Beginner Level
Intermediate Level
Advanced Level
Introduction
We have read that by creating modules, developers can easily create reusable code blocks that can be shared across different projects, making it easier to maintain and scale applications. This Python tutorial will cover the basics of creating modules in Python. Whether you're a beginner or an experienced Python developer, this tutorial will provide you with valuable insights into how modules can help you write more efficient and maintainable code.
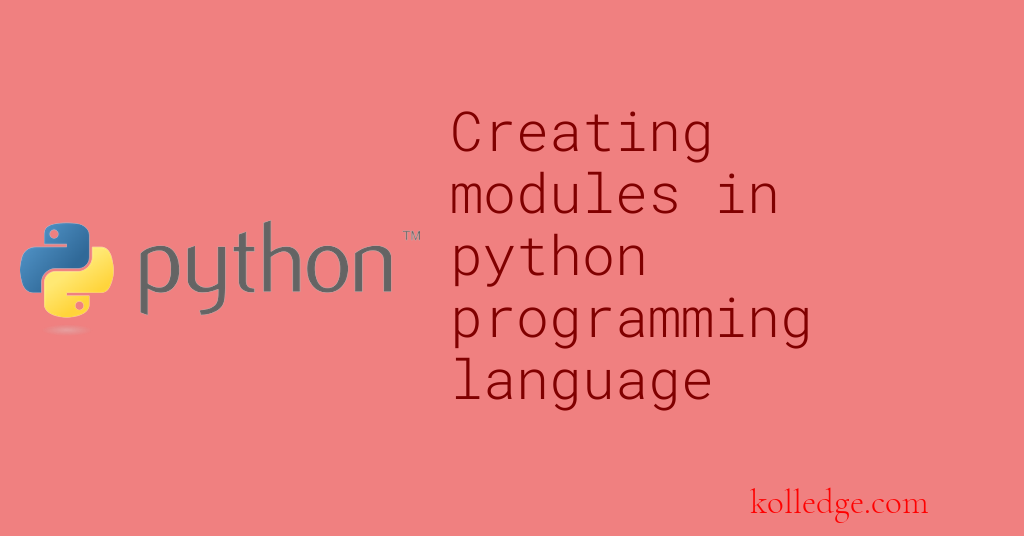
Table of Contents :
- How to create modules in Python
- Naming a Python Module
- Including functions in Module
- Including classes in Module
- Including Variables in Module
- Code sample of creating a module
- Using the module
How to create modules in Python
- A module in Python is simply a file containing Python code.
- We can create a module by
- creating a new file with a
.py
extension and - putting our code in it.
- creating a new file with a
- A module can have code containing function definitions, class definitions, and variables.
- Modules in Python are typically created in a text editor or any python IDE, and saved with a
.py
extension.
Naming a Python Module
- We can give any name to our module.
- We cannot use reserved keywords in module names.
- In fact the name of the module should comply with the naming conventions of python.
Including functions in Module
- Our module can contain function definitions.
- Example :
# mymodule.py
def greet(name):
print("Hello, " + name)
Including classes in Module :
- Our module can contain class definitions.
- Example :
# mymodule.py
class MyClass:
# Class Body
Including Variables in Module :
- Our module can also contain variables of various types (arrays, dictionaries, objects, etc.)
- Example :
# mymodule.py
person = {
"name": "John",
"age": 36,
"country": "Norway"
}
Code sample of creating a module
- Now we'll have a look at a working example of creating a module in python.
- For example, let's say we want to create a module that contains a function for generating Fibonacci numbers.
- We would start by creating a file called
fib.py
- entering the code given below in the file.
- This code defines a function called
fibonacci
, which takes an argument n and returns the nth Fibonacci number.
- We would start by creating a file called
- Code Sample :
# fib.py
def fibonacci(n):
if n <= 1:
return 1
else:
return fib(n-1) + fib(n-2)
Using the module
- We can use the module that we have created in our code by using the
import
statement. - There are different forms of using the import statement.
- For this example we need to import a function from the module.
- To use the module that we have just created we can write the following code :
from fib import fibonacci
res = fibonacci(8)
print("The result of fibonacci = ", res)
# Output
# The result of fib = 34