Introduction
In Python, everything is an object. Classes are also objects in Python and are treated as first-class citizens in the language. Python provides an elegant mechanism for modifying classes or functions using decorators. Class Decorators are an advanced feature in Python that can be used to dynamically alter the behavior of a class at runtime. They can be invoked before the class is even defined, or for instance, after the class initialization. In this tutorial, we will explore the concept of Class Decorators in Python, their syntax, and the various use cases where they can come in handy. So, let's dive in!
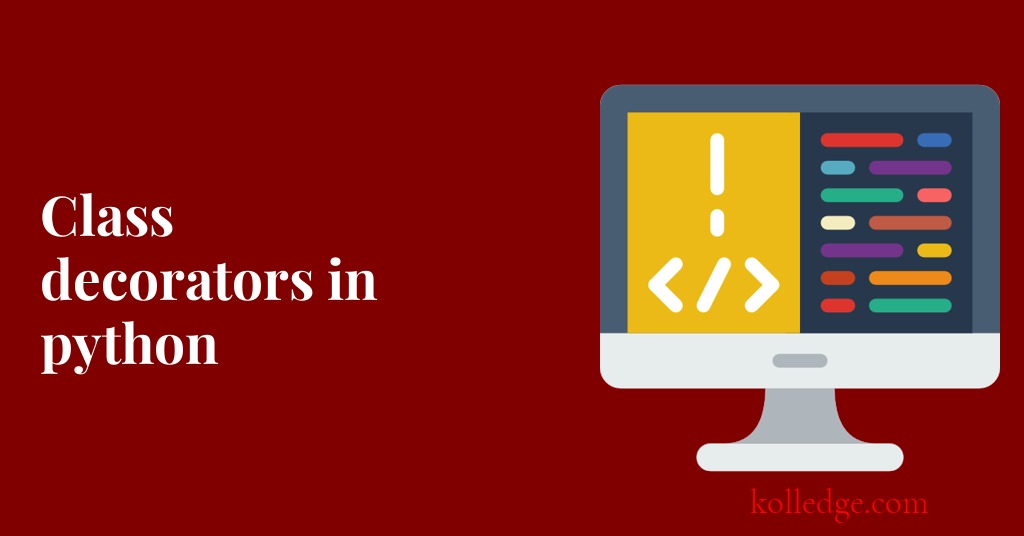
Table of Contents :
- Class Decorators
- Creating class decorators
- Using class decorators
Class Decorators :
Creating class decorators
- Just like functions, you can also use decorators to modify the behavior of a Python class.
- To create a class decorator, you need to define a function that takes a class as an argument and returns a modified class.
- Code Sample :
def uppercase_class(cls):
# Get all the class attributes
attributes = cls.__dict__.copy()
# Modify the attributes that are not under (special) methods
for name, value in attributes.items():
if not name.startswith("__") and callable(value):
def uppercaser(*args, kwargs):
result = value(*args, kwargs)
if isinstance(result, str):
return result.upper()
else:
return result
setattr(cls, name, uppercaser)
return cls
Explanation :
- In this example, the
uppercase_class
function is a decorator that takes a class as an argument - It modifies the class's methods to return their result in uppercase.
- To do this, the decorator creates a modified version of each method that calls the original method and returns its result in uppercase if it's a string.
Using class decorators
- Let's take a look how we can use the class decorator that we created in previous section.
- Code Sample :
@uppercase_class
class Greeting:
def hello(self, name):
return f"Hello, {name}!"
greet = Greeting()
print(greet.hello("Alice"))
print(greet.hello("Bob"))
Output:
HELLO, ALICE!
HELLO, BOB!
Explanation :
- In this example, we use the
uppercase_class
to create a decorator that modifies theGreeting
class by returning the greetings in uppercase. - We create an instance of the
Greeting
class and call thehello
method with the name parameter. - The output shows the modified version of the
hello
method's return value, which is in uppercase.
Prev. Tutorial : Decorators with arguments
Next Tutorial : Property decorators