, 30, 40]
print("my_list = ", my_list)
new_list = [x for x in my_list if 15 < x < 35]
print("new_list = ", new_list)
# Output
# my_list = [10, 20, 30, 40]
# new_list = [20, 30]
Prev. Tutorial : Filter List/Tuple elements
Next Tutorial : Reducing a list