Beginner Level
Intermediate Level
Advanced Level
Introduction
With threading, we can execute multiple tasks at the same time, speeding up our program's performance. However, when we have multiple threads executing, we need a way to synchronize their activities and make them work together seamlessly. That's where threading events come in. Threading events allow you to coordinate thread activities by signaling when certain conditions have been met. In this tutorial, we'll explore threading events in Python and learn how to use them to make our multithreaded programs more efficient and effective.
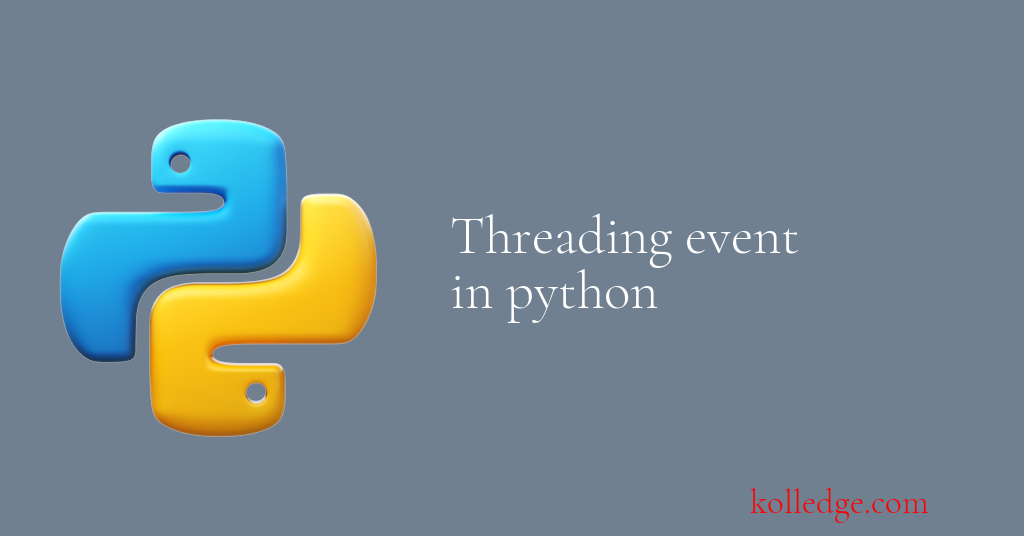
Table of Contents :
- Python Threading Event Object
- Creating Python Threading Event
- Clearing a Threading Event
- Waiting for a Threading Event with Timeout
Python Threading Event Object :
- A threading Event is a synchronization object that allows one thread to signal an event while other threads wait for that event to occur.
- An Event is typically used to signal a thread to stop running, or to inform a thread when a particular task has completed.
Creating Python Threading Event :
- First, you need to import the threading module and create an instance of the Event object.
- Once you have the Event object, you can start one or more threads and have them wait for the event to occur.
- You can then signal the event from another thread when the appropriate condition has been met.
- Code Sample :
import threading
# Create the event object
event = threading.Event()
# Define the worker function
def worker():
print("Worker waiting for event")
event.wait()
print("Worker continuing to run")
# Start the worker thread
thread = threading.Thread(target=worker)
thread.start()
# Wait for a user input
input("Press any key to trigger the event\n")
# Set the event
event.set()
Explanation :
- The main thread creates an instance of the threading Event object using the
threading.Event()
constructor. - The worker function is defined to print a message that it is waiting for the event, then waits for the event using the event.wait() method.
- A new thread is created using the
threading.Thread()
constructor with the worker function as a target, and started withthread.start()
. - The main thread waits for user input using the
input()
function. - When the user enters any key, the event is set using the
event.set()
method. - The worker thread is unblocked and proceeds to print a message indicating it continues to run.
Clearing a Threading Event :
- After an event has been set using the
set()
method, it remains set until explicitly cleared using theclear()
method. - Example:
# Clear the event
event.clear()
Waiting for a Threading Event with Timeout :
- The
wait()
method can be used with an optional timeout parameter to limit the amount of time a thread waits for an event to occur. - The method will return
True
if the event was set within the timeout andFalse
otherwise. - Code Sample :
# Wait for the event to be set, but only for 5 seconds
event.wait(5)
Prev. Tutorial : Multithreaded program
Next Tutorial : Stopping a thread