Beginner Level
Intermediate Level
Advanced Level
Introduction
Integers are one of the fundamental data types in Python and represent whole numbers. In Python, integers are considered to be objects that have certain attributes and methods that can be used to manipulate them. This tutorial will cover the basics of integers in Python, including how to declare, assign, and perform arithmetic operations on them. Whether you are a beginner or experienced programmer, understanding integers is essential to working with Python effectively. So, let's dive in and explore the world of integers in Python!
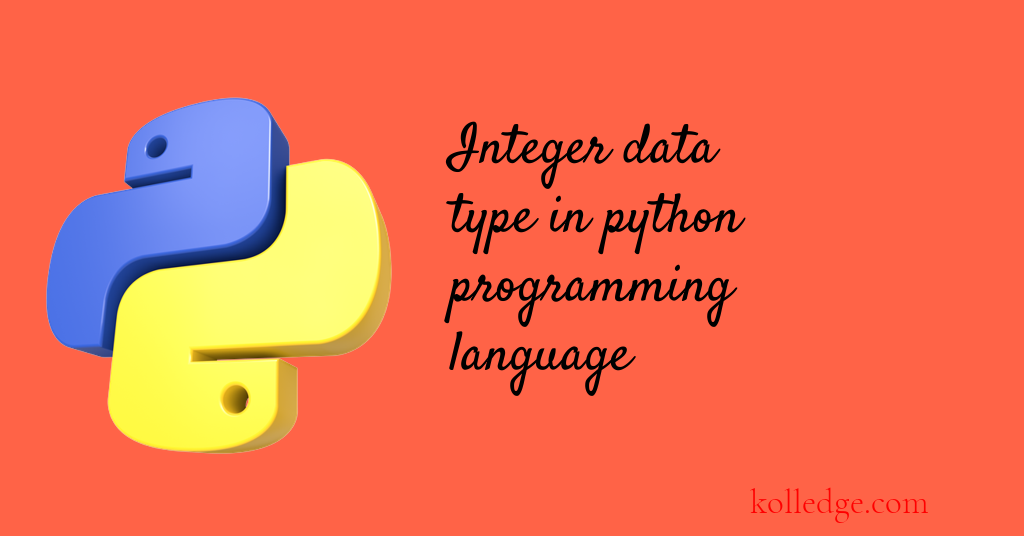
Table of Contents :
- Integer data type
- Creating integer variable
- Creating instance of int class
- Octal, Hexadecimal and binary numbers in Python
Integer data type
- In Python the integer data type represents integer values i.e whole numbers like :
1, 4, 0, 100, 200, -4, -6, -350, 1200
etc. - A simple use case of using the integer data type is saving number of employees of a company in an integer variable.
- The Integer data type is implemented using an
int
class in Python. - In Python all integers are objects of this
int
class. - Integer data type can store positive and negative integer numbers..
- An integer variable can be created in two ways in Python
- Assigning an integer value to the variable
- Creating instance of int() class.
- Integer values other than base 10 can also be stored e.g.
- Binary (base 2)
- Octal (base 8)
- Hexadecimal numbers (base 16) etc.
Creating integer variable :
- An integer variable can be created by simply assigning an integer to a variable.
- Code Sample :
x = 10
print("x is an integer")
print(f"Value of x = {x}")
print(f"Type of x is {type(x)}")
# Output
# x is an integer
# Value of x = 10
# Type of x is <class 'int'>
Creating instance of int class
- we can create an integer variable by creating an instance of
int
class. - Code Sample :
x = int(10)
print("x is an integer")
print(f"Value of x = {x}")
print(f"Type of x is {type(x)}")
# Output
# x is an integer
# Value of x = 10
# Type of x is <class 'int'>
Octal, Hexadecimal and binary numbers in Python :
- Octal numbers are base 8 integers.
- In Python octal numbers are represented by adding a preceding 0 (zero).
- Hexadecimal numbers are base 16 integers.
- In Python hexadecimal numbers are represented by adding a preceding 0x.
- Binary numbers are base 2 integers.
- In Python binary numbers are represented by adding a preceding 0b.
- Code Sample :
x = 0O15
print("x is an Octal number.")
print(f"Value of x = {x}")
print(f"Type of x is {type(x)}")
print()
y = 0x17
print("y is an Hexadecimal number.")
print(f"Value of y = {y}")
print(f"Type of y is {type(y)}")
print()
z = 0b101101
print("z is a binary number.")
print(f"Value of z = {z}")
print(f"Type of z is {type(z)}")
print()
# Output
# x is an Octal number.
# Value of x = 13
# Type of x is <class 'int'>
# y is an Hexadecimal number.
# Value of y = 23
# Type of y is <class 'int'>
# z is a binary number.
# Value of z = 45
# Type of z is <class 'int'>
Prev. Tutorial : Data types in Python
Next Tutorial : Float Data Type