Beginner Level
Intermediate Level
Advanced Level
Introduction
In Python, a dictionary is a data structure that's used to store key-value pairs. Dictionaries are incredibly helpful, as they enable you to easily store and retrieve data. In this tutorial, you'll learn how to access the values of a dictionary in Python. Whether you're new to Python or have some experience, this tutorial will provide you with a fundamental knowledge of how to manipulate values in a dictionary. So, let's dive in and learn how to access the values of a dictionary using Python!
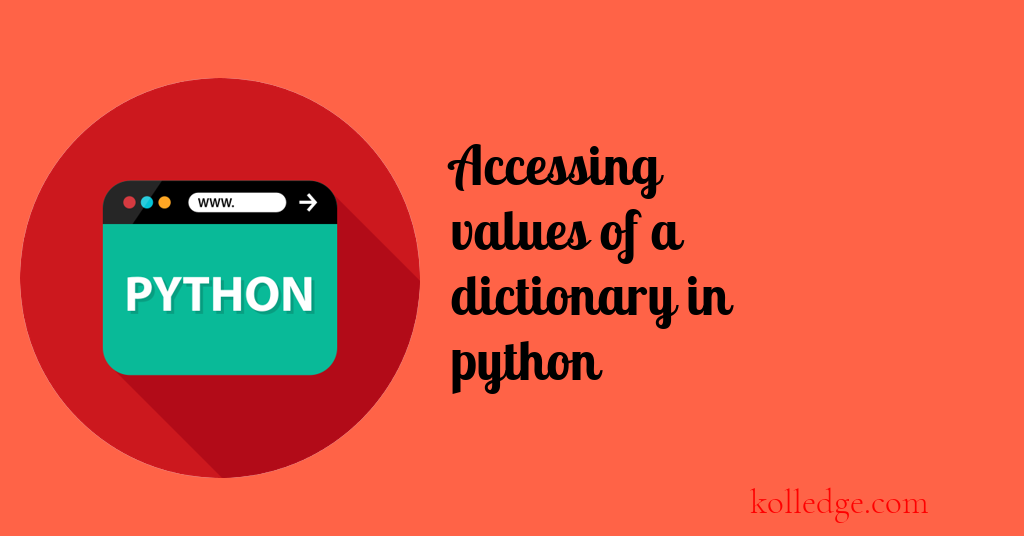
Table of Contents :
- Accessing values of a dictionary
- Accessing values Using square bracket notation
- Accessing values using the get() method
Accessing values of a dictionary :
- We can access the values of a dictionary by using :
- square brackets
- get() method.
Accessing values Using square bracket notation :
- The basic syntax of accessing values from dictionary using square brackets is :
value = d[key]
- If we try to access a key that does not exist, then python interpreter will raise an error.
- Code Sample :
quantities = {
"apple": 10,
"oranges": 20,
"banana": 25,
"Mangoes": 35
}
print("Printing Quantities of fruits :")
print("Quantity of apples = ", quantities["apple"])
print("Quantity of oranges = ", quantities["oranges"])
print("Quantity of banana = ", quantities["banana"])
print("Quantity of Mangoes = ", quantities["Mangoes"])
# Output
# Printing Quantities of fruits :
# Quantity of apples = 10
# Quantity of oranges = 20
# Quantity of banana = 25
# Quantity of Mangoes = 35
Accessing values using the get() method :
- The basic syntax of accessing values from dictionary using
get()
method is :value = d.get(key)
- If we try to access a key that does not exist, then
get()
method will returnNone
. - We can also pass a second argument to the
get()
method which will be used as the default value. - In other words this second parameter will be returned if the key does not exist in the dictionary.
- Code Sample :
quantities = {
"apple": 10,
"oranges": 20,
"banana": 25,
"Mangoes": 35
}
print("Printing Quantities of fruits :")
print("Quantity of apples = ", quantities.get("apple"))
print("Quantity of oranges = ", quantities.get("oranges"))
print("Quantity of banana = ", quantities.get("banana"))
print("Quantity of Mangoes = ", quantities.get("Mangoes"))
print("Quantity of Guavas = ", quantities.get("Guavas"))
print("Quantity of Guavas = ", quantities.get("Guavas", "Zero"))
# Output
# Printing Quantities of fruits :
# Quantity of apples = 10
# Quantity of oranges = 20
# Quantity of banana = 25
# Quantity of Mangoes = 35
# Quantity of Guavas = None
# Quantity of Guavas = Zero
Prev. Tutorial : Dictionaries
Next Tutorial : Adding entry to a dictionary